Question
Setting up the project ( 2 points ) Create a new Java project called Module01Test_1410 . Add a package called m01 and inside this package,
Setting up the project ( 2 points )
Create a new Java project called Module01Test_1410.
Add a package called m01and inside this package, a class Module01 that includes a main method. The main method is for your own testing in addition to the JUnit test requirements. Inside class Module01, you will declare two public static methods: challenge1 and challenge2. More details are provided below.
In addition, create a second source folder called tests and add the class Module01Test which includes the jUnit tests for both methods.
Style | Doc Comments (4 points)
Follow the style guide and best practices described in Guidelines and Expectations.
Pay special attention to the sections about doc comments and white space (indentation, grouping). All public methods and classes should have doc comments, including the JUnit Test class. However, there is no need to add doc comments for JUnit test methods (methods preceded by @Test).
Method challenge1 (13 points)
Create a public static method challenge1 that has one parameter str of type String and returns a String. It should create and return a new string based on the parameter str and the specifications listed below. (Hint: no recursion needed)
Use appropriate methods from class String and/or StringBuilder to implement the method challenge1. Here are the specifications:
Replaces all dashes (-) with the hash-sign (#) (4 out of 13 points)
Reverses the order of the characters in the string. (4 out of 13 points)
Adds an exclamation mark at the end of the string if it is more than 4 characters long. (4 out of 13 points)
The empty string should return the empty string. (1 out of 13 points)
Examples: The double quotes indicate that the method returns a string, but they should not be included as characters in the string.
challenge1("") returns "" challenge1("-") returns "#" challenge1("abcd") returns "dcba" challenge1("-xx-") returns "#xx#" challenge1("a!b!c") returns "c!b!a!" challenge1("-a --b ---c") returns "c### b## a#!"
Important: the method needs to work with any string passed as an argument. Tip: You'll be asked to write at least 3 JUnit tests based on the examples provided above. Test your method with all the various input strings from the examples, even if you use the main method for some of the testing.
JUnit Tests for challenge1: (7 points)
Write at least three JUnit test methods to test the method challenge1 based on the input data provided in the examples. Each JUnit test method should have a descriptive name. (1 out of 7 points) Tip: Start the name of the JUnit test method with the name of the method you are testing followed by an underscore and a description of the type/category of input you are testing.
Method challenge2 (16 points)
Create a public static method challenge2 that has one parameter called wordList of type ArrayList
Use appropriate methods from class String and/or StringBuilder to implement the method challenge2. Here are the specifications:
Creates a new output string by combining the first two characters of each word (4 out of 16 points) followed by an period (see output) (2 out of 16 points)
Doesn't add tiny words that are less than 2 characters long to the output string (4 out of 16 points)
Counts the number of tiny words and adds that count to the end of the (4 out of 16 points) output string surrounded by brackets.
If the list is empty, an empty string should be returned. (2 out of 16 points)
Examples: The double quotes indicate that the method returns a string, but they should not be included as characters in the string. Note that I use the same array name for each example. If you want to run the examples in your main method, keep in mind that we can declare a variable with a given name only once.
wordList includes one string: "ant" challenge2(wordList) returns "an.(0)" // no tiny word => count is 0
wordList includes one string: "a" challenge2(wordList) returns "(1)" // one tiny word => count is 1
wordList includes 5 strings: "a", "abc", "a", "abc", "a" challenge2(wordList) returns "ab.ab.(3)" // 3 tiny words => count is 3
wordList includes 3 strings: "ant", "bee", "cat" challenge2(wordList) returns "an.be.ca.(0)"
wordList includes 5 strings: "Ant", "a", "I", "BEAR", "camel" challenge2(wordList) returns "An.BE.ca.(2)"
wordList includes no strings (it is empty) challenge2(wordList) returns ""
Important: the method needs to work with any integer array passed as an argument. Tip: You'll be asked to write at least 3 JUnit tests based on the examples provided above. Test your method with all the various input strings from the examples, even if you use the main method for some of the testing.
JUnit Tests for challenge2: (7 points)
Write at least three JUnit test methods to test the method challenge2 based on the input data provided in the examples. Each JUnit test method should have a descriptive name. (1 out of 7 points) Tip: Start the name of the JUnit test method with the name of the method you are testing followed by an underscore and a description of the type/category of input you are testing.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
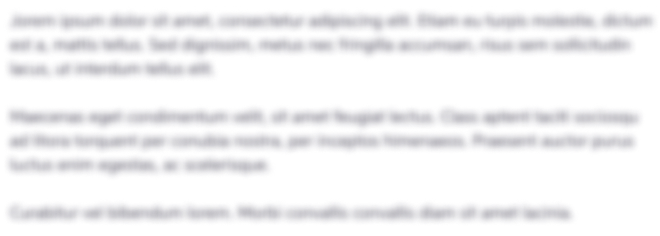
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started