Question
Render Conway's Game of Life in a .NET application . A grid should be rendered representing the individual cells. Cells can be turned on and
- Render Conway's Game of Life in a .NET application. A grid should be rendered representing the individual cells. Cells can be turned on and off by clicking on them with the mouse. Once a group of cells are turned on and the game runs they should live or die according the 4 rules of the game.
- Living cells with less than 2 living neighbors die in the next generation.
- Living cells with more than 3 living neighbors die in the next generation.
- Living cells with 2 or 3 living neighbors live in the next generation.
- Dead cells with exactly 3 living neighbors live in the next generation.
- Start, Pause and Next menu items. The game should start running by clicking on a Start menu item. The game should be pause by clicking on a Pause menu item. If currently paused, the game can be advanced 1 generation by clicking on a Next menu item.
- Emptying the universe.The universe should be emptied of all living cells through a New or Clear menu item.
Please answer the questions with the codes. If I could get clear codes that work fine, I'll appreciate that.
And instructions below are some instructions for this project, so might be helpful.
INSTRUCTIONS
- Cells- The heart of the game is a 2-d array of cells. A cell must be able to assume one of two different states: alive or dead. In its simplest form a cell could be represented by a Boolean. It might also be represented by a simple structure or class containing a Boolean. A structure or a class would allow the cell to store additional information like how many generations it's been alive.
- Universe and the Scratch Pad- Cells exist in a 2 dimensional universe. Programmatically the simplest representation of this universe would a 2-d array of cells. When the game starts running we will need two such arrays: one to represent the current universe and a second to function as a kind of scratch pad in which to calculate the next generation. Both arrays need to be the same size. Let's say 100 cells wide and 100 cells high. From here on out we'll refer to one as the universe and the second as the scratch pad.
- Next Generation- To calculate the next generation of cells we need to iterate through the universe using a couple of nested for loops and apply a few simple rules to decide whether the corresponding cell in the scratch pad should be turned on or off.
- Rules- The rules are very simple.
- Any living cell in the current universe with less than 2 living neighbors dies in the next generation as if by under-population. If a cell meets this criteria in the universe array then make the same cell dead in the scratch pad array.
- Any living cell with more than 3 living neighbors will die in the next generation as if by over-population. If so in the universe then kill it in the scratch pad.
- Any living cell with 2 or 3 living neighbors will live on into the next generation. If this is the case in the universe then the same cell lives in the scratch pad.
- Any dead cell with exactly 3 living neighbors will be born into the next generation as if by reproduction. If so in the universe then make that cell alive in the scratch pad.
- Counting Neighbors- The heart of a Game of life program is the counting of neighbors. It's the most complex operation that has to be performed. We'll probably want to make a function or method to do it; you should call it something obvious like CountNeighbors. It should take as two parameters the X and Y coordinate of the cell to be tested and it should return an integer between 0 and 8. It should simply tally up the number of living cells directly adjoining the test cell and return that value. Most cells in the universe array will have 8 neighbors, but not all of them. What about cells that exist on the universe edge? There are two common strategies for dealing with these boundary cells.
- The simplest approach is to treat every cell outside of the universe as dead. Most boundary cells will only have 5 neighbors; the three nonexistent neighbors will be assumed dead.
- A more complex approach, but one perhaps producing better results, is to treat the universe array as toroidal or wrapping around on itself. The left and right edges of the universe are connected to each other and so are the top and bottom edges. For boundary cells with only 5 true neighbors their other 3 neighbors exist on the opposite edge of the universe.
- Replace the Universe with the Scratchpad- After iterating through all the cells in the universe and applying the rules then the scratch pad will now contain the next generation. At this point simply replace the universe with the scratch pad before moving on to the next generation.
- Initial Random Universe- One requirement of the assignment will be to generate a random universe of cells. Start this byiterating through the universe array using two nested for loops. As we visit each cell generate a random number between 0 and 2 inclusive. If the random number's value is 0 then make that cell alive, otherwise make it dead. After this is completed the initial universe should be composed of roughly 1/3 living cells and 2/3 dead cells. Later on you can play around with the actual percentage of living to dead cells and see how that affects the game.
Various code fragments you might find useful in programming Game of Life.
HOW TO CENTER TEXT INSIDE A RECTANGLE...
Font font = new Font("Arial", 20f);
StringFormat stringFormat = new StringFormat();
stringFormat.Alignment = StringAlignment.Center;
stringFormat.LineAlignment = StringAlignment.Center;
Rectangle rect = new Rectangle(0,0,100,100)
; int neighbors = 8;
e.Graphics.DrawString(neighbors.ToString(), font, Brushes.Black, rect, stringFormat);
HOW TO SWAP TWO ARRAYS...
bool[,] universe = new bool[5, 5];
bool[,] scratchPad = new bool[5, 5];
// Swap them...
bool[,] temp = universe;
universe = scratchPad;
scratchPad = temp;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
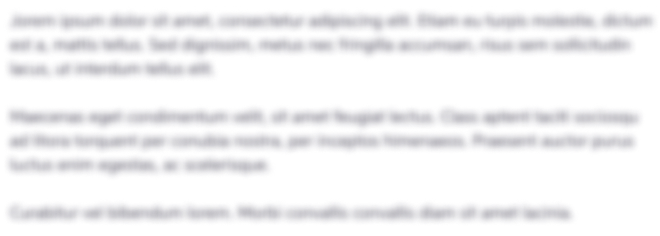
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started