Question
So I have gotten every part of this homework to work except for the copyFloor() function. I did the select floor a little differently and
So I have gotten every part of this homework to work except for the copyFloor() function. I did the select floor a little differently and integrated that option into all the other options. I have code at the top of my driver class showing that my deep copying functions for the Student and Floor classes work but when trying to implement the same logic into my actual driver class it doesn't copy the floor over.
I've played around with it but haven't gotten anything. Thank you for your help.
Student Class:
public class Student implements Cloneable {
final int MAX_WRITEUPS = 3;
private String name;
private int idNumber;
private int numWriteups;
public String getName() {
return name;
}
/**
* Student constructor that takes all three fields so the user can declare and instantiate a
* variable of type Student() at the same time using syntax:
* Student student = new Student(String name, int idNumber, int numWriteups);
*/
public Student(String name, int idNumber, int numWriteups) {
this.name = name;
this.idNumber = idNumber;
this.numWriteups = numWriteups;
}
/**
* Empty Student constructor so user can enter a new student with an empty student variable
* and set their attributes -name,idNumber, and numWriteups- after the variable declaration.
* */
public Student() {
}
public void setName(String name) {
this.name = name;
}
public int getIdNumber() {
return idNumber;
}
public void setIdNumber(int idNumber) {
this.idNumber = idNumber;
}
public int getNumWriteups() {
return numWriteups;
}
public void setNumWriteups(int numWriteups) {
this.numWriteups = numWriteups;
}
public int getMAX_WRITEUPS() {
return MAX_WRITEUPS;
}
@Override
public Student clone() {
Student clone = new Student();
clone.setName(this.getName());
clone.setIdNumber(this.getIdNumber());
clone.setNumWriteups(this.getNumWriteups());
return clone;
}
@Override
public String toString() {
return "Student: " + this.getName() + " has ID number: "
+ this.getIdNumber() + " and " + this.getNumWriteups()
+ " write-ups";
}
}
Floor Class:
/eed to write the error messages and then send the program back to menu for every exception
/eed to write the javadoc for every class and every method
/eed to ask tomorrow about the selectFloor function
public class Floor{
private Student[] students;
final int CAPACITY = 50;
int numFloor = 0;
public Floor(){
students = new Student[CAPACITY];
}
public void addStudent(Student student, int position) throws FullFloorException, IllegalArgumentException{
if(numFloor > 50){
throw new FullFloorException("Cannot add students to full floor.");
}
if(position >= numFloor+1){
throw new IllegalArgumentException("No gaps between rooms allowed.");
}
numFloor++;
if(students[position] != null){
for(int i = numFloor+1; i >= position; i--){
students[i + 1] = students[i];
}
}
students[position] = student;
}
public Student removeStudent(int position) throws IllegalArgumentException, EmptyFloorException{
if(numFloor > 1){
for(int i = position; i
students[i] = students[i+1];
}
}
if (numFloor == 1){
students[position] = null;
}
if(numFloor == 0){
throw new EmptyFloorException("Cannot Remove Student from Empty Floor.");
}
if(position
throw new IllegalArgumentException("Cannot Remove Student from Negative Position.");
}
if(position == numFloor-1){
students[position] = null;
}
if(position > numFloor-1){
throw new IllegalArgumentException("There is no Student in this position on the floor.");
}
numFloor--;
return students[position];
}
public Student getStudent(int position) throws IllegalArgumentException{
if(students[position] == null){
throw new IllegalArgumentException("There is no Student in this position on the floor.");
}
else{
return students[position];
}
}
public void setStudent(Student student, int position) throws IllegalArgumentException{
if(position
throw new IllegalArgumentException("There are no negative positions.");
}
else if(position > numFloor){
throw new IllegalArgumentException("Cannot have gaps between students on a floor.");
}
else{
students[position] = student;
}
}
public int count(){
return numFloor;
}
@Override
public Floor clone(){
Floor clone = new Floor();
for(int i = 0; i
try {
Student cloneStud = students[i].clone();
clone.addStudent(cloneStud , i );
