Question
Rock Paper Scissors game - implement some methods for it The childhood method of resolving a dispute was to play Rock, Paper, Scissors. Rock beats
Rock Paper Scissors game - implement some methods for it
The childhood method of resolving a dispute was to play Rock, Paper, Scissors.
Rock beats scissors, scissors beats paper, and paper beats rock. If you tie, you play again until the tie is broken. Players say "Ro sham bo" and then show their hand.
Fill in the methods play(), eval(), getUserHand() per the instructions to get a working game you can play against the computer.
import java.util.Random; import java.util.Scanner;
// This class asks the user to write a method doing the comparison // of two hands for the game Rock, Paper, Scissors. class Main { // Create enumeration constants for the types of hands. public enum Hand { ROCK, PAPER, SCISSORS } // Create enumeration constants for the outcomes of who wins. public enum Winner { COMPUTER, TIE, USER }
// Additional private member variables used in the game playing class. private Random random; private Scanner scan; // Constructor that takes the command line arguments to look // for an integer random seed to start the computer's hand. public Main(String[] args) { // Set up the random number generator. if (args.length == 1) { long seed = Long.parseLong(args[0]); random = new Random(seed); } else { random = new Random(); } // Set up the input scanner. scan = new Scanner(System.in); } // Method to play a round of RPS. void play() { TODO: Get a hand from the computer by calling getComputerHand(); TODO: Get a hand from the user by calling getUserHand(); TODO: Pass the return values from those two methods to printWinner(). } // Compute and print the winner of the match, given a hand for each // of computer and user. Handle the error case of indeterminate winner. void printWinner(Hand computerHand, Hand userHand) { Winner winner = eval(computerHand, userHand); System.out.println("C: " + computerHand + " vs U: " + userHand); switch (winner) { case COMPUTER: System.out.println("Computer wins."); break; case TIE: System.out.println("It's a tie."); break; case USER: System.out.println("User wins."); break; default: System.out.println("Error, no one wins!"); break; } } // Evaluate a pair of hands to see which wins. Use the typical ordering of // rock > scissors > paper > rock. Winner eval(Hand computerHand, Hand userHand) { TODO: Use Boolean logic on (computerHand == Hand.ROCK) and the other TODO: possible hands, also for userHand, to calculate who wins and then TODO: return Winner.COMPUTER, Winner.USER, or Winner.TIE depending on who wins. } // Get a random computer hand. private Hand getComputerHand() { int num = (int) (random.nextDouble() * 3.0); if (num == 0) { return Hand.ROCK; } else if (num == 1) { return Hand.PAPER; } else { return Hand.SCISSORS; } } // Get a user hand from the scanner. private Hand getUserHand() { System.out.println("Let's play Rock/Paper/Scissors."); System.out.println("What's your hand, rock/paper/scissors?"); // Scanner reads keyboard for words "rock", "paper", or "scissors". TODO: read the next token from the keyboard (scan) and return TODO: one of Hand.ROCK, Hand.PAPER, or Hand.SCISSORS TODO: depending on the user's input. } // Method to set up the Main object, apply a computer hand, // prompt for user hand, evaluate the hands, and print result. // Run from the command line with no arguments, or with a single // positive long integer to seed the random number generator. public static void main(String[] args) { Main rpsPlayer = new Main(args); rpsPlayer.play(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
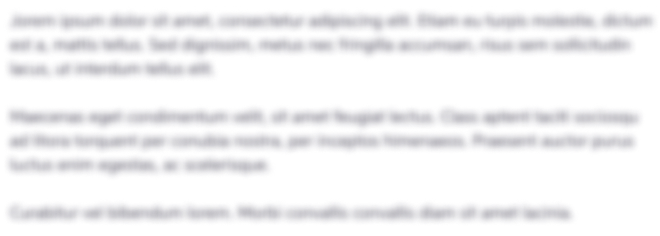
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started