Question
Scenario/Summary [THIS IS C++ LANGUAGE] We have two separate goals this week: We are going to create an abstract Employee class and two pure virtual
Scenario/Summary [THIS IS C++ LANGUAGE]
We have two separate goals this week:
We are going to create an abstract Employee class and two pure virtual functions - calculatePay() and displayEmployee(). The abstract Employee class will prevent a programmer from creating an object based on Employee, however, a pointer can still be created. Objects based on Salaried and Hourly will be allowed. The pure virtual function calculatePay() in Employee will force the child classes to implement calculatePay(). The other pure virtual function displayEmployee() in Employee will force the child classes to implement displayEmployee().
We are going to implement Polymorphism and dynamic binding in this Lab.
STEP 1: Understand the UML Diagram
Notice in the updated UML diagram that the Employee class is designated as abstract by having the class name Employee italicized. Also, the calculatePay method is italicized, which means that it is a pure virtual function and needs to be implemented in the derived classes. In addition, make displayEmployee() method a pure virtual function as well.
STEP 3: Modify the Employee Class
Define calculatePay() as a pure virtual function.
Define displayEmployee() as a pure virtual function.
When class Employee contains two pure virtual functions, it becomes an abstract class.
STEP 4: Create Generalized Input Methods
Reuse method getInput() from the previous Lab to prompt the user to enter Employee information.
STEP 5: Modify the Main Method
Create two employee pointers with:
The first employee pointer refers to a salaried employee and the second employee pointer refers to a hourly employee.
Prompt the user to enter information for these two pointers and display the calculated result.
For salaried employee, the following information needs to be displayed:
Partial Sample Output:
For hourly employee, the following information needs to be displayed:
Partial Sample Output:
PREVIOUS WEEK'S CODE:
Source.cpp
Main
#include
#include
#include
#include "Employee.h" //***REVISED
#include "Salaried.h" //***NEW
#include "Hourly.h" //***NEW
// The Methods
void DisplayApplicationInformation();
void DisplayDivider(string outputTitle);
string GetInput(string inputType);
void TerminateApplication();
//int Employee :: numEmployees = 0;
int main(){ // begin main()
// The Variables
DisplayApplicationInformation();
DisplayDivider("Start Program");
Employee employeeObject1;
// Gathering user input
DisplayDivider(" Employee 1 ");
string firstName = GetInput("First Name: ");
employeeObject1.setFirstName(firstName);
string lastName = GetInput("Last Name: ");
employeeObject1.setLastName(lastName);
string gender = GetInput("Gender: ");
char firstCharacterGender = gender[0]; // Takes value and places into an array - using the first character
employeeObject1.setGender(firstCharacterGender);
string dependents = GetInput("Dependents: ");
employeeObject1.setDependents(dependents); // Using new Setter method
string annualSalary = GetInput("Annual Salary: ");
employeeObject1.setAnnualSalary(annualSalary); // Using new Setter method
string healthInsurance = GetInput("Health Insurance: ");
employeeObject1.getBenefit().setHealthInsurance(healthInsurance);
string lifeInsurance = GetInput("Life Insurance: ");
employeeObject1.getBenefit().setLifeInsurance(stod(lifeInsurance));
string vacation = GetInput("Vacation: ");
employeeObject1.getBenefit().setVacation(stoi(vacation));
// Display Employee Object
employeeObject1.displayEmployee();
cout
DisplayDivider("Number of Employee Object(s) Created");
cout
// ***NEW Salaried Employee Section
DisplayDivider(" Employee 2 ");
