Question
Simulate two CPU scheduling algorithms. FCFS [Code template provided and explained in the class] SJF [Make necessary changes to FCFS to implement this] You must
Simulate two CPU scheduling algorithms. FCFS [Code template provided and explained in the class] SJF [Make necessary changes to FCFS to implement this] You must use the template provided in the class. To understand how to meet the requirements stated below. Required outputs for each algorithm: During the simulation (run for 100 time units): If any process is running, print the process id and global time at each timestamp. If no process is running, print the global time and mention that no process is ready. At the end of the simulation: Print waiting time (WT), completion time (CT) and turnaround time (TAT) for each process. Print average waiting time and average turnaround time. For this task, you can implement additional variables/methods in any class. Prepare a text file (output.txt) with your output lines. You can do this by simply copying the console output to a text file. Otherwise, you can use Java File IO to do it. Follow this link for reference: Java
Create and Write To Files. Submit the text file along with your source codes.
You must use this template:
package schedulingos; import java.util.Comparator; import java.util.PriorityQueue; public class FCFS{ static PriorityQueue
package schedulingos; public class GlobalTimer { int time; public GlobalTimer(int initialTime){ this.time=initialTime; } }
package schedulingos; public class Process { int id; int arrivalTime; int duration; GlobalTimer globalTimer; public Process(int id,int arrivalTime, int duration, GlobalTimer globalTimer){ this.id=id; this.arrivalTime=arrivalTime; this.duration=duration; this.globalTimer=globalTimer; } public void runProcess(){ // TASK: Write your code here // The process will print it's id and global time for the duration specified by burst time } public int getId() { return id; } public int getArrivalTime() { return arrivalTime; } public int getDuration() { return duration; } public GlobalTimer getGlobalTimer() { return globalTimer; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
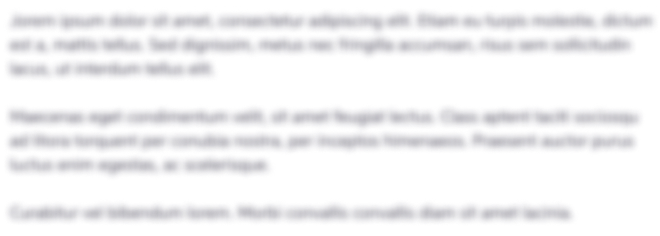
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started