Question
Requirement: You run four computer labs. Each lab contains certain number of computer stations to be determined at runtime. Your program should keep track of
Requirement:
You run four computer labs. Each lab contains certain number of computer stations to be determined at runtime.
Your program should keep track of the status of each computer station, whether it's empty or used by some user. Whenever a user (identified by 5-digit ID number) logs in or logs off, the program updates its data, and displays the new status of the lab.
In addition to the login and logoff, your program also supports a search command that looks up whether a certain user is using any computer station or not.
Your program then needs to dynamically allocate space for the current labs array of arrays using the entry in labsizes that corresponds (same index) to the labs[index] for the size. Please start with the starter code as it controls the Menu and showLabs displays.
Please implement the following functions:
void createArrays(IntPtr labs[], int labsizes[]); // should dynamically allocate the arrays.
void freeArrays(IntPtr labs[]); // should free the arrays
void search(IntPtr labs[], int labsizes[]); // should search for the user in the lab
void logout(IntPtr labs[], int labsizes[]); // should logout the user from the lab
Don't forget to add a comment at the top of the function to explain what it does.
=======================================================
Example output
An example execution of the program is displayed below:
Welcome to the LabMonitorProgram! Please enter the number of computer stations in each lab: How many computers in Lab 1?4 How many computers in Lab 2?5 How many computers in Lab 3?6 How many computers in Lab 4?4
MAIN MENU
0) Quit 1) Simulate login 2) Simulate logout 3) Search 1 Enter the 5 digit ID number of the user logging in: 33333 Enter the lab number the user is logging in from (1-4): 3 Enter computer station number the user is logging in to (1-6): 3 LAB STATUS Lab # Computer Stations 1 1: empty 2: empty 3: empty 4: empty 2 1: empty 2: empty 3: empty 4: empty 5: empty 3 1: empty 2: empty 3: 33333 4: empty 5: empty 6: empty 4 1: empty 2: empty 3: empty
MAIN MENU 0) Quit 1) Simulate login 2) Simulate logout 3) Search 1 Enter the 5 digit ID number of the user logging in: 22222 Enter the lab number the user is logging in from (1-4): 2 Enter computer station number the user is logging in to (1-5): 2 LAB STATUS Lab # Computer Stations 1 1: empty 2: empty 3: empty 4: empty 2 1: empty 2: 22222 3: empty 4: empty 5: empty 3 1: empty 2: empty 3: 33333 4: empty 5: empty 6: empty 4 1: empty 2: empty 3: empty
MAIN MENU 0) Quit 1) Simulate login 2) Simulate logout 3) Search 3 Enter the 5 digit ID number of the user logging in: 22222 User 22222 logged in Lab 2 at computer 2
MAIN MENU 0) Quit 1) Simulate login 2) Simulate logout 3) Search 2 Enter the 5 digit ID number of the user logging in: 11111 User not logged in. LAB STATUS Lab # Computer Stations 1 1: empty 2: empty 3: empty 4: empty 2 1: empty 2: 22222 3: empty 4: empty 5: empty 3 1: empty 2: empty 3: 33333 4: empty 5: empty 6: empty 4 1: empty 2: empty 3: empty 4: empty
MAIN MENU 0) Quit 1) Simulate login 2) Simulate logout 3) Search 2 Enter the 5 digit ID number of the user logging in: 33333 Logout user 33333 in Lab 3 at computer 3 LAB STATUS Lab # Computer Stations 1 1: empty 2: empty 3: empty 4: empty 2 1: empty 2: 22222 3: empty 4: empty 5: empty 3 1: empty 2: empty 3: empty 4: empty 5: empty 6: empty 4 1: empty 2: empty 3: empty 4: empty
MAIN MENU 0) Quit 1) Simulate login 2) Simulate logout 3) Search 0 Bye!