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (FullFloorException e) {
e.printStackTrace();
}
}
return clone;
}
@Override
public String toString(){
String s = "";
for(int i = 0; i
if(i == 0){
s += ("ROOM\t\t\tNAME\t\t\tID\t\tWRITEUPS") + " " + ("------------------------------------------------------------------------")
+ " " + (i+1) + "\t\t\t" + students[i].getName() + "\t\t\t" + students[i].getIdNumber() + "\t\t" + students[i].getNumWriteups() + " " ;
}
else{
s += ((i+1) + "\t\t\t" + students[i].getName() + "\t\t\t" + students[i].getIdNumber() + "\t\t" + students[i].getNumWriteups() + " ");
}
}
return s;
}
}
Full Floor Exception Class:
public class FullFloorException extends Exception{
private String message;
public FullFloorException(String message){
super(message);
this.message = message;
}
public String getMessage(){
return message;
}
}
Empty Floor Exception Class:
public class EmptyFloorException extends Exception {
private String message;
public EmptyFloorException(String message){
super(message);
this.message = message;
}
public String getMessage(){
return message;
}
}
Driver Class:
//clone floor needs to be fixed
//i don't think it is deep cloning because when I copy to another floor it gives me an empty floor
import java.util.Scanner;
public class ResidenceHallManager extends Student{
public static void main(String[] args){
/*Floor floora = new Floor(); // this chunk of code works that is why I don't understand my problem
Student brad = new Student("brad",0,0);
Student kennia = brad.clone();
Student b = brad.clone();
try {
floora.addStudent(brad, 0);
floora.addStudent(b, 1);
floora.addStudent(kennia, 2);
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (FullFloorException e) {
e.printStackTrace();
}
System.out.println(floora.toString());
Floor floorb = floora.clone();
System.out.println(floorb.toString());
System.out.println(floorb.getStudent(0).toString());
*/
Floor floor1 = new Floor();
Floor floor2 = new Floor();
Floor floor3 = new Floor();
Menu(floor1,floor2,floor3);
}
public static Floor selectFloor(Floor floor1, Floor floor2, Floor floor3){
int floorNum;
System.out.print("Enter 1 for Floor 1, 2 for Floor 2, or 3 for Floor 3:");
Scanner input = new Scanner(System.in);
floorNum = input.nextInt();
if(!(floorNum == 1 || floorNum == 2 || floorNum == 3)){
System.out.print(" Please enter a valid number to choose the respective floor (1-3):");
floorNum = input.nextInt();
}
switch(floorNum){
case 1:
return floor1;
case 2:
return floor2;
case 3:
return floor3;
}
return null;
}
public static void addToFloor(Floor floor1, Floor floor2, Floor floor3){
String name;
Scanner input = new Scanner(System.in);
int id, writeUps;
System.out.println("Please enter the floor you would like to place the student in:");
Floor floor = selectFloor(floor1, floor2, floor3);
Student student1 = getStudentInfo();
System.out.print("Please enter the room number you would like to place the student in:");
int position1 = input.nextInt() - 1;
try{
floor.addStudent(student1, position1);
}catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (FullFloorException e) {
e.printStackTrace();
}
System.out.println("Student: " + student1.getName() + " has been added to the floor");
}
public static void removeFromFloor(Floor floor1, Floor floor2, Floor floor3){
Scanner input = new Scanner(System.in);
System.out.println("Please enter the floor you would like to remove the student from:");
Floor floor = selectFloor(floor1, floor2, floor3);
System.out.println("Please enter the room number you would like to remove the student from:");
int position1 = input.nextInt()-1;
Student student1 = floor.getStudent(position1);
try {
floor.removeStudent(position1);
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (EmptyFloorException e) {
e.printStackTrace();
}
System.out.println("Student: " + student1.getName() + " has been removed from the floor");
}
public static void swapStudents(Floor floor1, Floor floor2, Floor floor3){
Scanner input = new Scanner(System.in);
System.out.println("Please enter the floor of the first student you'd like to swap:");
Floor floora = selectFloor(floor1, floor2, floor3);
System.out.println("Please enter the room number of the first student you'd like to swap:");
int position1 = input.nextInt()-1;
System.out.println("Please enter the floor of the second student you'd like to swap:");
Floor floorb = selectFloor(floor1, floor2, floor3);
