Question
Reads an unspecified number of sorted values from a file specified by the user, and displays the median value (The median is the middle number
Reads an unspecified number of sorted values from a file specified by the user, and displays the median value (The median is the middle number when the numbers are sorted by size.), average value, and how many numbers were read. (Note: Do not use an array.)
Using the pseudocode developed in the last exercise, write the program in these two steps:
Step 1: Write a shell program that implements stub functions demonstrating that your function calls work. (Simple cout statements in functions.)
Step 2: Fill in the code and complete the program
Documented source code with shell and stub functions for Step 1.
Documented source code for completed program in Step 2.
Code down below is the code from previous to build on.
/ Reads an unspecified number of sorted values from a file specified by the user, // and displays the median value (The median is the middle number when the numbers // are sorted by size.), average value, and how many numbers were read. // (Note: Do not use an array.) #include using namespace std; // Prompt user for file name and return ifstream // Pre: inData declared // Post: inData contains valid ifstream for data file void GetFileInfo(ifstream& inData); // Reads and counts values in a file and calculates the average // Pre: inData contains valid ifstream to a file of sorted float values // Post: numCt contains number of values in file // avg contains average of the values void GetInitialValues(ifstream &inData, int &numCt, float &avg); // Finds and returns the median of float values in a file // Pre: inData links to a file of sorted float values and // numCt contains the number of values // Post: Return median of values read from file float GetMedian(ifstream &inData, int numCt); // Prints calculated results // Pre: median contains calculated median // avg contains calculated average // numCt contains number of values read // Post: Formatted values printed to screen void DisplayResults(float median, float avg, int numCt); int main(){ ifstream inData; // Link to input file int numCt; // Number of values in file float avg; // Average of numbers read float median; // Holds median value from file // Call function to get/open file GetFileInfo(inData); // Call function to calculate initial values (numCt, sum, avg) GetInitialValues(inData, numCt, avg); // Call function to find median value median = GetMedian(inData, numCt); // Display results DisplayResults(median, avg, numCt); // Close file inData.close(); return 0; } // Prompt user for file name and return ifstream // Pre: inData declared // Post: inData contains valid ifstream for data file void GetFileInfo(ifstream& inData){ // Prompt user for file name and open file // Loop until valid file name provided } // Reads and counts values in a file and calculates the average // Pre: inData contains valid ifstream to a file of sorted float values // Post: numCt contains number of values in file // avg contains average of the values void GetInitialValues(ifstream &inData, int &numCt, float &avg){ float sum = 0; // Sum of all values in file initialized to 0 float num; numCt = 0; // Priming read of file // Loop until end of file // Update sum // Update numCt // Read next number // Calculate average } // Finds and returns the median of float values in a file. The median is the // middle number when the numbers are sorted by size. If there are an even number // of values, the median is the average of the middle two. // Pre: inData links to a file of sorted float values and // numCt contains the number of values // Post: Return median of values read from file float GetMedian(ifstream &inData, int numCt){ float num1, num2; // Values read from file int numsToRead; // Number of values to read from file float median; // Median value from file // If numCt is odd numsToRead = // Middle of list of numbers median = GetNumber(inData, numsToRead); // See below for documentation // else // Get middle two numbers (num1 & num2) median = (num1 + num2) / 2; return median; } // Prints calculated results // Pre: median contains calculated median // avg contains calculated average // numCt contains number of values read // Post: Formatted values printed to screen void DisplayResults(float median, float avg, int numCt){ // Output median, avg , and numCt } // NOTE: This function still needs a prototype at the top // Returns the specified number from a file // Pre: inData contains link to a valid file containing float values // numsToRead contains the index of the value to return from the file // Post: Returns the numsToRead(th) number in the file float GetNumber(ifstream &inData, int numsToRead){ // Read numsToRead values from the file // Return last number read from file }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
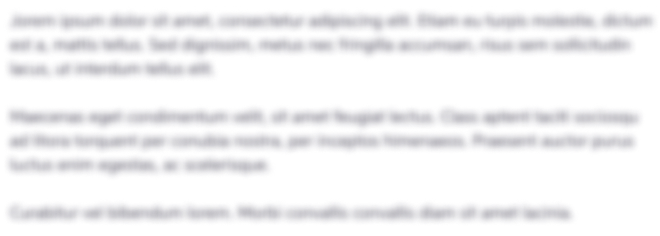
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started