Salaried salariedEmployee1;
// Employee 2 [Salaried] Object
firstName = GetInput("First Name: ");
salariedEmployee1.setFirstName(firstName);
lastName = GetInput("Last Name: ");
salariedEmployee1.setLastName(lastName);
gender = GetInput("Gender: ");
firstCharacterGender = gender[0]; // Takes value and places into an array - using the first character
salariedEmployee1.setGender(firstCharacterGender);
dependents = GetInput("Dependents: ");
salariedEmployee1.setDependents(dependents); // Using new Setter method
annualSalary = GetInput("Annual Salary: ");
salariedEmployee1.setAnnualSalary(annualSalary); // Using new Setter method
healthInsurance = GetInput("Health Insurance: ");
salariedEmployee1.getBenefit().setHealthInsurance(healthInsurance);
lifeInsurance = GetInput("Life Insurance: ");
salariedEmployee1.getBenefit().setLifeInsurance(stod(lifeInsurance));
vacation = GetInput("Vacation: ");
salariedEmployee1.getBenefit().setVacation(stoi(vacation));
// Display Salaried Employee Object
salariedEmployee1.displayEmployee();
cout
DisplayDivider("Number of Employee Object(s) Created");
cout
// ***NEW Hourly Employee Section
DisplayDivider(" Employee 3 ");
Hourly hourlyEmployee1;
// Employee 3 [Hourly] Object
firstName = GetInput("First Name: ");
hourlyEmployee1.setFirstName(firstName);
lastName = GetInput("Last Name: ");
hourlyEmployee1.setLastName(lastName);
gender = GetInput("Gender: ");
firstCharacterGender = gender[0]; // Takes value and places into an array - using the first character
hourlyEmployee1.setGender(firstCharacterGender);
dependents = GetInput("Dependents: ");
hourlyEmployee1.setDependents(dependents); // Using new Setter method
annualSalary = GetInput("Annual Salary: ");
hourlyEmployee1.setAnnualSalary(); // Using new Setter method
healthInsurance = GetInput("Health Insurance: ");
hourlyEmployee1.getBenefit().setHealthInsurance(healthInsurance);
lifeInsurance = GetInput("Life Insurance: ");
hourlyEmployee1.getBenefit().setLifeInsurance(stod(lifeInsurance));
vacation = GetInput("Vacation: ");
hourlyEmployee1.getBenefit().setVacation(stoi(vacation));
// Display Hourly Employee Object
hourlyEmployee1.displayEmployee();
cout
DisplayDivider("Number of Employee Object(s) Created");
cout
TerminateApplication();
} // end main()
void DisplayApplicationInformation(){ // begin DisplayApplicationInformation
// Method: Provide the program user some basic information about the program
cout
cout
} // end DisplayApplicationInformation
void DisplayDivider(string outputTitle){ // begin DisplayDivider
// Method: Provide a meaningful output separator between different sections of the program output
cout
cout
} // end DisplayDivider
string GetInput(string inputType){ // begin inputType
// Method: Generalized function that will prompt the user for a specific type of information
string strInput;
cout
getline(cin, strInput);
return strInput;
} // end GetInput
void TerminateApplication(){ // begin TerminateApplication
// Method: Provides a program termination message and then terminate the application
cout
cout
system("pause"); // Keep the output window from automatically closing
} // end TerminateApplication
Hourly.h
Hourly Header
#include "Employee.h"
using namespace std;
#ifndef HOURLY_H
#define HOURLY_H
class Hourly : public Employee{ // begin Hourly class
private:
static const int MIN_WAGE = 10;
static const int MAX_WAGE = 75;
static const int MIN_HOURS = 0;
static const int MAX_HOURS = 50;
double wage;
double hours;
string category;
public:
Hourly();
Hourly(double wge, double hrs, string cat);
Hourly(string first, string last, char gen, int dep, double wge, double hrs, Benefit ben, string cat);
double calculatePay();
void displayEmployee();
double getAnnualSalary();
void setAnnualSalary();
