Question
Ruby Programming on Classes Task: Design and implement a car class that contains various methods and variables to replicate real-world functionality In considering a Car
Ruby Programming on Classes
Task: Design and implement a car class that contains various methods and variables to replicate real-world functionality
In considering a Car class, there is obviously a wide variety of information that we
could capture using instance variables. For this assignment, let's limit our instance
variables to encapsulate the make, model, and year.
For those instance variables, there are also some operations we want to conduct
using those values. We want to be able to retrieve and print out those values, initialize those values to some beginning state, and modify those values by reassigning them. We can do all those operations by defining instance methods in our Car class that accomplish those tasks.
So, our Car class design might look like this by way of a pseudo-code description:
Car instance variables
----------------------
make
model
year
Car instance methods
--------------------
get_make
get_model
get_year
set_make
set_model
set_year
__________________________
Criteria: Program should run like this:
$ classcar.rb
How many cars do you want to create? 2
Enter make for car 1: AstonMartin
Enter model for car 1: DB5
Enter year for car 1: 1962
Enter make for car 2: McLaren
Enter model for car 2: F1
Enter year for car 2: 2014
You have the following cars:
1962 AstonMartin DB5
2014 McLaren F1
$
________________________
Given Ruby File:
# DEFINE YOUR CAR CLASS HERE
# MAIN PORTION OF PROGRAM
# create empty array
array_of_cars = Array.new
# prompt for and get number of cars
print "How many cars do you want to create? "
num_cars = gets.to_i
# create that many cars
for i in 1..num_cars
# get the make, model, and year
puts
print "Enter make for car #{i}: "
make = gets.chomp
print "Enter model for car #{i}: "
model = gets.chomp
print "Enter year of car #{i}: "
year = gets.to_i
# create a new Car object
c = Car.new
# set the make, model, and year
# that we just read in
c.set_make(make)
c.set_model(model)
c.set_year(year)
# add the Car object to the array
array_of_cars << c
end
# print out the information for
# the Car objects in the array
puts
puts "You have the following cars: "
for car in array_of_cars
puts "#{car.get_year} #{car.get_make} #{car.get_model}"
end
Step by Step Solution
There are 3 Steps involved in it
Step: 1
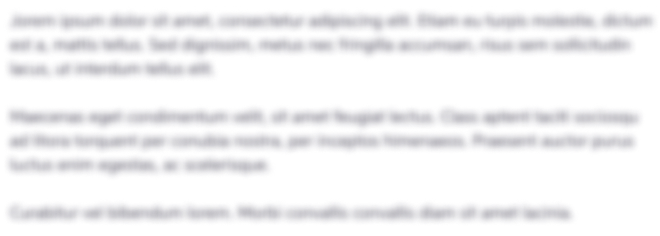
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started