Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Showroom The Showroom class is a bit more sophisticated. Its purpose is to store a collection of Vehicle objects. Each Showroom that you create could
Showroom
The Showroom class is a bit more sophisticated. Its purpose is to store a collection of Vehicle objects.
Each Showroom that you create could have a different number of Vehicles depending on its size so for
this assignment well use a vector. Your Showroom should contain variables for the following:
The name of the Showroom
A vector to store Vehicle objects
A maximum capacity of the showroom we dont want to add Vehicles beyond this limit
In addition, you should create the following functions:
Default constructor all parameters have default values
Showroomstring name "Unnamed Showroom' unsigned int capacity ;
Accessor
vector GetVehicleList;
Behaviors
void AddVehicleVehicle v;
void Showinventory;
float GetinventoryValue;
Function Reference
Constructor Same as all constructors! Initialize class member variables
GetVehicleList Return the vector objects, so other code can access it
AddVehicle If the Showroom is already full numberOfVehicles capacity then you should
print out "Showroom is full! Cannot add Mazda Miata" this will use
the GetYearMakeModel function from the Vehicle class
If there is space in the showroom, add the passin Vehicle to the class vector.
Vector objects can be expanded with the pushback function.
ShowInventory Show all vehicles in the showroom, using the Display function of each vehicle
GetInventoryValue Sum up the prices of all vehicles in the showroom and return that value
#include "Vehicle.h
#include "Showroom.h
#include
#include
using namespace std;
int main
Initialize some data. It's hardcoded here, but this data could come from a file, database, etc
Vehicle vehicles
VehicleFord "Mustang",
VehicleMazdaCX
VehicleDodge "Charger",
VehicleTesla "Model S
VehicleToyota "Prius",
VehicleNissan "Leaf",
VehicleChevrolet "Volt",
;
Set the precision for showing prices with decimal places
cout std::fixed std::setprecision;
int testNum;
cin testNum;
if testNum
Showroom testShowroom;
testShowroom.ShowInventory;
else if testNum
Showroom oneSmall Showroom", ;
one.AddVehiclevehicles;
one.AddVehiclevehicles;
one.ShowInventory;
else if testNum
Showroom oneFull Showroom", ;
one.AddVehiclevehicles;
one.AddVehiclevehicles;
one.AddVehiclevehicles;
one.ShowInventory;
else if testNum
Showroom onePrice Test", ;
one.AddVehiclevehicles;
one.AddVehiclevehicles;
one.AddVehiclevehicles;
cout "Total value: $ one.GetInventoryValue;
else if testNum
Showroom oneRoom ;
one.AddVehiclevehicles;
one.AddVehiclevehicles;
one.AddVehiclevehicles;
cout "Total value: $ one.GetInventoryValue endl;
Showroom twoRoom ;
two.AddVehiclevehicles;
two.AddVehiclevehicles;
two.AddVehiclevehicles;
two.AddVehiclevehicles;
two.AddVehiclevehicles;
two.AddVehiclevehicles;
cout "Total value: $ two.GetInventoryValue;
return ;
#include
#include
using namespace std;
class Vehicle
private:
std::string make;
std::string model;
unsigned int year;
float price;
unsigned int mileage;
public:
Vehicle
make "COP;
model "Rust Bucket";
year ;
price ;
mileage ;
Vehiclestring make, string model, int year, float price, int mileage
thismake make;
thismodel model;
thisyear year;
thisprice price;
thismileage mileage;
void Display
cout year make model $ price mileage endl;
string GetYearMakeModel
string str tostringyear make model;
return str;
float GetPrice
return price;
;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
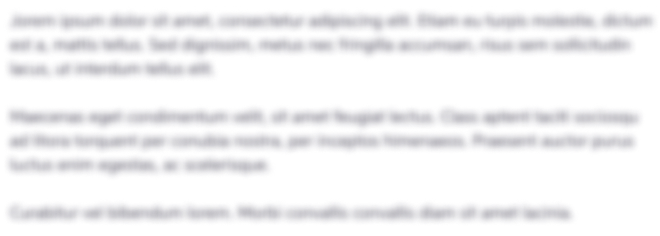
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started