Question
Sentence Histograms For this assignment, you will be furnished with the skeleton structure of a Swing GUI application for displaying letter occurrence histograms for sentences
Sentence Histograms
For this assignment, you will be furnished with the skeleton structure of a Swing GUI application for displaying letter occurrence histograms for sentences in a selected text file. The skeleton code is in two files that you can download from the links in the Project Setup section below. These files contain empty methods for four methods, which you will need to develop. It will be your task to develop the event handlers and histogram display methods, plus any support utility methods you need to make the program operate as described below.
Program Operation
When the program is launched from the command line, the following GUI will appear:
When the "Load File" button is pressed, a file chooser should appear. You must develop the code to implement this feature.
When a properly formatted input file is selected and opened, the sentences in the file will be numbered and displayed in the left panel, as shown here. You must develop the code to implement this feature.
When the "Show Histo for Sentence" button is pressed, or when the cursor is in the textfield and the Enter key is pressed, the program should attempt to parse an integer from the text field. If there is no value or the value cannot be parsed as an integer, the program should present the JOptionPane message shown here. You must develop the code to implement this feature.
If the value in the text field can be parsed as an integer, but the integer value is less than zero or greater than the greatest sentence index, the program presents the JOptionPane message shown here. This is functionality that is already provided by the skeleton code, so you do not need to implement this feature.
However, if the value can be parsed as an integer within range, then the program should compute the letter occurrence histogram for the sentence and display it graphically in the right pane, as shown here. You must develop the code to implement this feature.
The histogram display methods should be developed so that when the GUI window is resized, the histogram display automatically refreshes and resizes to fit within the available space, as shown below. You must develop the code to implement this feature.
Input File Format
The input file format is the same as for Program 2. For details please see that assignment. The sample data file for the output shown above is:
Specific Requirements
A. Your name must appear in the title bar of the GUI window. This should be done in the Histogram class constructor.
B. You must write the code for the Histogram class's loadButtonActionPerformed( ActionEvent evt ) method. This event handler must take the following actions when the "Load File" button is pressed:
1. Launch a JFileChooser dialog 2. The file chooser must open to the current user folder, as shown in lecture 3. Do not use a file filter with the file chooser 4. If the selected file is not null: a. Set the text of the sourceArea (a GUI variable in the Histogram class) to the String that is returned from outPanel's readFile method using the selected file as input (outPanel is also a GUI variable in the Histogram class). b. Enable the numField text field GUI variable c. Set the text in numField to an empty string d. enable the showButton GUI variable 5. But if the selected file returned from the file chooser is null, do nothing
6. You should be aware that JComponents such as JButtons and JTextfields have the instance method setEnabled(boolean b), such that if b is true, then the component is enabled (i.e., active), but if b is false, then the component is grayed out (i.e., inactive).
7. You should also know that JTextfields also have instance method getText() to retrieve the string in the text field, and instance method setText( String s ) to sets the string in the text field to the string argument.
C. You must write the code for the Histogram class's showButtonActionPerformed( ActionEvent evt ) method. This event handler must take the following actions when the "Show Histo for Sentence" button is pressed:
1. Retrieve the String value that is in the numField textField.
2. If the value retrieved cannot be parsed as an integer, display a JOptionPane message dialog stating, "Text field is not an integer".
3. If the value retrieved can be parsed as an integer, the program should invoke outPanel's showHisto method using the parsed integer as the first argument and "true" (without the quotation marks) as the second argument. You do not need to test whether the integer is within the proper range, since the skeleton code that is provided to you already knows how to handle out of range value by popping up the out-of-range JOptionPane message, so you do not need to program this.
If the integer value is within range and your code for the HistogramPanel class is implemented properly, a letter histogram for the selected sentence will display in the right-hand panel of the GUI with 10% margins on all sides, as shown here:
D. You must write the code for the HistogramPanel class's drawLines( Graphics gc ) method. This method must:
1. Draw the x- and y- coordinate axis lines for the histogram as shown in figure above. Please note that the x-axis line is the red line that appears at the bottom of all the histogram bars and the y-axis line is the red line that appears next to the leftmost histogram bar. The axis lines are not the red lines shown above that surround the entire image.
2. Do not hard code any fixed values for the size of the output panel outPanel on which you will draw the axes. Instead, use this.getHeight() and this.getWidth() to obtain the current height and width of outPanel. This will allow your axes to adjust automatically when the window is resized.
