Question
Redo HW13: Student Grades (or do it for the first time : ), but this time change the structure to a class named CGrade .
Redo HW13: Student Grades (or do it for the first time : ), but this time change the structure to a class named CGrade. The class should have member variables for all input data (quiz1, quiz2, midterm, finalExam, percent, name, and grade). Make all member variables private. Include member functions, the same as the ones in HW13(GetInfo, CalcGrade, CalcPercent, and DispGrade). The member functions should all be public. Also, include the 3 special constructor functions. Create a default, type, and copy constructors. The default constructor will assign zeros (0s) to all grades and percent, give the name NULL to the student, and set the grade to an F. This should be displayed first in main to show that everything is zeroed out. The type constructor should take as parameters all of your private member variables with defaults of 0s for all grades, percent, and a grade of an F. However, the first argument should (which is the name of the student) should not have a default value, but one should be provided during the allocation of the object. The last constructor, copy, should copy member by member. Be sure to cout a statement (inside the constructors implementation) when each constructor is called.
Note: If you get a warning in your type constructor saying deprecated conversion from string constant to char* dont worry about it. As the class progresses, we will figure out a way to understand and get rid of this warning.
The program has 3 files total. A header file called cgrade.h, where your class should be declared. An implementation file called cgrade.cpp, where your functions implementations are stored. Lastly, youll need a file to store main called main.cpp. You can use your existing code and change it where you need to. You can save your files in your HW16 directory.
To help you get started, I went ahead and created a HW16 subdirectory for you and put starter kit in there called CGradeStarterKit.tar.gz. This is a compressed archive file, much like a zip file, it contains files that need to be extracted. To extract the files, use this command:
tar xvzf CGradeStarterKit.tar.gz
This will extract the files contained within the archive into the present working directory. You should then find a partially completed main.cpp and cgrade.h files and an executable file. Youll need to fill in the ??? in those files and create the implementation of the functions in a file called cgrade.cpp. To run the example executable is ./GradeCalc
NOTE: You only need to extract the files once! If you extract the cpp file, write some code, and then extract again at a later point in time, the original cpp file in the archive will be extracted and overwrite any code that you've already written!
A sample run is below:
$ ./GradeCalc Default Constructor! Type Constructor! Copy Constructor! The grades of S1 (default constructor): NULL: 0.0%F Info for S1: Enter student's full name: Elon Musk Enter Quiz 1 grade (out of 10): 6 Enter Quiz 2 grade (out of 10): 7 Enter midterm grade (out of 100): 73 Enter final exam grade (out of 100): 88 The grades of the students are: Elon Musk: 78.5% C Edgar Allen Poe: 0.0% F Edgar Allen Poe: 0.0% F
This is cgrade.h
#ifndef CGRADE_HEADER #define CGRADE_HEADER
class CGrade { public: // constructors CGrade(); // default constructor ??? // type constructor ??? // copy constructor
// member functions ???
private: // data members char m_name[256]; double m_quiz1; double m_quiz2; double m_midterm; double m_finalExam; double m_percent; char m_grade; };
#endif // CGRADE_HEADER
This is main.cpp
#include
// ==== main ================================================================== // // ============================================================================
int main() { CGrade S1; // first student CGrade S2(???); // second student ??? // copy of the second student
// Display the default constructor info cout << " The grades of S1 (default constructor): "; ???
// prompt the user for name/grades cout << " Info for S1: "; ???
// call the function to calculate percent/grade ???
// display their grades cout << " The grades of the students are: "; ???
return 0; } // end of "main"
This is HW13
HW13: Student Grades
You are to write a program for a class with the following grading policies:
There are two quizzes, each graded on the basis of 10 points.
There is one midterm exam and one final exam, each graded on the basis of 100 points.
The final exam counts for 50 percent of the grade, the midterm counts for 25 percent, and the two quizzes together count for a total of 25 percent.
Any grade of 90 or more is an A, any grade 80 or more (but less than 90) is a B, any grade of 70 or more (but less than 80) is a C, any grade of 60 or more (but less than 70) is a D, and any grade below 60 is an F.
The program will read in the name and scores of only 2 students and output the students name, percentage, and grade for the class. Define and use a structure for the student record. A sample run is below:
$ ./a.out Info for S1: Enter student's full name: Edgar Allen Poe Enter Quiz 1 grade (out of 10): 9 Enter Quiz 2 grade (out of 10): 10 Enter midterm grade (out of 100): 98 Enter final exam grade (out of 100): 97 Info for S2: Enter student's full name: Elon Musk Enter Quiz 1 grade (out of 10): 6 Enter Quiz 2 grade (out of 10): 7 Enter midterm grade (out of 100): 73 Enter final exam grade (out of 100): 88 The grades of the students are: Edgar Allen Poe: 96.8% A Elon Musk: 78.5% C
Here are the guidelines for how it will be programmed. First and foremost, the structure will be called Grade and it will have 7 variables inside of it: quiz1, quiz2, midterm, finalExam, percent, name, and grade. Quiz1, quiz2, midterm, and final exam will be a double that contains the score the student received. Percent will have the weighted percent for the class. Grade is a char that will hold the overall class grade. And name is a char array that will hold the students first, middle (if any), and last name.
In main, youll create and allocate space for 2 students using the structure. Youll then pass as reference, one at a time, the student info structure to a function called GetInfo which will collect the information needed to fill the majority of the structure (quiz1, quiz2, midterm, finalExam, and name). Then main will call the CalcGrade function and pass by reference, one at a time, the student info structure to calculate the overall grade for each student. Finally, main will call the DispGrade function, passing each structure by value (also one at a time), to display the final grade for each student.
GetInfo will initialize the structure for each student with the grade they received for each quiz, midterm, and final exam as well as their full name. This will have a void return type and a parameter of pass by reference.
CalcGrade will call the function CalcPercent to calculate the percentage of the student. Then CalcGrade will use the update information in the structure to assign the appropriate grade. This will have a void return type and a parameter of pass by reference.
CalcPercent will calculate the weighted percentage for the student. This will have a void return type and a parameter of pass by reference.
DispGrade will display the name, percentage, and grade for each student. Note that the percentage will be displayed with only one decimal value (tenths place). This will have a void return type and a parameter of pass by value.
On Canvas, Ill post up a flowchart for you to better understand the flow of the program. You can save your code in a file named studentGrades.cpp in your HW13 directory.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
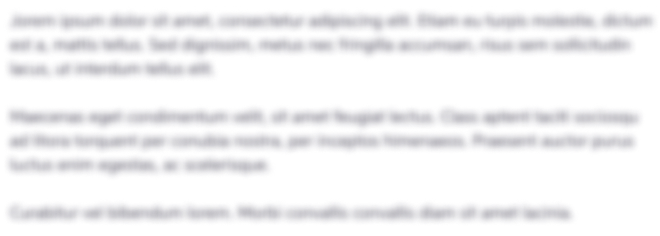
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started