Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Really need help modifying this program to work. Will give great rating to Chegg. C++ //Fraction.h - DO NOT CHANGE #ifndef _FRACTION_H #define _FRACTION_H #include
Really need help modifying this program to work. Will give great rating to Chegg. C++
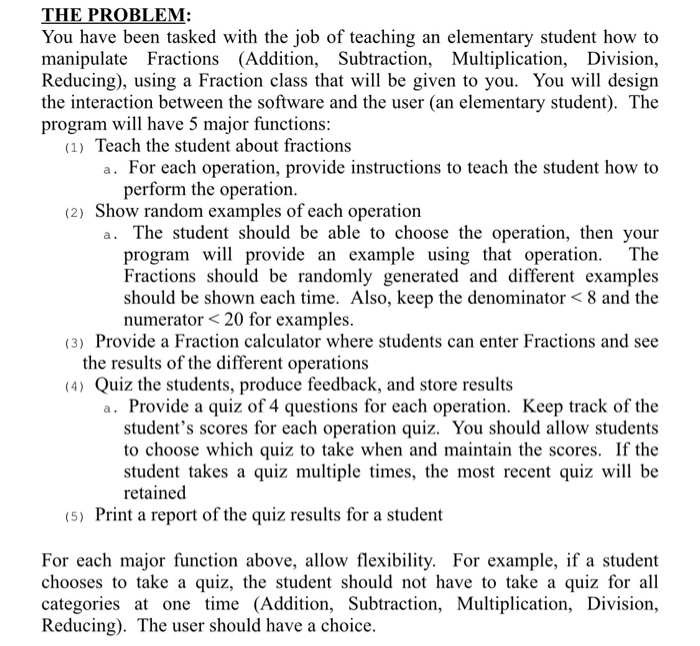
//Fraction.h - DO NOT CHANGE
#ifndef _FRACTION_H
#define _FRACTION_H
#include
using namespace std;
//Helper function
int gcd(int a, int b);
class Fraction{
//Data Members
int num;
int den;
public:
//Constructors
Fraction();
Fraction(int n);
Fraction(int n, int d);
//Extra methods
void reduce();
void PrintNotReduced(ostream& os)const;
//Getters and Setters
int getNum()const;
int getDen()const;
void setNum(int n);
void setDen(int d);
//Arithmetic Operators
friend Fraction operator+(const Fraction& left, const Fraction& right);
friend Fraction operator-(const Fraction& left, const Fraction& right);
Fraction operator*(const Fraction& right)const; //left operand is the calling object
friend Fraction operator/(const Fraction& left, const Fraction& right);
//Relational Operators
friend bool operator==(const Fraction& left, const Fraction& right);
friend bool operator!=(const Fraction& left, const Fraction& right);
friend bool operator
friend bool operator>(const Fraction& left, const Fraction& right);
friend bool operator
friend bool operator>=(const Fraction& left, const Fraction& right);
//Insertion/Extraction Operators
friend ostream& operator
friend istream& operator>>(istream& is, Fraction& right);
//Increment/Decrement Operators
Fraction operator++(int); // postfix
Fraction operator++(); //prefix
friend Fraction operator--(Fraction& op, int); //postfix
friend Fraction operator--(Fraction& op); // prefix
};
#endif
//Fraction.cpp - DO NOT CHANGE
#include "Fraction.h"
//***************************************************************
//class definition/ implementation
//***************************************************************
//***************************************************************
//Constructors
//***************************************************************
Fraction::Fraction():num(0), den(1){}
Fraction::Fraction(int n):num(n), den(1){}
Fraction::Fraction(int n, int d):num(n){
if (d == 0)
d = 1;
den = d;
}
//***************************************************************
//Helper function
//***************************************************************
int gcd(int a, int b){
if (b == 0)
return a;
return gcd (b, a % b);
}
//***************************************************************
//Extra methods
//***************************************************************
void Fraction::reduce(){
int val = gcd(num, den);
num/= val;
den/= val;
}
void Fraction::PrintNotReduced(ostream& os)const{
os
}
//***************************************************************
//Getters and Setters
//***************************************************************
int Fraction::getNum()const{
return num;
}
int Fraction::getDen()const{
return den;
}
void Fraction::setNum(int n){
num = n;
}
void Fraction::setDen(int d){
if (d == 0)
d = 1;
den = d;
}
//***************************************************************
//Arithmetic Operators
//***************************************************************
Fraction operator+(const Fraction& left, const Fraction& right){
Fraction temp;
temp.num = left.num * right.den + left.den * right.num;
temp.den = left.den * right.den;
return temp;
}
Fraction operator-(const Fraction& left, const Fraction& right){
Fraction temp;
temp.num = left.num * right.den - left.den * right.num;
temp.den = left.den * right.den;
return temp;
}
Fraction Fraction::operator*(const Fraction& right)const{
Fraction result;
