Question
Rock/Paper/Scissors Game You are part of a development team that is creating a program which which simulates the rock, paper, scissors game. The rules for
Rock/Paper/Scissors Game
You are part of a development team that is creating a program which which simulates the rock, paper, scissors game. The rules for the game: Rock beats scissors. Paper beats Rock. Scissors beats Paper. The development team's lead programmer has chosen to use a driver and stubs for the application's initial prototype. She has asked you to finish the initial program which will serve as an alpha-prototype.
Requirements: (1) Global constants are defined at the top of the program. These constants must be used. (2) No function parameters are to be changed. The parameters being passed to the function and returned by the function must remain intact. and (3) the main() function is not to be changed.
Here's the original code for the assignment:
#include
// Global Constants const int ROCK=0, PAPER=1, SCISSORS=2; const int COMPUTER=0, PLAYER=1, TIE=2;
// Function Prototypes int playerInput(); int computerInput(); int chooseWinner(int, int); void displayResults(int, int, int);
//FUNCTION main int main(){ int computer, player,winner; // INTRODUCTION cout<<" Welcome to the Rock, Paper, Scissors Game " <<"----------------------------------------- "; player = playerInput(); computer = computerInput(); winner = chooseWinner(player, computer); displayResults(player, computer, winner); return 0; }
/****************** FUNCTION playerInput The purpose of this function is to solicit user input in selecting Rock, Scissors or Paper No parameters are sent to the function. The function returns the user's input as an integer in the form of one of the pre-defined constants. **********************/ int playerInput(){ // FUNCTION SPECIFICATION // 1. LOOP USED TO VALIDATE USER INPUT // 2. PLAYER INPUTS ROCK, PAPER, SCISSORS VALUE // 3. DISPLAY PLAYER'S CHOICE CHOICE AS ROCK PAPER SCISSORS // 4. RETURNS INTEGER VALUE OF ROCK, PAPER, SCISSORS CHOSEN BY PLAYER return ROCK;// Possible Values: ROCK(0) or PAPER(1) or SCISSORS(2) }
/****************** FUNCTION computerInput The purpose of this function is to generate random input in the form of Rock, Scissors or Paper No parameters are sent to the function. The function returns the commputer's selection in the form of one of the pre-defined constants. **********************/ int computerInput(){ // CRITERIA // 1. COMPUTER RANDOMLY GENERATES ROCK, PAPER, SCISSORS VALUE // 2. DISPLAY COMPUTER'S CHOICE AS ROCK PAPER SCISSORS // 3. RETURNS INTEGER VALUE OF ROCK, PAPER, SCISSORS CHOSEN BY PLAYER return ROCK; // Possible Values: ROCK(0) or PAPER(1) or SCISSORS(2) }
/****************** FUNCTION chooseWinner The purpose of this function is to compare the values generated by User and Computer and return a constant indicating winner or tie. Parameters for user selection and random computer selection are sent to the function. The function returns the winner in the form of one of the pre-defined constants. **********************/ int chooseWinner(int, int){ // CRITERIA // 1. COMPARE PLAYER AND COMPUTER SELECTIONS // USING RULES FOR ROCK PAPER SCISSOR // 2. RETURNS Integer COMPUTER, PLAYER, OR TIE. return TIE; //COMPUTER=0, PLAYER=1, TIE=2; }
/****************** FUNCTION displayResults The purpose of this function is to display the selections of the user, computer, and the winner
Parameters for user selection, random computer selection, and winner are sent to the function in the form of integer constants. The function does not return a value. **********************/ void displayResults(int, int, int){ // CRITERIA - Feel free to incorporate text-based graphics // 1. Display player's choice // 2. Display the computer's choice // 3. Display winner/tie }
Here's the code for the program so far:
#include
// Global Constants const int ROCK = 0, PAPER = 1, SCISSORS = 2; const int COMPUTER = 0, PLAYER = 1, TIE = 2;
// Function Prototypes int playerInput(); int computerInput(); int chooseWinner(int, int); void displayResults(int, int, int);
//FUNCTION main int main() { int computer, player, winner; // INTRODUCTION cout << " Welcome to the Rock, Paper, Scissors Game " << "----------------------------------------- "; player = playerInput(); computer = computerInput(); winner = chooseWinner(player, computer); displayResults(player, computer, winner); return 0; }
/****************** FUNCTION playerInput
The purpose of this function is to solicit user input in selecting Rock, Scissors or Paper
No parameters are sent to the function. The function returns the user's input as an integer in the form of one of the pre-defined constants.
