Question
Receiving errors that size and theData cannot be resolved to a variable and receiving errors. Not sure what the problem is but here is the
Receiving errors that size and theData cannot be resolved to a variable and receiving errors. Not sure what the problem is but here is the code and after is the test code.
package lab2; import java.util.Arrays; import java.util.AbstractList;
/** * This class implements some of the methods of the Java * ArrayList class. * * */ public class MyArrayList extends AbstractList { // Data Fields /** The default initial capacity */ private static final int INITIAL_CAPACITY = 10; /** The underlying data array */ private E[] data; /** The current size */ private int pos = 0; /** The current capacity */ private int capacity = 0;
/** * Construct an empty ArrayList with the default * initial capacity */ public MyArrayList() { capacity = INITIAL_CAPACITY; data = (E[]) new Object[capacity]; }
/** * Allocate a new array to hold the directory * */ private void reallocate() { capacity = 2 * capacity; data = Arrays.copyOf(data, capacity); } /** * Add an entry to the end of the list * @param anEntry - The anEntry to be inserted * @return true/false - if the entry is inserted successfully at the end */ public boolean add(E anEntry) { if (pos == capacity) { reallocate(); } data[pos] = anEntry; pos++; return true; } /** * Get a value in the array based on its index. * @param index - The index of the item desired * @return The contents of the array at that index * @throws ArrayIndexOutOfBoundsException - if the index * is negative or if it is greater than or equal to the * current size */ public E get(int index) { if (index = pos) { throw new ArrayIndexOutOfBoundsException(index); } return data[index]; } /** * Set the value in the array based on its index. * @param index - The index of the item desired * @param newValue - The new value to store at this position * @return The old value at this position * @throws ArrayIndexOutOfBoundsException - if the index * is negative or if it is greater than or equal to the * current size * @throws NullPointerException - if newValue is null */ public E set(int index, E newValue) { if (index = pos) { throw new ArrayIndexOutOfBoundsException(index); } if(newValue == null) throw new NullPointerException(); E oldValue = data[index]; data[index] = newValue; return oldValue; } /** * Get the current size of the array * @return The current size of the array */ public int size() { return pos; } /** * Returns the index of the first occurence of the specified element * in this list, or -1 if this list does not contain the element * @param item The object to search for * @returns The index of the first occurence of the specified item * or -1 if this list does not contain the element */ public int indexOf(Object item) { for (int i = 0; i size) { throw new ArrayIndexOutOfBoundsException(index); } if(newValue.equals(null)) { throw new NullPointerException(); } if(size==capacity) { this.reallocate(); }
for(int i=size; i>=index; i--) { theData[i+1]=theData[i];} theData[index]=newValue; size++; } /** * Construct an empty ArrayList with a specified initial capacity * @param capacity - The initial capacity * @throws IllegalArgumentException - if the capacity is less 0 */ public MyArrayList(int capacity) { if(capacity=size) { throw new ArrayIndexOutOfBoundsException(index); } E oldValue = theData[index]; for(int i=index; i { theData[i]=theData[i+1]; } size--; }
/** * Count the total number of elements equals to elem * @param theValue - the compared element * @return the total number of replicas or -1 if not found in the list */ public int countApperance(E theValue) { int total=0; for (int i=0; i {if(theValue.equals(theData[i])) {total++;}} if(total==0) {total=(-1);} return total; } /** * Remove all the duplicated elements equals to theValue * @param theValue - the duplicated element to be removed */ public void removeDuplicate(E theValue) { for (int i=0; i {if(theData[i].equals(theValue)) {this.remove(i);}} } /* * ================================ The end of functions need to be filled =================================================== * */
_____________________________________________________________________________________________________________________________________________________
2+ * This is the Junit Test file for MyArrayList class, 4 package lab2; 6+ import static org.junit. Assert. *;. 5 12 public class TestMyArrayList { private MyArrayListStep by Step Solution
There are 3 Steps involved in it
Step: 1
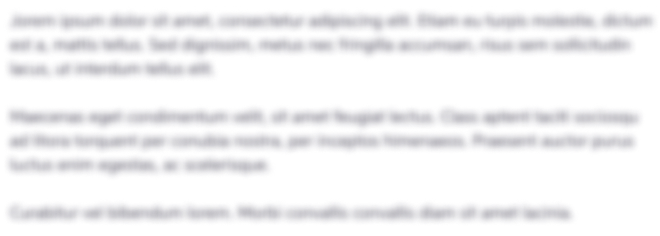
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started