Question
REQUIREMENTS Part 1: Implement your Thing class Start by choosing what sort of list you would like to keep. For example: Book information Vacation and
REQUIREMENTS
Part 1: Implement your Thing class
Start by choosing what sort of list you would like to keep. For example:
Book information
Vacation and travel information
Pets
Real estate properties
pretty much any sort of list
You can be creative about your list. It is prohibited to used grocery items, student, bank accounts, or songs. It may be possible to use reuse code across more than one programming assignment, so try to choose something that interests you.
Implement the sort of Thing to be stored on your list as a class. The class must have the following characteristics:
At least 5 instance variables
At least one numeric attribute
At least one String attribute
One String attribute should be usable as a search key
All instance variable must be private
Implement getters and setters for all the attributes of your Thing.
Implement the equals method for your Thing.
Part 2: Implement your Collection class
1- Implement a collection class of things using an array. You may NOT use the Java library classes ArrayList or Vector or any other java collection class. You must use simple java Arrays. The name of your collection class should include the name of your Thing. For example, if your Thing is called Country, then your collection class should be called CountryArrayBag.
2- Implement the constructor which accepts as an argument the maximum number of elements that can be stored in your collection.
3- Implement the following methods on your collection class:
insert:takesasinputvaluesforalltheinstancevariablesandcreatesanobjectand
store in the collection.
display: displays all the elements that are stored in the collection. The displayed
list should accurately reflect the items stored in the data structure. That is if an item
is updated or deleted, the displayed list should show that.
search: takes as input a search key then searches the list for a matching object.
Then, retrieves and displays all the information for the matching object. The search key should be key insensitive. For example, ITEM, item and Item match each other. Your search key must be a String not a number.
delete: takes as input a search key by the user, searches your list for a matching object and delete the matching object.
update:takesasinputasearchkeybytheuser,searchyourlistforamatchingobject, present the current object's data to the user and allow the user to change any field (or fields) for that item. Be very careful to not break the encapsulation of the classes. You may wish to find the item in the list, make a copy of that item, then delete the item from the list, allow the user to change, and then add the new item to the list.
size: display the number of items in the list.
Your code must check for errors, such as entering text in a numeric field or entering more items than can be held in the array of objects. Gracefully recover from any problem without crashing the program. You may display error messages to the user in case of any problem.
Implement a Driver class that includes at least one call for each one of the methods you implemented. You can just use any arbitrary input values in your methods calls in your driver and you do not have to read the inputs from the user.
Part 3: Graphical User Interface (GUI)
As discussed in class, you are given a template for your GUI implementation. You are free to either use the suggested interface or to change it as much as you would like. Just make sure that your interface is intuitive and easy to use.
/////////////////////////////////////////////////////////////////////////////////////////////////////////
Here is what I have so far:
FishArrayBag Class:
public class FishArrayBag {
//instance variables
private Fish[] fishList;
private int numFish;
//constructor
public FishArrayBag(int maxFish) {
fishList = new Fish[maxFish];
}
//method to add a fish to the list
public void addFish(Fish f) {
this.fishList[this.numFish] = f;
this.numFish ++;
}
//method to delete a fish
public void removeFish(int idNum) {
int index = getIndexOf(idNum);
Fish result = removeEntry(index);
}
private Fish removeEntry(int index) {
Fish result =null;
if (index >=0)