**********************/ int playerInput() { // FUNCTION SPECIFICATION // 1. LOOP USED TO VALIDATE USER INPUT // 2. PLAYER INPUTS ROCK, PAPER, SCISSORS VALUE // 3. DISPLAY PLAYER'S CHOICE CHOICE AS ROCK PAPER SCISSORS // 4. RETURNS INTEGER VALUE OF ROCK, PAPER, SCISSORS CHOSEN BY PLAYER
int player;
cout << "Enter 0 for ROCK, 1 for PAPER, or 2 for SCISSORS >> " << endl; cin >> player;
while (player < 0 && player > 2) { cout << "Invalid integer." << endl; cout << "Enter 0 for ROCK, 1 for PAPER, or 2 for SCISSORS >> "; cin >> player; }
if (player == 0) cout << "You choose ROCK!" << endl; else if (player == 1) cout << "You choose PAPER!" << endl; else if (player == 2) cout << "You choose SCISSORS!" << endl; else cout << "Error." << endl;
return player; // Possible Values: ROCK(0) or PAPER(1) or SCISSORS(2) }
/****************** FUNCTION computerInput
The purpose of this function is to generate random input in the form of Rock, Scissors or Paper
No parameters are sent to the function. The function returns the commputer's selection in the form of one of the pre-defined constants.
**********************/
int computerInput() { // CRITERIA // 1. COMPUTER RANDOMLY GENERATES ROCK, PAPER, SCISSORS VALUE // 2. DISPLAY COMPUTER'S CHOICE AS ROCK PAPER SCISSORS // 3. RETURNS INTEGER VALUE OF ROCK, PAPER, SCISSORS CHOSEN BY PLAYER
// Random int between 0 and 2 int computer = (rand() % 3);
if (computer == 0) cout << "The computer chooses ROCK!" << endl; else if (computer == 1) cout << "The computer chooses PAPER!" << endl; else if (computer == 2) cout << "The computer chooses SCISSORS!" << endl; else cout << "Error." << endl;
return computer; // Possible Values: ROCK(0) or PAPER(1) or SCISSORS(2) }
/****************** FUNCTION chooseWinner
The purpose of this function is to compare the values generated by User and Computer and return a constant indicating winner or tie.
Parameters for user selection and random computer selection are sent to the function. The function returns the winner in the form of one of the pre-defined constants.
**********************/
int chooseWinner(int player, int computer) { // CRITERIA // 1. COMPARE PLAYER AND COMPUTER SELECTIONS // USING RULES FOR ROCK PAPER SCISSOR // 2. RETURNS Integer COMPUTER, PLAYER, OR TIE.
if (player == computer) return TIE;
if (player == ROCK && computer == SCISSORS) return PLAYER; else if (player == PAPER && computer == ROCK) return PLAYER; else if (player == SCISSORS && computer == PAPER) return PLAYER;
return COMPUTER; //COMPUTER=0, PLAYER=1, TIE=2; }
/****************** FUNCTION displayResults
The purpose of this function is to display the selections of the user, computer, and the winner
Parameters for user selection, random computer selection, and winner are sent to the function in the form of integer constants. The function does not return a value.
**********************/ void displayResults(int player, int computer, int winner) { // CRITERIA - Feel free to incorporate text-based graphics // 1. Display player's choice // 2. Display the computer's choice // 3. Display winner/tie cout << endl; if (player == 0) cout << "You choose ROCK!" << endl; else if (player == 1) cout << "You choose PAPER!" << endl; else if (player == 2) cout << "You choose SCISSORS!" << endl;
if (computer == 0) cout << "The computer chooses ROCK!" << endl; else if (computer == 1) cout << "The computer chooses PAPER!" << endl; else if (computer == 2) cout << "The computer chooses SCISSORS!" << endl;
if (winner == PLAYER) cout << "The winner is you!" << endl; else if (winner == COMPUTER) cout << "The winner is the computer!" << endl; else cout << "It's a tie!" << endl; }
I wrote code for a random preprocessor directive and the playerInput, computerInput, chooseWinner, and displayResults functions. In the playerInput function, I'm unclear why the while loop isn't iterating until the player inputs 0, 1, or 2. That's as far as I can solve for this assignment. I appreciate your coding assistance to produce the assignment's result!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
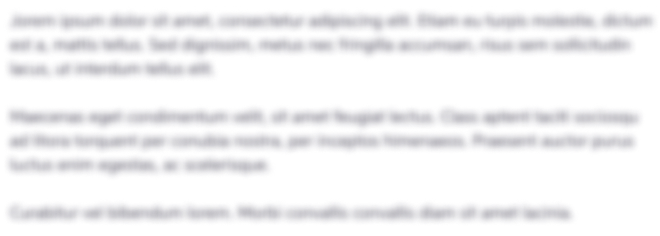
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started