// Getters & Setters
double getWage();
void setWage();
double getHours();
void setHours();
string getCategory();
void setCategory();
}; // end Hourly class
#endif
Hourly.cpp
Hourly Class
#include
#include
#include
#include "Employee.h"
#include "Hourly.h"
Hourly :: Hourly(){ // begin [default] Hourly constructor
// The Variables
wage = 0;
hours = 0;
category = "Unknown";
} // end [default] Hourly constructor
Hourly :: Hourly(double wge, double hrs, string cat){ // begin Hourly constructor [with parameters]
wage = wge;
hours = hrs;
category = cat;
} // end Hourly constructor [with parameters]
Hourly :: Hourly(string first, string last, char gen, int dep, double wge, double hrs, Benefit ben, string cat) : Employee(first, last, gen, dep, ben){ // begin Hourly [loaded] constructor
wage = wge;
hours = hrs;
category = cat;
} // end Hourly [loaded] constructor
double Hourly :: calculatePay(){ // begin Hourly calculatePay method
return wage * hours;
} // end Hourly calculatePay method
void Hourly :: setAnnualSalary(){ // begin Hourly setAnnualSalary method
annualSalary = calculatePay() * 50;
} // end Hourly setAnnualSalary method
void Hourly :: displayEmployee() { // begin Hourly displayEmployee method
Employee :: displayEmployee();
cout
cout
cout
cout
cout
} // end Hourly displayEmployee method
Employee.h
Employee Header
using namespace std;
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
#include "Benefit.h"
class Employee{ // begin Employee class
protected: // ***REVISED to protected
string firstName;
string lastName;
char gender;
int dependents;
double annualSalary;
Benefit benefit;
private:
static int numEmployees;
public:
Employee();
// Employee(string first, string last, char gen, int dep, double salary);
// ***REVISED
Employee(string first, string last, char gen, int dep, Benefit benefit);
double calculatePay();
void displayEmployee();
string getFirstName();
void setFirstName(string first);
string getLastName();
void setLastName(string last);
char getGender();
void setGender(char gen);
int getDependents();
void setDependents(int dep);
void setDependents(string dep);
double getAnnualSalary();
void setAnnualSalary(double salary);
void setAnnualSalary(string salary);
static int getNumEmployees();
// ***REVISED
Benefit getBenefit();
void setBenefit(Benefit ben);
}; // end Employee class
#endif
Employee.cpp
Employee Class
#include
#include
#include
#include "Employee.h"
// ***REMOVED?
//class iEmployee{ // begin iEmployee class
//public:
//virtual double calculatePay() = 0;
//}; // end iEmployee class
Employee :: Employee(){ // begin [default] Employee constructor
// The Constructors
// The Variables
firstName = "not given";
lastName = "not given";
gender = 'U'; // U for unknown
dependents = 0;
annualSalary = 20000;
numEmployees++;
} // end [default] Employee constructor
//Employee::Employee(string first, string last, char gen, int dep, double salary){ // begin Employee constructor [with parameters]
// ***REVISED
Employee::Employee(string first, string last, char gen, int dep, Benefit ben){ // begin Employee constructor [with parameters]
firstName = first;
lastName = last;
gender = gen;
dependents = dep;
numEmployees++;
} // end Employee constructor [with parameters]
double Employee :: calculatePay(){ // begin calculatePay
return annualSalary / 52;
} // end calculatePay
// Display Interface
void Employee :: displayEmployee(){ // begin displayEmployee
cout
cout
cout
cout
cout
cout
cout
// ***REVISED
benefit.displayBenefits();
} // end displayEmployee
// The Getters & Setters
string Employee :: getFirstName(){ // begin getFirstName
return firstName;
} // end getFirstName
void Employee :: setFirstName(string first){ // begin setFirstName
firstName = first;
} // end setFirstName
