Question
***READ INSTRUCTIONS BELOW CAREFULLY PLEASE. C++ ONLY*** Trying to build a pig dice game but my code is breaking and I need help finishing. Here
***READ INSTRUCTIONS BELOW CAREFULLY PLEASE. C++ ONLY***
Trying to build a pig dice game but my code is breaking and I need help finishing. Here are the error codes:
The game will first ask the user to pick between game A or game B. Then will proceed to have players 1 & 2 (HUMAN CONTROLLED, NO CP's) roll (for example, player one will have the option of rolling until they hit a 1 or snake eyes. If they choose to hold, it will go to player 2), while keeping track of the score.
*For game A (one dice game), if player 1 or 2 rolls a 1, their score goes back to 0.
*For game B (two dice game), if player 1 or 2 rolls snake eyes (two 1's) OR rolls a 1, their score goes back to 0.
Here is my code:
GameRules.h:
#pragma once
#include "Player.h"
#include "Dice.h"
using namespace std;
class GameRules
{
//define protected variables
protected:
//define two players
//define player 1
Player p1;
//define player 2
Player p2;
//define public variables
public:
//constructor of game rules
//passing two player in parameter
GameRules::GameRules(Player, Player);
//setter for player one
void setPlayer1(Player);
//setter for player two
void setPlayer2(Player);
//create virtual function play
virtual void play() = 0;
};
GameRules.cpp:
#include "GameRules.h"
//set both player to a and b
GameRules::GameRules(Player a, Player b)
{
p1 = a;
p2 = b;
}
void GameRules::setPlayer1(Player a)
{
//setting p1 to a
//calling overloaded = operator here
p1 = a;
}
void GameRules::setPlayer2(Player b)
{
//setting p2 to b
//calling overloaded = operator here
p2 = b;
}
GameModeA.h:
#pragma once
#include "GameRules.h"
//define game mode a that extends game rules
class GameModeA : public GameRules
{
//adding dice to the private member
private:
//adding dice d1
Dice d1;
//define public methods to the class dice
public:
//adding gamemode a constructor
//calling parent constructor as well by doing : gameRules(a,b)
GameModeA(Player a, Player b) : GameRules(a, b) {}
//define virtual function play definition here
void play();
};
GameModeA.cpp:
#include
#include "GameRules.h"
#include "GameModeA.h"
using namespace std;
void GameModeA::play()
{
//set player one turn to true
p1.Turn(true);
int n;
char playerChoice;
//loop until it's player one turn
}
GameModeB.h:
#pragma once
#include "GameRules.h"
// game mode b extends gameRules
//this is second child class
class GameModeB : public GameRules
{
private:
//having two dice in game mode B
Dice d1;
Dice d2;
public:
//constructor player a and b : calling parent class constructor also
GameModeB(Player a, Player b) : GameRules(a, b) {}
//declare gamemodeB play definition
void play();
};
GameModeB.cpp:
#include
#include "GameRules.h"
#include "GameModeB.h"
using namespace std;
void GameModeB::play()
{
//set player one turn to true
p1.Turn(true);
int n, n1;
char playerChoice;
//while player one turn
}
Player.h:
#pragma once
#include
#include "GameRules.h"
#include "Dice.h"
//declare player class
class Player
{
private:
//define name to store player name
std::string name;
//turn will decide whose turn is it
bool turn;
//store score of player
int score;
public:
//constructor of player using name field
Player(std::string name);
//default constructor of class player
Player();
//copy constructor of player
//it will set current player to the one what is passing in parameter
Player(const Player &p);
//overriding = operator to assign player value to calling object
//passing player as const so that cannot change value of p
Player& operator=(const Player &p);
//setter for name
void setName(std::string n);
//setter for score
void Score(int sc);
//getter for store. const so that cannot change value of score
int Score()const;
//set turn
void Turn(bool t);
//get the turn. const so that cannot change turn
bool Turn()const;
//getter of the name
std::string Name()const;
};
Player.cpp:
#include
#include "Player.h"
Player::Player(std::string name)
{
//set class name to parameter name
this->name = name;
//set turn to false initially
turn = false;
//set score to 0
score = 0;
}
//default constructor of class player
Player::Player()
{
//set name to x
name = "X";
//set turn to false
turn = false;
//set score to 0
score = 0;
}
//copy constructor of player
//it will set current player to the one what is passing in parameter
Player::Player(const Player &p)
{
//set this class pointer to the address of player p
*this = p;
}
//overriding = operator to assign player value to calling object
//passing player as const so that cannot change value of p
Player& Player::operator=(const Player &p)
{
//set name to p name
name = p.name;
//set turn to p turn
turn = p.turn;
//set score to p score
score = p.score;
//return calling object reference
return *this;
}
//setter for name
void Player::setName(std::string n)
{
//set name to n
name = n;
}
//setter for score
void Player::Score(int sc)
{
//set score to sc
score = sc;
}
//getter for store. const so that cannot change value of score
int Player::Score()const
