Searching - a user should be able to search by ID or by item name create only one menu option for searching (no sub-menu) a
- Searching - a user should be able to search by ID or by item name
- create only one menu option for searching (no sub-menu)
- a user should be able to enter the name of the item using any case, (for example, sTRolleR)
- store items in all lower/upper case to expedite the search
- write one search function that can search by ID and name and goes through the array of structs/objects only once
- allow partial search
- must return all matching items (for example, a user can enter \"roll\" to search for a \"stroller\"; return \"rolls\", \"stroller\")
- see C++ find() function
- show the entire record if found or an appropriate message if not
#include #include #include #include
using namespace std;
const int MAX = 100;
class Records {
private:
string car_ID, car_model; int quantity; double price;
public:
// SET FUNCTIONS void setCarID(string item_ID) { this->car_ID = item_ID; }
void setQuantity(int quantity) { this->quantity = quantity; }
void setCarModel(string item_name) {
this->car_model = item_name;
}
void setPrice(double price) { this->price = price; }
//GET FUNCTIONS string getCarID() { return car_ID; }
int getQuantity() { return quantity; }
string getCarModel() { return car_model; }
double getPrice() { return price; }
};
void readRecords(Records Inventory[], int& count); void printRecords(Records Inventory[], int& count); void searchID(Records Inventory[], int& count); void searchName(Records Inventory[], int& count); void bubbleSort(Records Inventory[], int& count, int sortOption);
int main(int argc, const char* argv[]) {
Records Inventory[100];
int n = 0;
readRecords(Inventory, n);
int choice; int choice1;
do { cout cout cout cout cout cout cout cout cout cout
cin >> choice; cout
switch (choice) {
case 1:
cout printRecords(Inventory, n); cout break;
case 2:
searchID(Inventory, n); cout break;
case 3:
searchName(Inventory, n); cout break;
case 4: do { cout cout cin >> choice1; cout if (choice1 >= 0 && choice1 { bubbleSort(Inventory, n, choice1); } else cout } while (choice1 3); printRecords(Inventory, n); break;
case 5: cout break;
default: cout }
} while (choice != 5);
return 0;
}
void readRecords(Records Inventory[], int& count)
{
ifstream myFile(\"input.txt\");
string car_ID, car_model; int quantity; double price;
if (!myFile) {
cout exit(EXIT_FAILURE);
}
while (!myFile.eof())
{
myFile >> car_ID >> car_model >> quantity >> price;
Inventory[count].setCarID(car_ID); Inventory[count].setCarModel(car_model); Inventory[count].setQuantity(quantity); Inventory[count].setPrice(price); count++;
}
myFile.close(); }
void printRecords(Records Inventory[], int& count)
{
for (int i = 0; i
{
cout
}
}
void searchID(Records Inventory[], int& count) {
string item; cout cin >> item; cout
for (int i = 0; i
if (Inventory[i].getCarID() == item) { cout cout return; }
cout
}
void searchName(Records Inventory[], int& count) {
string name; cout cin >> name; cout
name[0] = toupper(name[0]);
for (int i = 0; i
if (Inventory[i].getCarModel() == name)
{ cout cout return; }
cout
}
//Add sort Function void bubbleSort(Records Inventory[], int& count, int sortOption) { cout Records tmp; for (int i = 0; i { for (int j = 0; j { switch (sortOption) { case 0: //sort by item Id if (Inventory[j].getCarID().compare(Inventory[j + 1].getCarID()) >0) { tmp = Inventory[j]; Inventory[j] = Inventory[j + 1]; Inventory[j + 1] = tmp; } break; case 1: //sort by item name if (Inventory[j].getCarModel().compare(Inventory[j + 1].getCarModel()) > 0) { tmp = Inventory[j]; Inventory[j] = Inventory[j + 1]; Inventory[j + 1] = tmp; } break; case 2: //sort by item Id if (Inventory[j].getQuantity() > Inventory[j + 1].getQuantity()) { tmp = Inventory[j]; Inventory[j] = Inventory[j + 1]; Inventory[j + 1] = tmp; } break; case 3: //sort by item Id if (Inventory[j].getPrice() > Inventory[j + 1].getPrice()) { tmp = Inventory[j]; Inventory[j] = Inventory[j + 1]; Inventory[j + 1] = tmp; } break; default: cout } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
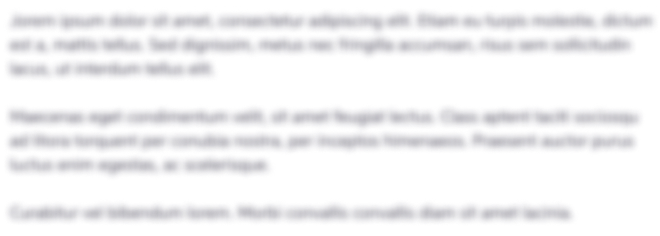
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started