Question
Simple JAVA program, Please fill in the bolded italicized part. import java.util.Calendar ; /** RaceHorseTester -- to test the RaceHorse class in which you have
Simple JAVA program, Please fill in the bolded italicized part.
import java.util.Calendar ;
/**
RaceHorseTester -- to test the RaceHorse class in which you have to write
a default constructor, a constructor with parameters and a toString method.
Change nothing here.
*/
public class RaceHorseTester
{
public static void main(String[] args)
{
RaceHorse horse ;
horse = new RaceHorse() ;
System.out.println(horse) ;
java.util.Calendar rightNow = java.util.Calendar.getInstance() ;
int year = rightNow.get(java.util.Calendar.YEAR) ;
System.out.println("RaceHorse[name = Horse, year = "
+ year + "][trainer = tbd, wins = 0, places = 0, losses = 0] WAS EXPECTED") ;
horse = new RaceHorse("Blaze", 2009, "Charles Chase") ;
horse.record(2) ;
horse.record(0) ;
horse.record(0) ;
horse.record(2) ;
horse.record(2) ;
horse.record(1) ;
System.out.println(horse) ;
System.out.println("RaceHorse[name = Blaze, year = 2009][trainer = Charles Chase, wins = 1, places = 3, losses = 2] WAS EXPECTED") ;
}
}
/**
A Horse object has a name and a year of birth.
The day of birth is always January 1.
There are two todo regions ... one for the default constructor and
one for the toString method.
Note: a nice way to get the current year is:
java.util.Calendar calendar = java.util.Calendar.getInstance() ;
year = calendar.get(java.util.Calendar.YEAR) ;
or if you import java.util.Calendar:
Calendar calendar = Calendar.getInstance() ;
year = calendar.get(Calendar.YEAR) ;
Similarly for the current month.
*/
class Horse
{
private String name ;
private int year ;
/**
Constructs a Horse object with default name and year
*/
public Horse()
{
name = "Horse";
java.util.Calendar calendar = java.util.Calendar.getInstance() ;
year = calendar.get(java.util.Calendar.YEAR) ;
}
/**
Constructs a Horse object with given name and year of birth
@param name1 the given name
@param year the year of birth
*/
public Horse(String name1, int year)
{
name = name1 ;
this.year = year ;
}
/**
Produces a string representation of the object
Note: use getClass().getName() rather than "Horse", so that the
subclass name will be correct.
@return a string representation
*/
public String toString()
{
//-----------Start below here. To do: approximate lines of code = 1
// 1. something like: "Horse[name = Lucky Star, year = 2014]"
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
}
/**
A RaceHorse is a specialized horse that has a trainer.
A Horse object has a name and a year of birth.
The day of birth is always January 1.
Find the todo regions below.
*/
//-----------Start below here. To do: approximate lines of code = 1
// 2. class ... so that RaceHorse is a subclass of Horse
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
{
private String trainer ;
private int wins ;
private int places ;
private int losses ;
/**
Constructs a RaceHorse object with default name and year and trainer
The default trainer is "tbd".
The wins, places, and losses start at zero.
*/
public RaceHorse()
{
super() ;
trainer = "tbd" ;
wins = places = losses = 0 ;
}
/**
Constructs a RaceHorse object with given name, year of birth and trainer
and sets the wins places and losses to zero.
@param name1 the given name
@param year the year of birth
@param trainer the name of the trainer
*/
public RaceHorse(String name1, int year, String trainer)
{
//-----------Start below here. To do: approximate lines of code = 2
// 3. super call
//4. trainer
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
wins = places = losses = 0 ;
}
/**
Records result of a race, incrementing one of wins, places, losses
@param code the result of the race: 1 = win, 2 = place, 0 = loss
*/
public void record(int code)
{
if (code == 0)
losses ++ ;
else if (code == 1)
wins ++ ;
else
places ++ ;
}
/**
Produces a string representation of the object
@return a string representation
*/
public String toString()
{
//-----------Start below here. To do: approximate lines of code = 2
// 5-6. something like: "RaceHorse[name = Lucky Star, year = 2011][trainer = Billy Wonder, wins = 1, places = 2, losses = 10]" and use super call
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
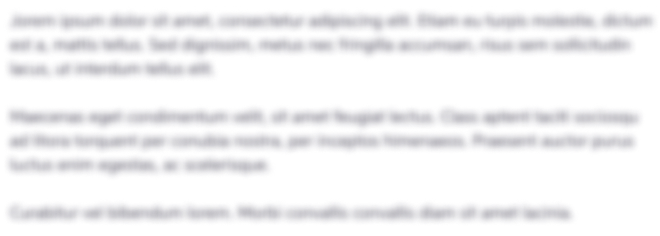
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started