E. You must write the code for the HistogramPanel class's drawHisto( Graphics gc ) method. This method must:
1. Draw blue rectangle histogram bars for the letter counts 2. The height of each bar must be in proportion to the count it represents 3. The tallest bar will be for the largest letter count value 4. The height of the tallest bar should be equal to: 80% of this.getHeight() 5. The width of each histogram bar should be equal to: (1/26) * ( 80% of this.getWidth()) 6. Dont worry about zero-height rectangles (they will display correctly)
Eclipse Project Setup
These instructions set up the skeleton program in Eclipses. It should run but do nothing other than show the initial GUI. You must extend the skeleton code as described above to achieve the required functionality.
1. Create a Java application project called Histogram"in Eclipse.
2. Create a Java package called histogram"in this project
3. Create a regular Java class file named HistogramPanel.java"in this package
4. Delete the entire contents of the HistogramPanel.java file in your NetBeans project and replacethem with the contents of the HistogramPanel.java file that you can download here. Be sure to edit the course file header to include your name (or names, if you are working as a team).
5. Now, also create a Histogram.java file in the project
6. Delete its entire contents and replace it with the contents of the Histogram.java file that you can download here. Be sure to edit the course file header to include your name (or names, if you are working as a team).
Programming Tips
1. Use the BarChart and BarChartPanel classes from lecture as a starting point. These classes create and display fixed size histograms, so you will need to extend the logic to handle resizing of the display window.
2. In your HistogramPanel class, scale the heights and widths of lines and rectangles using this.getHeight() and this.getWidth(). Once you have these values, it is relatively simple algebra to scale the lines and rectangle.
3. Don't try to do everything inside the drawLines() method. It will be easier to debug if you put self-contained functionality in separate support methods. See also the Bar Chart code for examples of how to do this. The following support methods are suggested:
int[] rawHisto( String s) -- method to compute letter counts for a string representing an entire sentence
int findMax( int[] inp ) -- method to find the max count in an array of letter counts
int[] scaledHisto( int[] inp ) -- method to scale all the histogram count values so that each countis replaced by the number of pixelsfor the height of the bar that represents that count; this method uses the result of findMax above to scale each bar so that the bar with the highest count is 0.8 * this.getHeight()tall; the heights of the other bars should be in proportion to the ratio of their counts to the highest count
Program Testing
A. Make sure your program runs correctly when you run it within Eclipse
B. Make sure the required course headerwith your name(s) is on both source files
C. Export an executable JAR file to a test folder on your desktop in the same manner as for Programs 1 and 2. It is not necessary to use a custom manifest file since we are not using an external library file, but it is OK if you do.
D. Add the cats.txt file to the test folder
E. Also add to the folder any other text files on which you wish to test your program
F. Open a command window and navigate to your test folder
G. Now, test your program using the command: javajar Histogram.jar , Your program should run and perform as required. If it does not, fix the problem and keep testing until you meet with success.
H. Be sure to submit the correct JAR file. You can verify that it contains the .java source files by entering: jar tvf Histogram.jar
What to Submit
For this assignment, you must submit an executable JAR file that contains your Java source files (".java" extension) as well as the bytecode files (".class") for running the program.
Both of your Java source files must contain the source file header for this course:
You should test your JAR file from the command line as described above before submitting it, as also discussed in lecture and in the Programming Resources section of this Webcourse.
Grading
Your program will be graded according to the grading rubric below. Additional penalties will be applied as necessary for:
late submissions
failure to include the source files
failure to include proper source file headers
failure for one teammate to submit
teaming submission errors
The penalties for the above items are described in the course Syllabus.
COP3330 Sentence Histograms byStep by Step Solution
There are 3 Steps involved in it
Step: 1
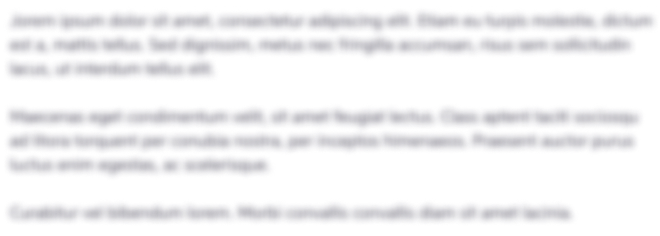
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started