Code provided:
#include
using namespace std;
// Type definition typedef int* IntPtr;
// Constants const int NUMLABS = 4;
void createArrays(IntPtr labs[], int labsizes[]);
/* freeArrays: Releases memory we allocated with "new". */ void freeArrays(IntPtr labs[]);
void showLabs(IntPtr labs[], int labsizes[]);
void logout(IntPtr labs[], int labsizes[]);
void search(IntPtr labs[], int labsizes[]);
int main() { IntPtr labs[NUMLABS]; // store the pointers to the dynamic array for each lab int labsizes[NUMLABS]; // Number of computers in each lab int choice = -1; cout <<"Welcome to the LabMonitorProgram! ";
cout <<"Please enter the number of computer stations in each lab: "; for (int i=0; i< NUMLABS; i++) { do { cout <<"How many computers in Lab "<< i+1<<"?"; cin >> labsizes[i]; } while (labsizes[i]<0); }
// Create ragged array structure createArrays(labs, labsizes);
// Main Menu while (choice != 0) { cout << endl; cout << "MAIN MENU" << endl; cout << "0) Quit" << endl; cout << "1) Simulate login" << endl; cout << "2) Simulate logout" << endl; cout << "3) Search" << endl; cin >> choice; if (choice == 1) { login(labs, labsizes); showLabs(labs, labsizes); } else if (choice == 2) { logout(labs, labsizes); showLabs(labs, labsizes); } else if (choice == 3) { search(labs, labsizes); } }
freeArrays(labs); // Free memory before exiting cout << "Bye! "; return 0; }
/* ToDo: COMMENTS NEEDED HERE: void createArrays(IntPtr labs[], int labsizes[]) { //TO BE DONE BY STUDENTS //Hint: for each of the 4 labs, dynamically // loop for each lab and allocate an int array of size in labsizes // for that lab. // initialize each element in the array to -1 (unused computer). // store the array in the labs
}
void freeArrays(IntPtr labs[]) { //TO BE DONE BY STUDENTS }
void showLabs(IntPtr labs[], int labsizes[]) {
int i; int j;
cout << "LAB STATUS" << endl; cout << "Lab # Computer Stations" << endl; for (i=0; i < NUMLABS; i++) { cout << i+1 << " "; for (j=0; j < labsizes[i]; j++) { cout << (j+1) << ": "; if (labs[i][j] == -1) { cout << "empty "; } else { cout << labs[i][j] << " "; } } cout << endl; } cout << endl; return; }
void login(IntPtr labs[], int labsizes[]) { int id, lab, num = -1;
// read user id do { cout << "Enter the 5 digit ID number of the user logging in:" << endl; cin >> id; } while ((id < 10000) || (id > 99999));
// read the lab number do { cout << "Enter the lab number the user is logging in from (1-" << NUMLABS << "):" << endl; cin >> lab; } while ((lab <= 0) || (lab > NUMLABS));
do { cout << "Enter computer station number the user is logging in to " << "(1-" << labsizes[lab-1] << "):" << endl; cin >> num; } while ((num <= 0) || (num > labsizes[lab-1]));
// Check to see if this station is free if (labs[lab-1][num-1]!=-1) { cout << "ERROR, user " << labs[lab-1][num-1] << " is already logged into that station." << endl; return; } // Assign this station to the user labs[lab-1][num-1] = id; return; }
/* ToDo: COMMENTS NEEDED HERE: Describe how your function does its job, as required by the comments given for the declaration of the function. */ void logout(IntPtr labs[], int labsizes[]) {
} void search(IntPtr labs[], int labsizes[]) { //TO BE DONE BY STUDENTS.
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
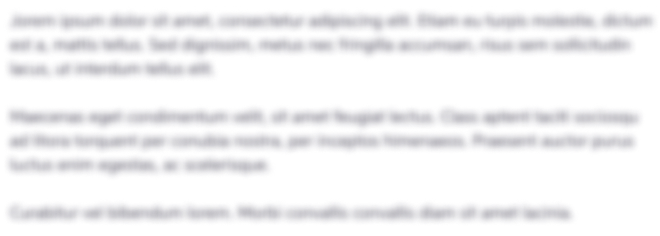
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started