Question
Redo HW13/HW16 (or do it for the first time : ), but this time youll overload 3 operators ( == , >> , and <
Redo HW13/HW16 (or do it for the first time : ), but this time youll overload 3 operators ( ==, >>, and <<). The idea is very similar to the examples we talked about in class using the class CCircNum with the files called OpOver1.cpp and Streams2.cpp. When youre overloading the extractor and insertion operators youll want to use the member functions you already created (i.e. DispGrade and GetInfo). However, youll want to now provide arguments to those two functions.
DispGrade should now have an ostream argument which defaults to cout. In addition, the entire function should be a constant. We talked about this idea in class and you can reference Streams2.cpp for help on that.
GetInfo should now have an istream argument that defaults to cin.
operator>> should have two arguments and return an istream by reference. Refer your notes on specifics for this.
operator<< should also have two arguments and return an ostream by reference.
For both the extraction and insertion operators, which one should have a constant parameter? Once you figure that out, implement it as so.
operator== should have an object passed by reference as a parameter and return a boolean. How should you check each private member, specifically the cstring? I suggest looking into the cstring library for help on that.
Note: If you get a warning in your type constructor saying deprecated conversion from string constant to char* dont worry about it as the class progress we will figure out a way to understand and get rid of this warning.
The program has 3 files total. A header file called cgradeOverload.h, where your class should be declared. An implementation file called cgradeOverload.cpp, where your functions implementations are stored. Lastly, youll need a file to store main called main.cpp. You can use your existing code and change it where you need to. You can save your files in your HW17 directory.
To help you get started, I went ahead and created a HW17 subdirectory for you and put starter kit in there called CGradeOverloadStarterKit.tar.gz. This is a compressed archive file, much like a zip file, it contains files that need to be extracted. To extract the files, use this command:
tar xvzf CGradeOverloadStarterKit.tar.gz
This will extract the files contained within the archive into the present working directory. You should then find a completed main.cpp and a partially completed cgradeOverload.h files and an executable file. Youll need to fill in the ??? in cgradeOverload.h and create the implementation of the functions in a file called cgradeOverload.cpp. You can copy and paste your previous cgrade.cpp file and change the two functions (DispGrade and GetInfo) and add the implementation of the overloaded functions. To run the example executable is ./GradeCalcOverload
NOTE: You only need to extract the files once! If you extract the cpp file, write some code, and then extract again at a later point in time, the original cpp file in the archive will be extracted and overwrite any code that you've already written!
A sample run is below:
$ ./GradeCalcOverload Default Constructor! Type Constructor! Copy Constructor! The grades of S1 (default constructor): NULL: 0.0% F Enter student's full name, Quiz 1 grade (out of 10.0), Quiz 2 grade (out of 10.0), midterm grade (out of 100.0), and final exam grade (out of 100.0). (HIT ENTER AFTER EACH ONE!!!) Elon Musk 6 7 73 88 S1 != S2 S2 == S3 The grades of the students are: Elon Musk: 78.5% C Edgar Allen Poe: 0.0% F Edgar Allen Poe: 0.0% F
This is HW13 Student Grades
studentGrades.cpp
#include
//Structure used for grade struct Grade { char name[80]; double quiz1;
double quiz2;
double midterm; double finalExam; double percent; char grade; };
//function prototypes void GetInfo(Grade &grade); void DispGrade(Grade &grade); void CalcGrade(Grade &grade); void CalcPercent(Grade &grade);
//start of main method int main() {
Grade s1,s2;
cout << "Enter for the first student:" << endl; GetInfo(s1);
cout << "Enter for the second student:" << endl; GetInfo(s2);
CalcGrade(s1); CalcGrade(s2);
cout << "The grades of the students are:" << endl; DispGrade(s1); DispGrade(s2);
return 0; }
// ====GetInfo================================================================= // This gets the information from the user // ============================================================================
void GetInfo(Grade &grade) { cout << "\tEnter student's full name: "; cin.getline(grade.name,80);
cout << "\tEnter Quiz 1 grade (out of 10):"; cin >> grade.quiz1;
cout << "\tEnter Quiz 2 grade (out of 10): "; cin >> grade.quiz2;
cout << "\tEnter midterm grade (out of 100): "; cin >> grade.midterm;
cout << "\tEnter final exam grade (out of 100): "; cin >> grade.finalExam;
cin.ignore(80,' '); }
// ====CalcGrade=============================================================== // This function calculates the grades // ============================================================================
void CalcGrade(Grade &grade) { CalcPercent(grade);
if(grade.percent>=90) grade.grade='A';
else if(grade.percent >=80 && grade.percent<90) grade.grade='B';
else if(grade.percent >=70 && grade.percent<80) grade.grade='C';
else if(grade.percent >=60 && grade.percent<70) grade.grade='D';
else grade.grade='F'; }
// ====CalcPercet============================================================== // This functino calculates the percentage // ============================================================================
void CalcPercent(Grade&grade) {
double q = double(grade.quiz1+grade.quiz2) / 20 * 25.0; double m = double(grade.midterm) / 100 * 25.0; double f = double(grade.midterm) / 100 * 50.0; grade.percent = q + m + f; }
// ====DispGrade=============================================================== // This function displays the grade // ============================================================================
void DispGrade(Grade &grade) { cout << fixed << setprecision(1);
cout << "\t" << grade.name << ":"
<< grade.percent
<< "%" << grade.grade << endl;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
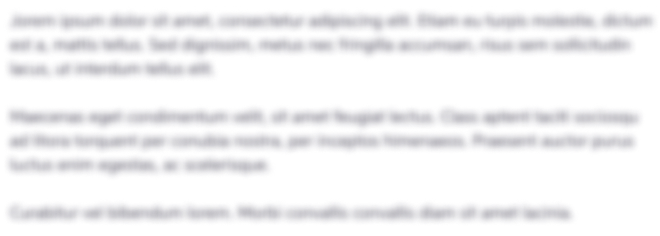
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started