result.num = num * right.num;
result.den = den * right.den;
return result;
}
Fraction operator/(const Fraction& left, const Fraction& right){
Fraction res;
res.num = left.num * right.den;
res.den = left.den * right.num;
return res;
}
//***************************************************************
//Relational Operators
//***************************************************************
bool operator==(const Fraction& left, const Fraction& right){
bool equal = false;
if (left.num* right.den == right.num * left.den)
equal = true;
return equal;
}
bool operator!=(const Fraction& left, const Fraction& right){
return !(left == right);
}
bool operator
bool lessThan = false;
if (left.num* right.den
lessThan = true;
return lessThan;
}
bool operator>(const Fraction& left, const Fraction& right){
return !( left
}
bool operator
bool lessThanEqual = false;
if (!(left.num* right.den > right.num * left.den))
lessThanEqual = true;
return lessThanEqual;
}
bool operator>=(const Fraction& left, const Fraction& right){
bool greaterThanEqual = false;
if (!(left.num* right.den
greaterThanEqual = true;
return greaterThanEqual;
}
//***************************************************************
//Insertion/Extraction Operators
//***************************************************************
ostream& operator
THE PROBLEM: You have been tasked with the job of teaching an elementary student how to manipulate Fractions (Addition, Subtraction, Multiplication, Division, Reducing), using a Fraction class that will be given to you. You will design the interaction between the software and the user (an elementary student). The program will have 5 major functions (1) Teach the student about fractions a. For each operation, provide instructions to teach the student how to perform the operation (2) Show random examples of each operation a. The student should be able to choose the operation, then your program will provide an example using that operation. The Fractions should be randomly generated and different examples should be shown each time. Also, keep the denominator 8 and the numerator 20 for examples (3) Provide a Fraction calculator where students can enter Fractions and see the results of the different operations (4) Quiz the students, produce feedback, and store results a. Provide a quiz of 4 questions for each operation. Keep track of the student's scores for each operation quiz. You should allow students to choose which quiz to take when and maintain the scores. If the student takes a quiz multiple times, the most recent quiz will be retained (5) Print a report of the quiz results for a student For each major function above, allow flexibility. For example, if a student chooses to take a quiz, the student should not have to take a quiz for all categories at one time (Addition, Subtraction, Multiplication, Division, Reducing). The user should have a choice Fraction temp = right;
temp.reduce();
os
return os;
}
istream& operator>>(istream& is, Fraction& right){
char dummy;
is >> right.num >> dummy >> right.den;
return is;
}
//***************************************************************
//Increment/Decrement Operators
//***************************************************************
Fraction Fraction::operator++(int){ // postfix
Fraction temp = *this; /eed a name for the calling object inside the method
num += den;
return temp;
}
Fraction Fraction::operator++(){
num += den;
return *this;
}
Fraction operator--(Fraction& op, int){ //postfix
Fraction temp = op;
op.num -= op.den;
return temp;
}
Fraction operator--(Fraction& op){ // prefix
op.num -= op.den;
return op;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
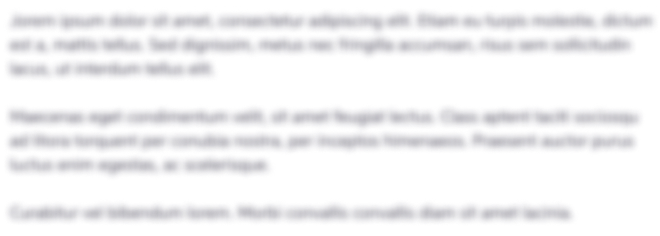
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started