Rollable interface: public interface Rollable { public void rollUp(); public void rollDown(); } CounterDisplay interface: public interface CounterDisplay { public void reset(); public void shuffle();
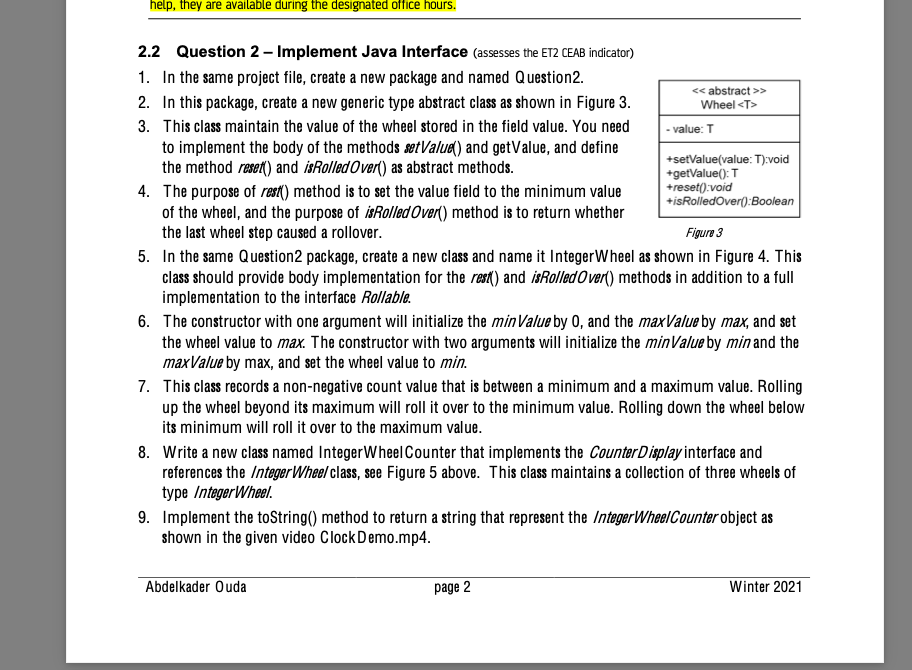
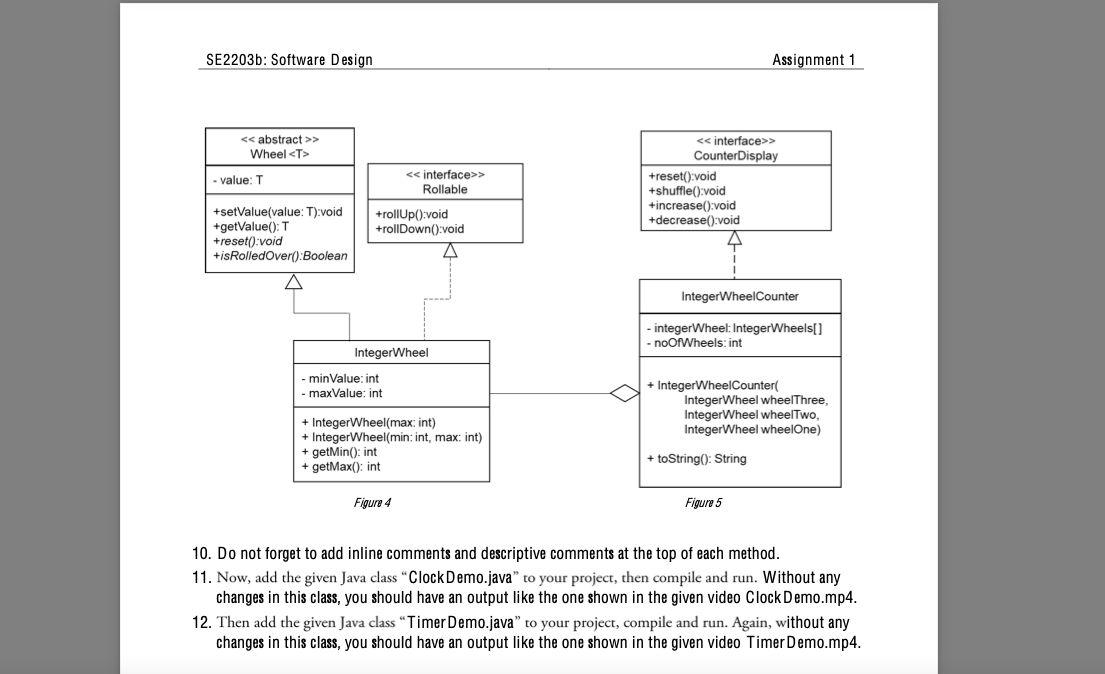
Rollable interface:
public interface Rollable { public void rollUp(); public void rollDown(); }
CounterDisplay interface:
public interface CounterDisplay { public void reset(); public void shuffle(); public void increase(); public void decrease(); }
ClockDemo.java class:
package Question2; import java.util.Timer; import java.util.TimerTask; public class ClockDemo extends TimerTask { static IntegerWheel seconds = new IntegerWheel(0, 59); static IntegerWheel minutes = new IntegerWheel(0, 59); static IntegerWheel hours = new IntegerWheel(0, 23); static IntegerWheelCounter theClock = new IntegerWheelCounter(hours, minutes, seconds); public void run() { System.out.print(" " + theClock); theClock.increase(); } public static void main(String[] args) { System.out.println("Wheels based clock"); System.out.println("=================="); System.out.println("HH:MM:SS"); // Starts at any random time theClock.shuffle(); Timer timer = new Timer(); timer.schedule(new ClockDemo(), 0, 1000); } }
TimerDemo.java class:
package Question2; import java.util.Timer; import java.util.TimerTask; public class TimerDemo extends TimerTask { static IntegerWheel seconds = new IntegerWheel(20); static IntegerWheel minutes = new IntegerWheel(0); static IntegerWheel hours = new IntegerWheel(0); static IntegerWheelCounter theTimer = new IntegerWheelCounter(hours, minutes, seconds); static Timer timer = new Timer(); public void run() { System.out.print(" " + theTimer); if (theTimer.toString().equals("00:00:00") ) { { timer.cancel(); timer.purge(); return; } } theTimer.decrease(); } public static void main(String[] args) { System.out.println("Wheels based timer"); System.out.println("=================="); timer.schedule(new TimerDemo(), 0, 1000); } }
Please do not change the code in ClockDemo and TimerDemo. It should work without changing them. The outputs should look exactly like the following:
For ClockDemo it should be like this and the seconds should change until the clock reads 17:29:02 :
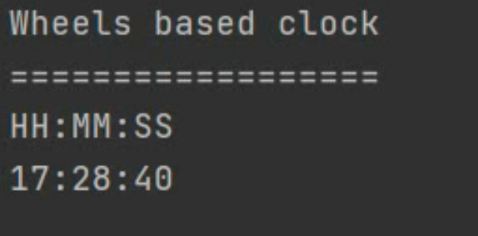
For TimerDemo it should be like this and the seconds should change until the clock reads 00:00:00 :
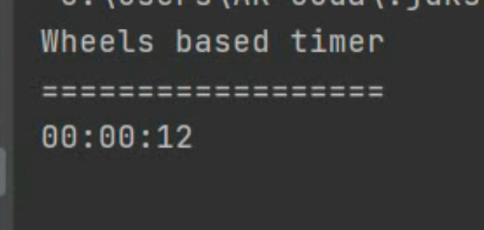
help, they are available during the designated office hours. 2.2 Question 2 - Implement Java Interface (assesses the ET2 CEAB indicator) 1. In the same project file, create a new package and named Question2. > 2. In this package, create a new generic type abstract class as shown in Figure 3. Wheel 3. This class maintain the value of the wheel stored in the field value. You need value: T to implement the body of the methods set Value) and getValue, and define the method reset() and isRolledOvert) as