{
//RETURN SCORE
return score;
}
//set turn
void Player::Turn(bool t)
{
//set turn to t
turn = t;
}
//get the turn. const so that cannot change turn
bool Player::Turn()const
{
//return turn
return turn;
}
//getter of the name
std::string Player::Name()const
{
return name;
}
Dice.h:
#pragma once
#include "Player.h"
#include "GameRules.h"
//declare class dice
class Dice
{
public:
//declare function roll for class dice
int roll();
};
Dice.cpp:
#include
#include "Dice.h"
#include "GameRules.h"
int Dice::roll()
{
//declare integer to store random number
int randInteger;
//setting random time to stop execution
srand(static_cast
//store value to rand integer
//range will be 1 to 6
randInteger = (int)(1 + rand() % (6 - 1 + 1));
return randInteger;
}
main.cpp:
#include
#include "GameRules.h"
#include "GameModeA.h"
#include "GameModeB.h"
#include "Player.h"
#include "Dice.h"
using namespace std;
int main()
{
char gameChoice;
cout
cin >> gameChoice;
gameChoice = (toupper(gameChoice));
if (gameChoice == 'E')
{
cout
system("pause");
return 0;
}
Player p1, p2;
string s1;
cout
cin >> s1;
p1.setName(s1);
cout
cin >> s1;
p2.setName(s1);
if (gameChoice == 'A')
{
GameModeA g(p1, p2);
g.play();
}
p1.Turn(true);
int n;
char playerChoice;
while (p1.Turn())
{
//print score of player one
cout
//print player name and instructions
cout
//get playerChoice
cin >> playerChoice;
//change choice to upper case
playerChoice = (toupper(playerChoice));
//check if choice is H
if (playerChoice == 'H')
{
//set player one turn to false
p1.Turn(false);
//set player two turn to true
p2.Turn(true);
//output player one name and score
cout
break;
}
//roll the dice and get random number n
Dice d1;
n = d1.roll();
//if n is 1
if (n == 1)
{
//set player one turn to false
p1.Turn(false);
//set player two turn to true
p2.Turn(true);
//set player one score to 0
p1.Score(0);
//print player one name and lose message
cout
//print score
cout
break;
}
//increase player one score by n
p1.Score(p1.Score() + n);
}
//loop until player two turn
while (p2.Turn())
{
//print score
//everything is same as mentioned above
cout
cout
cin >> playerChoice;
playerChoice = (toupper(playerChoice));
if (playerChoice == 'H')
{
p2.Turn(false);
p1.Turn(true);
cout
break;
}
Dice d1;
n = d1.roll();
if (n == 1)
{
p2.Turn(false);
p1.Turn(true);
cout
cout
break;
}
p2.Score(p2.Score() + n);
}
//if player one score is more than player two
if (p1.Score() > p2.Score())
{
//print player one win
cout
}
//if player one score is less than player two
else if (p1.Score()
{
//print player two win
cout
}
else
{
//else print draw
cout
}
if (gameChoice == 'B')
{
GameModeB g(p1, p2);
g.play();
}
p1.Turn(true);
int n, n1;
char playerChoice;
while (p1.Turn())
{
//print score
cout
//print turn notification
cout
//get player choice
cin >> playerChoice;
//convert choice to upper case
playerChoice = (toupper(playerChoice));
//if choice is H
if (playerChoice == 'H')
{
//set player one turn to false
p1.Turn(false);
//set player two turn to true
p2.Turn(true);
cout
break;
}
Dice d1, d2;
//roll first dice
n = d1.roll();
//roll second dice
n1 = d2.roll();
//if both are showing 1
if (n == 1 && n1 == 1)
{
//player one turn to false
p1.Turn(false);
//player two turn to true
p2.Turn(true);
//player one score to 0
p1.Score(0);
cout
cout
break;
}
//increase player one score by n and n1
p1.Score(p1.Score() + n + n1);
}
//while player two turn
//logic is same as above
while (p2.Turn())
{
cout
cout
cin >> playerChoice;
playerChoice = (toupper(playerChoice));
if (playerChoice == 'H')
{
p2.Turn(false);
p1.Turn(true);
cout
break;
}
Dice d1, d2;
n = d1.roll();
n1 = d2.roll();
if (n == 1 && n1 == 1)
{
p2.Turn(false);
p1.Turn(true);
p2.Score(0);
cout
cout
break;
}
p2.Score(p2.Score() + n + n1);
}
if (p1.Score() > p2.Score())
{
cout
}
else if (p1.Score()
{
cout
}
else
{
cout
}
if (playerChoice == 'E')
{
cout
}
}
816 Errors A oWarningsM 0 MessagesBuild + IntelliSense Entire Solution Search Error List Project PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice PigDice File main.cpp main.cpp gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h gamerules.h Code Description Line 3) C2086 int n:redefinition C2086 'char playerChoice: redefinition C3646 p: unknown override specifier ? (4430 missing type specifier-int assumed. Note: C++ does not support default-int 'p2: unknown override specifier missing type specifier-int assumed. Note: C++does not support default-int syntax error: identifier Player. 23 23 C3646 C4430 ?C2061 C2061 syntax error: identifier Player C2061 syntax error: identifier Player C3646 'p1: unknown override specifier C4430 missing type specifier- int assumed. Note: C++ does not support default-int C3646 p2: unknown override specifier ( 23 4430 missing type specifier-int assumed. Note: C++ does not support default-int C2061 syntax error: identifier Player C2061 syntax error: identifier "Player C2061 syntax error: identifier PlayerStep by Step Solution
There are 3 Steps involved in it
Step: 1
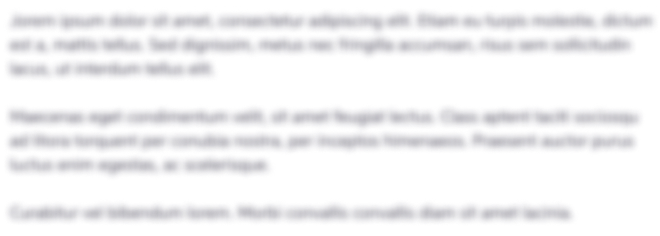
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started