Question
SAMPLE TEXT. A sample text message. is the contents of this message. Examples: Instance: sender.displayName() -> Marina, text -> Deadline is Tuesday. Output:
SAMPLE TEXT.
"A sample text message." is the contents of this message.
Examples:
Instance: sender.displayName() -> "
text -> "Deadline is Tuesday."
Output: "
Instance: sender.displayName() -> "Nabi",
text -> "<(>_<)>"
Output: "Nabi [2019-12-20]: <(>_<)>"
IMPORTANT: The format must be exactly as above including spaces and capitalization for our grader to give you credit.
1.3 PhotoMessage class (extends abstract class)
The PhotoMessage class will also 'extend' the abstract class Message. In addition, this class will also have its own instance variable extension, which is the file extension of the photo.
Key rules for this class:
Only premium users can send PhotoMessage. The constructor will take a string as the source path of the photo. In order to create a valid photo message, this path should be referring to a photo. This path is considered valid if the extension name is one of the following: "jpg", "jpeg", "gif", "png", "tif", "tiff" and "raw". public PhotoMessage(User sender, String photoSource)
throws OperationDeniedException
The constructor must call 'super' and pass in the parameters required by the Message constructor as the FIRST LINE. In this case, it's "super(sender);". This is a requirement of extending an abstract class. You must use the 'super classes' constructor via the keyword 'super'.
Initialize contents with the argument photoSource, and make the file extension extracted from photoSource to lowercase and store it to the variable extension (excluding the dot).
You don't need to check if a file exists at the given path in photoSource.
photoSource will always be in the form "[filename].[extension]".
Examples:
Can be used as photoSource and will not throw error due to having acceptable extensions "Nabi.Yuxuan.jpg", "?????.GiF", "A.E.I.O.U.TIF"
Might be passed in as photoSource and will throw error due to unaccepted extensions "saJ.Md&.^dsas.F123", "d/images/example.pdf", "A.E.I.O.U.TXT"
Will not be passed in as photoSource (Will not use these strings to test) ". ", ".png", ".jpg", "file.", " ", "?????"
EXCEPTIONS
If the sender is not a premium user, you should throw an OperationDeniedException with message DENIED_USER_GROUP (defined in Message abstract class). The keyword instanceof will be helpful to this method (Usage:
public String getExtension()
Method will return the extension of this message.
public String getContents()
Returns a String in the form:
"SenderName [2020-01-15]: Picture at image/example.tif"
Explanation:
"SenderName" is the displayed name of the sender. You can use the getter to access the sender. "2020-01-15" is the date of this message. This part of the string is obtained by calling date.toString(). "image/example.tif" is the contents of this message.
Examples:
Instance: sender.displayName() -> "
photoSource -> "users/Marina/images/IMG_5550.JPG"
Output: "
Instance: sender.displayName() -> "Nabi",
photoSource -> "selfie.gif"
Output: "Nabi [2020-01-21]: Picture at selfie.gif"
IMPORTANT: The format must be exactly as above including spaces and capitalization for our grader to give you credit.
1.4 MessageType class (enum)
We have provided a MessageType enum class to express the message types. An enum class is a special kind of class to declare nominal constants, which is perfect to express the message type. Without using an enum class, we will have to assign arbitrary values to these constants, and this is definitely not a neat solution.
In this class, we defined two constants: TEXT, PHOTO to represent the two message types. To reference these declarations in other classes, just call them like how you would call other class variables (e.g. MessageType.TEXT). The variables declared here will be used (and only used) as the msgType argument of User.sendMessage() (Part 2.1).
Note that this is not a subclass of Message class. You don't need to modify this class, and you don't need to worry about styling this class.
STARTER CODE 1.1
import java.time.LocalDate;
public abstract class Message {
/* Error message to use in OperationDeniedException */ protected static final String DENIED_USER_GROUP = "This operation is disabled in your user group.";
/* instance variables */ private LocalDate date; private User sender; protected String contents;
public Message(User sender) { /* TODO */
this.date = LocalDate.now(); }
public LocalDate getDate() {
. /* TODO */ return null; }
public User getSender() {
/* TODO */ return null; }
public abstract String getContents();
}
STARTER CODE 1.2
public class TextMessage extends Message {
/* Error message to use in OperationDeniedException */ private static final String EXCEED_MAX_LENGTH = "Your input exceeded the maximum length limit.";
public TextMessage(User sender, String text) throws OperationDeniedException { /* TODO */ }
public String getContents() { /* TODO */ return null; }
}
STARTER CODE 1.3
public class PhotoMessage extends Message {
/* Error message to use in OperationDeniedException */ private static final String INVALID_INPUT = "The source path given cannot be parsed as photo.";
/* instance variable */ private String extension;
public PhotoMessage(User sender, String photoSource) throws OperationDeniedException { /* TODO */ }
public String getContents() { /* TODO */ return null; }
public String getExtension() { /* TODO */ return null; }
} STARTER CODE 1.4
/** * Message Types. Use these types as the msgType argument of User.sendMessage(). */ public enum MessageType { TEXT, // MessageType.TEXT
Step by Step Solution
There are 3 Steps involved in it
Step: 1
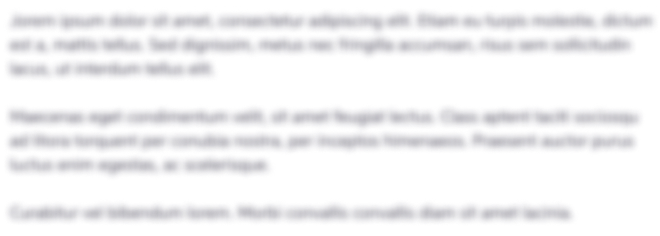
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started