string Employee :: getLastName(){ // begin getLastName
return lastName;
} // end getLastName
void Employee :: setLastName(string last){ // begin setLastName
lastName = last;
} // end setLastName
char Employee :: getGender(){ // begin getGender
return gender;
} // end getGender
void Employee :: setGender(char gen){ // begin setGender
gender = gen;
} // end setGender
int Employee :: getDependents(){ // begin getDependents
return dependents;
} // end getDependents
void Employee :: setDependents(int dep){ // begin setDependents
dependents = dep;
} // end setDependents
// ** New string dependents
void Employee :: setDependents(string dep){ // begin setDependents
dependents = stoi(dep); // String to integer conversion
} // end setDependents
double Employee :: getAnnualSalary(){ // begin getAnnualSalary
return annualSalary;
} // end getAnnualSalary
void Employee :: setAnnualSalary(double salary){ // begin setAnnualSalary
annualSalary = salary;
} // end setAnnualSalary
void Employee :: setAnnualSalary(string salary){ // begin setAnnualSalary
annualSalary = stod(salary); // String to double conversion
} // end setAnnualSalary
// ***REVISED
Benefit Employee :: getBenefit(){ // begin getBenefit
return benefit;
} // end getBenefit
void Employee :: setBenefit(Benefit ben){ // begin setBenefit
benefit = ben;
} // end setBenefit
// ***REVISED
int Employee :: numEmployees = 0;
int Employee :: getNumEmployees(){ // begin getNumEmployees
return numEmployees;
} // end getNumEmployees
Salaried.h
Salaried Header
#include "Employee.h"
using namespace std;
#ifndef SALARIED_H
#define SALARIED_H
class Salaried : public Employee{ // begin Salaried class
private:
static const int MIN_MANAGEMENT_LEVEL = 0;
static const int MAX_MANAGEMENT_LEVEL = 3;
static const int BONUS_PERCENT = 10;
int managementLevel;
public:
Salaried();
Salaried(string first, string last, char gen, int dep, double sal, Benefit ben, int manLevel);
Salaried(double sal, int manLevel);
double calculatePay();
void displayEmployee();
int getManagementLevel();
void setManagementLevel();
}; // end Salaried class
#endif
Salaried.cpp
Salaried Class
#include
#include
#include
#include "Employee.h"
#include "Salaried.h"
Salaried :: Salaried(){ // begin [default] Salaried constructor
// The Variables
managementLevel = 0;
} // end [default] Salaried constructor
Salaried :: Salaried(string first, string last, char gen, int dep, double sal, Benefit ben, int manLevel) : Employee(first, last, gen, dep, ben){ // begin Salaried [loaded] constructor
managementLevel = manLevel;
annualSalary = sal;
} // end Salaried [loaded] constructor
Salaried :: Salaried(double sal, int manLevel){ // begin Salaried constructor [with parameters]
annualSalary = sal;
managementLevel = manLevel;
} // end Salaried constructor [with parameters]
double Salaried :: calculatePay(){ // begin Salaried calculatePay method
return Employee :: calculatePay() * (1 + (managementLevel * BONUS_PERCENT));
} // end Salaried calculatePay method
void Salaried :: displayEmployee() { // begin Salaried displayEmployee method
Employee :: displayEmployee();
cout
cout
cout
} // end Salaried displayEmployee method
Benefit.h
Benefit Header
using namespace std;
#ifndef BENEFIT_H
#define BENEFIT_H
class Benefit{ // begin Benefit class
private:
string healthInsurance;
double lifeInsurance;
int vacation;
public:
Benefit();
Benefit(string health, double life, int vac);
void displayBenefits();
string getHealthInsurance();
void setHealthInsurance(string health);
double getLifeInsurance();
void setLifeInsurance(double life);
int getVacation();
void setVacation(int vac);
}; // end Benefit class
#endif
Benefit.cpp
Benefit Class
#include
#include
#include
#include "Benefit.h"
Benefit :: Benefit(){ // begin [default] Benefit constructor