System.out.println("Please enter the room number of the second student you'd like to swap:");
int position2 = input.nextInt()-1;
Student studenta = floora.getStudent(position1);
Student studentb = floorb.getStudent(position2);
try {
floora.removeStudent(position1);
floorb.removeStudent(position2);
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (EmptyFloorException e) {
e.printStackTrace();
}
try {
floora.addStudent(studentb, position1);
floorb.addStudent(studenta, position2);
} catch (FullFloorException e) {
e.printStackTrace();
}
System.out.println("Students: " + studenta.getName() + " and " + studentb.getName() + " have been switched" );
}
public static void moveStudent(Floor floor1, Floor floor2, Floor floor3){
Scanner input = new Scanner(System.in);
System.out.println("Please enter the floor of the student you'd like to move:");
Floor floora = selectFloor(floor1, floor2, floor3);
System.out.println("Please enter the room number of the student you'd like to move:");
int position1 = input.nextInt()-1;
Student student1 = floora.getStudent(position1);
try {
floora.removeStudent(position1);
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (EmptyFloorException e) {
e.printStackTrace();
}
System.out.println("Please enter the floor you'd like to move the student to:");
Floor floorb = selectFloor(floor1,floor2,floor3);
System.out.println("Please enter the room number you'd like to move the student to:");
int position2 = input.nextInt()-1;
try {
floorb.addStudent(student1, position2);
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (FullFloorException e) {
e.printStackTrace();
}
System.out.println(student1.getName() + " has been moved");
}
public static void copyFloor(Floor floor1, Floor floor2, Floor floor3){
System.out.println("Please select the floor you'd like to copy:");
Floor floora = selectFloor(floor1,floor2,floor3);
System.out.println("Enter the floor you'd like to copy it to:");
Floor floorb = selectFloor(floor1,floor2,floor3);
if(floorb.equals(floor1)){
floor1 = floora.clone();
System.out.println("The floor has been copied to floor 1.");
}
if(floorb.equals(floor2)){
floor2 = floora.clone();
System.out.println("The floor has been copied to floor 2.");
}
if(floorb.equals(floor3)){
floor3 = floora.clone();
System.out.println("The floor has been copied to floor 3.");
}
}
public static Student getStudentInfo(){
Scanner input = new Scanner(System.in);
System.out.print("Add a Student:");
System.out.print(" Please enter the student's name:");
String name = input.nextLine();
System.out.print("Please enter the student's ID Number:");
int id = input.nextInt();
System.out.print("Please enter the number of write-ups the student has if any:");
int writeup = input.nextInt();
Student student = new Student(name,id,writeup);
return student;
}
public static void printFloor(Floor floor1, Floor floor2, Floor floor3){
System.out.println("Enter the floor you'd like to print:");
Floor floora = selectFloor(floor1,floor2,floor3);
if(floora.equals(floor1)){
System.out.println(" Floor 1:");
}
if(floora.equals(floor2)){
System.out.println(" Floor 2:");
}
if(floora.equals(floor3)){
System.out.println(" Floor 3:");
}
if(floora.count() == 0){
System.out.println("Empty Floor.");
}
System.out.println(floora.toString());
}
public static void writeUp(Floor floor1, Floor floor2, Floor floor3){
System.out.println("From which floor would you like to write a student up:");
Floor floor = selectFloor(floor1,floor2,floor3);
Scanner input = new Scanner(System.in);
System.out.println("Enter the name of the student you would like to write up:");
String thisGuy = input.nextLine();
for(int i = 0; i
if(floor.getStudent(i).getName().equals(thisGuy)){
floor.getStudent(i).setNumWriteups(floor.getStudent(i).getNumWriteups() + 1);
System.out.println("Student: " + floor.getStudent(i).getName() + " has " + floor.getStudent(i).getNumWriteups() + " write-up(s)");
if(floor.getStudent(i).getNumWriteups() >= 3){
try {
floor.removeStudent(i);
System.out.println("The student has three write-ups and has been removed from the floor.");
} catch (IllegalArgumentException e) {
} catch (EmptyFloorException e) {
}
}
}
}
}
public static void Menu(Floor floor1, Floor floor2, Floor floor3){
System.out.println(" Please Select an Option:");
System.out.println("A) Add a student");
System.out.println("R) Remove a student");
System.out.println("S) Swap students");
