Question
Seeking help on how to add the needed conditions, in Python, attached is the code I am currently working with, just need clarification as to
Seeking help on how to add the needed conditions, in Python, attached is the code I am currently working with, just need clarification as to where to add the changes needed thank you.
Assignment: Arrays Buffers with and Without Duplicates. The ArrayBuffer class in Assignment 1 had methods for insert, find, remove, removeStable, and display, as well as a private helper function called locationOf. In this assignment, we will create two variants of the ArrayBuffer class; ArrayBufferNoDups and ArrayBufferWithDups as children classes of the ArrayBuffer class. As the name implies, the first class does not allow the insertion of duplicate values, while the second one does. The ArrayBufferNoDups class should look like the ArrayBuffer class in Assignment 1. Only the insert operation has to change. Both remove functions should assume that there is never more than one copy of a value. For this assignment I would also like the distinction between the two remove functions to be clearer. So please rename them fastRemove and stableRemove. The ArrayBufferWithDups class should also look like the class in ArrayBuffer class in Assignment 1. This time, do not change the insert function, and implement both remove functions as for the NoDups case. But, in addition, add three new methods, called findAll, fastRemoveAll, and stableRemoveAll. The findAll function should return an int with the number of elements that have the same value as the target value, while the two removeAll functions should remove all copies of the target value. Have both removeAll functions return an int with the number of values that were actually removed. For this assignment, we will make two more changes. First, omit the BUFFER_SZ constant, and instead have the constructor take an integer argument for the size.
Below is my portion of the code in which I am attempting to insert what's asked above
class BufferArray: def __init__(self, numberofelement, listofelements): self.listofelements = listofelements self.numberofelement = numberofelement self.buffer_size = 20 def insert(self, value): if len(self.listofelements) >= 20: return False self.listofelements.append(value) self.numberofelement =+ self.numberofelement return True def remove(self, value): temp = self.listofelements.index(value) if temp: del self.listofelements[temp] self.numberofelement -= 1 return True else: return False def find(self, target): if self.listofelements.count(target) > 0: return True else: return False def locationof(self, target): if self.listofelements.count(target) < 0: return -1 return self.listofelements.index(target) def display(self): if len(self.listofelements) == 0: print(' ') return for i in self.listofelements: print(i, end = ',') print(" ") bufferArray = BufferArray(5,[4,1,6,3,2]) bufferArray.insert(9) bufferArray.display() bufferArray.remove(6) #subsitution for stable remove bufferArray.display() print(bufferArray.find(9)) print(bufferArray.locationof(9))
Step by Step Solution
There are 3 Steps involved in it
Step: 1
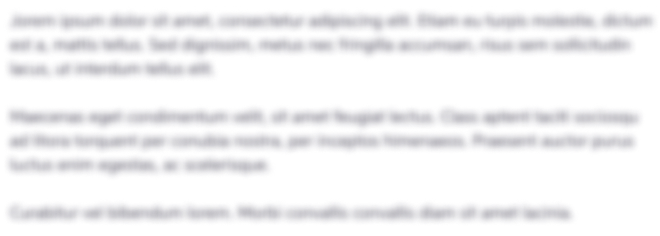
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started