abstract methods. +setValue(value: T):void +getValue(): T 4. The purpose of rest() method is to set the value field to the minimum value +reset():void +isRolledOver(): Boolean of the wheel, and the purpose of isRolled Over() method is to return whether the last wheel step caused a rollover. Figure 3 5. In the same Question package, create a new class and name it Integer Wheel as shown in Figure 4. This class should provide body implementation for the rest) and isRolled Over() methods in addition to a full implementation to the interface Rollable. 6. The constructor with one argument will initialize the minValue by 0, and the maxValue by max, and set the wheel value to max. The constructor with two arguments will initialize the minValue by min and the maxValue by max, and set the wheel value to min. 7. This class records a non-negative count value that is between a minimum and a maximum value. Rolling up the wheel beyond its maximum will roll it over to the minimum value. Rolling down the wheel below its minimum will roll it over to the maximum value. 8. Write a new class named Integer Wheel Counter that implements the Counter Display interface and references the Integer Wheel class, see Figure 5 above. This class maintains a collection of three wheels of type Integer Wheel. 9. Implement the toString() method to return a string that represent the Integer Wheel Counter object as shown in the given video Clock Demo.mp4. Abdelkader Ouda page 2 Winter 2021 SE2203b: Software Design Assignment 1 > Wheel value: T > Rollable > Counter Display +reset():void +shuffle():void +increase():void +decrease():void +setValue(value: T):void +getValue(): T +reset():void +isRolledOver(): Boolean +rollUp():void +rollDown():void A IntegerWheelCounter - integer Wheel: IntegerWheels[] - noOfWheels: int Integer Wheel - minValue: int - maxValue: int + Integer Wheel Counter IntegerWheel wheelThree, Integer Wheel wheelTwo, Integer Wheel wheelOne) + IntegerWheel(max: int) + Integer Wheel(min:int, max: int) + getMin(): int + getMax(): int + toString(): String Figure 4 Figure 5 10. Do not forget to add inline comments and descriptive comments at the top of each method. 11. Now, add the given Java class "Clock Demo.java to your project, then compile and run. Without any changes in this class, you should have an output like the one shown in the given video Clock Demo.mp4. 12. Then add the given Java class Timer Demo.java" to your project, compile and run. Again, without any changes in this class, you should have an output like the one shown in the given video Timer Demo.mp4. Wheels based clock %3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D HH:MM:SS 17:28:40 Wheels based timer 00:00:12 help, they are available during the designated office hours. 