{
result = fishList[index];
numFish--;
fishList[index] = fishList[numFish];
fishList[numFish]=null;
}
return result;
}
//method to return the index of idNum
private int getIndexOf(Fish idNum) {
int index = getIndexOf(idNum);
return index;
}
//method to search for a fish
public String searchFish(int idNum) {
String output = "Fish not found.";
for (int i = 0; i if(this.fishList[i].getIdentifier() == idNum) output = this.fishList[i].toString(); System.out.println(output); } return output; } //method to print all items of the list //method to update a specific item of the list public boolean replace(Fish idNum, Fish newItem) { int index =this.getIndexOf(idNum); if (index>=0) { fishList[index] =newItem; return true; } return false; } } FishCollectionInterface Class: import FishCollectionInterface.InsertListenerClass.SearchListenerClass; import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.control.TextArea; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.stage.Stage; public class FishCollectionInterface extends Application { FishArrayBag list = new FishArrayBag(10); Stage window; // Scenes Scene homeScene; Scene insertScene; Scene searchScene; Scene deleteScene; Scene updateScene; // Panes, each scene needs a pane HBox homePane; VBox insertPane; VBox searchPane; HBox updatePane; HBox deletePane; // Buttons in the home scene Button insertBtn; Button searchBtn; Button deleteBtn; Button updateBtn; Button sizeBtn; Button displayBtn; // Buttons to return to Home menu Button homeBtnInsert; Button homeBtnSearch; // Insert Scene items TextField insert_id_TF; TextField insert_common_name_TF; TextField insert_scientific_name_TF; TextField insert_max_size_TF; TextField insert_max_temp_TF; TextField insert_id_res; // Search Scene items TextField search_id_TF; TextArea search_result; public static void main(String[] args) { Application.launch(args); } // A method to switch to the appropriate scene when a button is clicked class ButtonListenerClass implements EventHandler @Override public void handle(ActionEvent e) { if (e.getSource() == insertBtn) { window.setScene(insertScene); } else if (e.getSource() == homeBtnInsert) { window.setScene(homeScene); } else if (e.getSource() == homeBtnSearch) { window.setScene(homeScene); } else if (e.getSource() == searchBtn) { window.setScene(searchScene); } } } @Override public void start(Stage primaryStage){ window = primaryStage; //Buttons to open the various scenes insertBtn = new Button("Insert"); searchBtn = new Button("Search"); deleteBtn = new Button("Delete"); updateBtn = new Button("Update"); displayBtn = new Button("Display"); sizeBtn = new Button("Size"); homeBtnInsert = new Button("Home"); homeBtnSearch = new Button("Home"); ButtonListenerClass btnListener = new ButtonListenerClass(); insertBtn.setOnAction(btnListener); searchBtn.setOnAction(btnListener); deleteBtn.setOnAction(btnListener); updateBtn.setOnAction(btnListener); displayBtn.setOnAction(btnListener); sizeBtn.setOnAction(btnListener); homeBtnInsert.setOnAction(btnListener); homeBtnSearch.setOnAction(btnListener); //Home scene Design //The home scene contains the main interface buttons homePane = new HBox(); homePane.getChildren().add(insertBtn); homePane.getChildren().add(searchBtn); homePane.getChildren().add(deleteBtn); homePane.getChildren().add(updateBtn); homePane.getChildren().add(displayBtn); homePane.getChildren().add(sizeBtn); homeScene = new Scene(homePane, 500, 300); primaryStage.setTitle("Collection Interface"); primaryStage.setScene(homeScene); primaryStage.show(); ////////////////////////////////////////////// //Insert Scene Design //creating visual items Label idLabel = new Label("ID:"); Label commonNameLabel = new Label("Common Name:"); Label scientificNameLabel = new Label("Scientific Name:"); Label maxSizeLabel = new Label("Maximum Size:"); Label maxTempLabel = new Label("Maximum Temp:"); Button fishInsert = new Button("Insert"); //Insert Text field //Text fields are declared as instance variables not local variables // because the listeners need to read from and write to text fields insert_id_TF = new TextField("Enter ID"); insert_common_name_TF = new TextField("Enter Common Name"); insert_scientific_name_TF = new TextField("Enter Scientific Name"); insert_max_size_TF = new TextField("Enter Max Size"); insert_max_temp_TF = new TextField("Enter Max Temp"); //Creating insert pane and adding items to it insertPane = new VBox(); insertPane.getChildren().add(homeBtnInsert); insertPane.getChildren().add(idLabel); insertPane.getChildren().add(insert_id_TF); insertPane.getChildren().add(commonNameLabel); insertPane.getChildren().add(insert_common_name_TF); insertPane.getChildren().add(scientificNameLabel); insertPane.getChildren().add(insert_scientific_name_TF); insertPane.getChildren().add(maxSizeLabel); insertPane.getChildren().add(insert_max_size_TF); insertPane.getChildren().add(maxTempLabel); insertPane.getChildren().add(insert_max_temp_TF); insertPane.getChildren().add(fishInsert); InsertListenerClass fishInsertListener = new InsertListenerClass(); fishInsert.setOnAction(fishInsertListener); //adding insert pane to insert scene insertScene = new Scene(insertPane, 300, 300); ////////////////////////////////////////////// //Search Scene Design Label searchLabel = new Label("ID:"); Button studentSearch = new Button("Search"); search_id_TF = new TextField("Enter ID"); search_result = new TextArea(); search_result.setEditable(false); //Creating search pane and adding items to it searchPane = new VBox(); searchPane.getChildren().add(homeBtnSearch); searchPane.getChildren().add(searchLabel); searchPane.getChildren().add(search_id_TF); searchPane.getChildren().add(studentSearch); searchPane.getChildren().add(search_result); SearchListenerClass searchListenerClass = new SearchListenerClass(); fishSearch.setOnAction(searchListenerClass); //adding insert pane to insert scene searchScene = new Scene(searchPane, 300, 300); } // A listener to be called to insert a fish in the collection class InsertListenerClass implements EventHandler @Override public void handle(ActionEvent ae) { System.out.println("Insert Button Clicked"); String id = insert_id_TF.getText(); String commonName2 = insert_common_name_TF.getText(); String scientificName2 = insert_scientific_name_TF.getText(); String maxSize = insert_max_size_TF.getText(); String maxTemp = insert_max_temp_TF.getText(); int idNum = Integer.parseInt(id); double maxSize2 = Double.parseDouble(maxSize); int maxTemp2 = Integer.parseInt(maxTemp); System.out.println(id + " "+commonName2 + " " +scientificName2 + " " +maxSize2 + "" +maxTemp2); Fish newFish = new Fish(idNum, commonName2, scientificName2, maxSize2, maxTemp2); list.addFish(newFish); } // A listener to be called to insert a fish into the collection class SearchListenerClass implements EventHandler private int idNum; @Override public void handle(ActionEvent ae) { System.out.println("Search Button Clicked"); String idStr = search_id_TF.getText(); int idNum = Integer.parseInt(idStr); search_result.setText(list.searchFish(this.idNum)); } } } } Fish Class: public class Fish { //instance variables private int identifier; private String commonName; private String scientificName; private double maxSize; private double maxTemp; //constructor for the fish class public Fish(int idNum, String commonName2, String scientificName2, double maxSize2, int maxTemp2) { this.identifier = idNum; this.commonName = commonName2; this.scientificName = scientificName2; this.maxSize = maxSize2; this.maxTemp = maxTemp2; } public int getIdentifier() { return identifier; } public void setIdentifier(int identifier) { this.identifier = identifier; } public String getCommonName() { return commonName; } public void setCommonName(String commonName) { this.commonName = commonName; } public String getScientificName() { return scientificName; } public void setScientificName() { this.scientificName = scientificName; } public double getMaxSize() { return maxSize; } public void setMaxSize(int maxSize) { this.maxSize = maxSize; } public double getMaxTemp() { return maxTemp; } public void setMaxTemp(double setMaxTemp) { this.maxTemp = maxTemp; } @Override public String toString(){ String output = ""; output +=this.identifier+"\t"; output += this.commonName + "\t"; output += this.scientificName + "\t"; output += this.maxSize + "\t"; output += this.maxTemp; return output; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
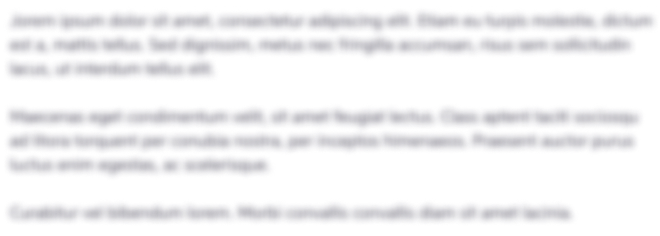
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started