// The Constructors
// The Variables
healthInsurance = "Not Given";
lifeInsurance = 0.00;
vacation = 0;
} // end [default] Benefit constructor
Benefit :: Benefit(string health, double life, int vac){ // begin Benefit constructor [with parameters]
healthInsurance = health;
lifeInsurance = life;
vacation = vac;
} // end Benefit constructor [with parameters]
// The Getters & Setters
string Benefit :: getHealthInsurance(){ // begin getHealthInsurance
return healthInsurance;
} // end getHealthInsurance
void Benefit :: setHealthInsurance(string health){ // begin setHealtInsurance
healthInsurance = health;
} // end setFirstName
double Benefit :: getLifeInsurance(){ // begin getLifeInsurance
return lifeInsurance;
} // end getLifeInsurance
void Benefit :: setLifeInsurance(double life){ // begin setLifeInsurance
lifeInsurance = life;
} // end setLifeInsurance
int Benefit :: getVacation(){ // begin getVacation
return vacation;
} // end getVacation
void Benefit :: setVacation(int vac){ // begin setVacation
vacation = vac;
} // end setVacation
// Display Interface
void Benefit :: displayBenefits(){ // begin displayBenefits
cout
cout
cout
cout
cout
} // end displayBenefits
Benefit Emplovee -healthinsurance string -lifeinsurance double vacation int +Benefit(0 +Benefit(in hins: string, in lins double, in vac: int) +displayBenefits) void #firstName . String #lastName : string #gender: char #dependents int #annua!Salary : double #benefitBenefit static numEm +Employee) +Employee(in fname: string, in Iname string, in gen: char, in dep: int, in benefits: Benefit) +static getNumEmployees0: int +CalculatePay): double +displayEmployee void ees : int = 0 Salaried -MIN MANAGEMENT LEVEL: int=0 -MAX MANAGEMENT LEVEL: int=3 BONUS PERCENT : double= 10 -managementLevel int +Salariedo +Salaried(in fname string, in Iname string, in gen: char, in dep int, in sal double, in ben Benefit, in manLevel: int) +Salaried(in sal: double, in manLevel: int) +CalculatePay double +dis void Hourl -MIN WAGE: double= 10 -MAX WAGE : double = 75 -MIN HOURS: double=0 MAX HOURS: double 50 wage: double -hours: double -cate +Hourly) +Hourly (in wage: double, in hours double, in category: string) +Hourly in fname string, in Iname : string, in gen: char, in dep: int, in wage: double, in hours: double, in ben: Benefit, in category string) +CalculatePay double +displayEm strin ee() void Benefit Emplovee -healthinsurance string -lifeinsurance double vacation int +Benefit(0 +Benefit(in hins: string, in lins double, in vac: int) +displayBenefits) void #firstName . String #lastName : string #gender: char #dependents int #annua!Salary : double #benefitBenefit static numEm +Employee) +Employee(in fname: string, in Iname string, in gen: char, in dep: int, in benefits: Benefit) +static getNumEmployees0: int +CalculatePay): double +displayEmployee void ees : int = 0 Salaried -MIN MANAGEMENT LEVEL: int=0 -MAX MANAGEMENT LEVEL: int=3 BONUS PERCENT : double= 10 -managementLevel int +Salariedo +Salaried(in fname string, in Iname string, in gen: char, in dep int, in sal double, in ben Benefit, in manLevel: int) +Salaried(in sal: double, in manLevel: int) +CalculatePay double +dis void Hourl -MIN WAGE: double= 10 -MAX WAGE : double = 75 -MIN HOURS: double=0 MAX HOURS: double 50 wage: double -hours: double -cate +Hourly) +Hourly (in wage: double, in hours double, in category: string) +Hourly in fname string, in Iname : string, in gen: char, in dep: int, in wage: double, in hours: double, in ben: Benefit, in category string) +CalculatePay double +displayEm strin ee() voidStep by Step Solution
There are 3 Steps involved in it
Step: 1
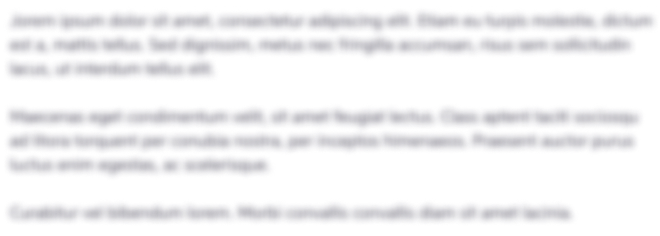
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started