System.out.println("M) Move student");
System.out.println("C) Copy Floor");
System.out.println("P) Print Floor");
System.out.println("W) Write-up student");
System.out.println("Q) Quit");
Scanner input = new Scanner(System.in);
String x = input.nextLine();
while(!(x.equalsIgnoreCase("A") || x.equalsIgnoreCase("R") || x.equalsIgnoreCase("S") || x.equalsIgnoreCase("M") || x.equalsIgnoreCase("C") || x.equalsIgnoreCase("P") || x.equalsIgnoreCase("W") || x.equalsIgnoreCase("Q"))){
System.out.println("Please enter a letter from the specified list (A,R,S,M,C,P,W,Q):");
x = input.nextLine();
}
if(x.equalsIgnoreCase("A")){
addToFloor(floor1,floor2,floor3);
Menu(floor1,floor2,floor3);
}
if(x.equalsIgnoreCase("R")){
removeFromFloor(floor1,floor2,floor3);
Menu(floor1,floor2,floor3);
}
if(x.equalsIgnoreCase("S")){
swapStudents(floor1,floor2,floor3);
Menu(floor1,floor2,floor3);
}
if(x.equalsIgnoreCase("M")){
moveStudent(floor1,floor2,floor3);
Menu(floor1,floor2,floor3);
}
if(x.equalsIgnoreCase("C")){
copyFloor(floor1,floor2,floor3);
Menu(floor1,floor2,floor3);
}
if(x.equalsIgnoreCase("P")){
printFloor(floor1,floor2,floor3);
Menu(floor1,floor2,floor3);
}
if(x.equalsIgnoreCase("W")){
writeUp(floor1,floor2,floor3);
Menu(floor1,floor2,floor3);
}
if(x.equalsIgnoreCase("Q")){
System.out.println("In a while crocodile...");
System.exit(0);
}
}
}
HOMEWORK 1 due Tuesday, February 7th no later than 6:00pm REMINDERS Be sure your code follows the coding style for CSE214 Make sure you read the warnings about academic dishonesty. Remember, all work you submit for homework assignments MUST be entirely your own work. Also, group efforts are not allowed Login to your grading account and click "Submit Assignment" to upload and submit your assignment. You are not allowed to use ArrayList, Vector or any other Java API Data Structure classes to implement this assignment except where noted You may use Scanner, InputStreamReader, or any other class that you wish for keyboard input. Stony Brook University has recently opened some new residence halls. Since these halls are much more modern than the other residence halls, the university would like to keep track of the students who live there electronically. You will write a program that will manage the students that live in a residence hall for the residence hall director. Your program will model a residence hall with three floors, each of which will be modeled by an array, containing student objects. For each student we will need to keep track of their name, id, and the number of times the student has been written up (documented). If a student has been written up 3 times, they wi be kicked out of the residence hall. To make the RA's job easier, all the students on the floor will be placed in the lowest available room number (there will be no holes in the array). If a student moves out, all the students remaining must be shifted down one room in order to make them closer to the the RA at the beginning of the hallway. Also, sometimes, maintenance work must be done on one floor, so therefore students must be moved to another floor. For the convenience of the building administrator (RHD), you will also have to implement a deep copy (clone) function, so the RHD can experiment with alternative floor plans without ruining their original, genius floor plan NOTE: All exceptions explicitly thrown in Required Classes except for lllegalArgumentException are custom exceptions that need to be made by you Required Classes Residence Hall Manager (Driver Class) public static void main (StringO args) Runs the User interface menu as shown in Required Functions o Can be changed as necessary for GUI/Android App o This method will contain three Floor objects for cloning. You may use an array of Floor objects if you like, but this is not required. We will refer to them as Floor 1, Floor 2, and Floor 3 Floor If you're familiar with Arra this works in a somewhat similar way Write a fully documented class named Floor which stores a list of Students in an array and provides an interface to interact with this list. A Floor up to 50 students at a time, so use the final variable CAPACITY 50. The class will be based on the following ADT specification private Student students
Step by Step Solution
There are 3 Steps involved in it
Step: 1
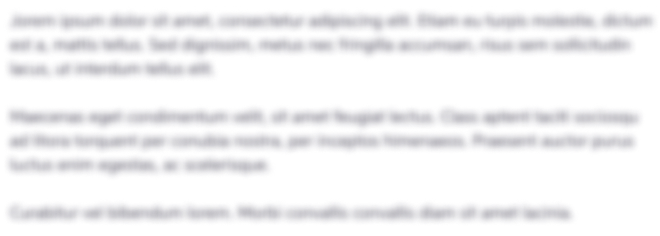
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started