2.2 Question 2 - Implement Java Interface (assesses the ET2 CEAB indicator) 1. In the same project file, create a new package and named Question2. > 2. In this package, create a new generic type abstract class as shown in Figure 3. Wheel 3. This class maintain the value of the wheel stored in the field value. You need value: T to implement the body of the methods set Value) and getValue, and define the method reset() and isRolledOvert) as abstract methods. +setValue(value: T):void +getValue(): T 4. The purpose of rest() method is to set the value field to the minimum value +reset():void +isRolledOver(): Boolean of the wheel, and the purpose of isRolled Over() method is to return whether the last wheel step caused a rollover. Figure 3 5. In the same Question package, create a new class and name it Integer Wheel as shown in Figure 4. This class should provide body implementation for the rest) and isRolled Over() methods in addition to a full implementation to the interface Rollable. 6. The constructor with one argument will initialize the minValue by 0, and the maxValue by max, and set the wheel value to max. The constructor with two arguments will initialize the minValue by min and the maxValue by max, and set the wheel value to min. 7. This class records a non-negative count value that is between a minimum and a maximum value. Rolling up the wheel beyond its maximum will roll it over to the minimum value. Rolling down the wheel below its minimum will roll it over to the maximum value. 8. Write a new class named Integer Wheel Counter that implements the Counter Display interface and references the Integer Wheel class, see Figure 5 above. This class maintains a collection of three wheels of type Integer Wheel. 9. Implement the toString() method to return a string that represent the Integer Wheel Counter object as shown in the given video Clock Demo.mp4. Abdelkader Ouda page 2 Winter 2021 SE2203b: Software Design Assignment 1 > Wheel value: T > Rollable > Counter Display +reset():void +shuffle():void +increase():void +decrease():void +setValue(value: T):void +getValue(): T +reset():void +isRolledOver(): Boolean +rollUp():void +rollDown():void A IntegerWheelCounter - integer Wheel: IntegerWheels[] - noOfWheels: int Integer Wheel - minValue: int - maxValue: int + Integer Wheel Counter IntegerWheel wheelThree, Integer Wheel wheelTwo, Integer Wheel wheelOne) + IntegerWheel(max: int) + Integer Wheel(min:int, max: int) + getMin(): int + getMax(): int + toString(): String Figure 4 Figure 5 10. Do not forget to add inline comments and descriptive comments at the top of each method. 11. Now, add the given Java class "Clock Demo.java to your project, then compile and run. Without any changes in this class, you should have an output like the one shown in the given video Clock Demo.mp4. 12. Then add the given Java class Timer Demo.java" to your project, compile and run. Again, without any changes in this class, you should have an output like the one shown in the given video Timer Demo.mp4. Wheels based clock %3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D%3D HH:MM:SS 17:28:40 Wheels based timer 00:00:12