Question
So I have this Java project that involves Sorted Doubly Linked list data Strutures Below are the instuctions and Program 1: Sorted Doubly Linked list
So I have this Java project that involves Sorted Doubly Linked list data Strutures
Below are the instuctions and Program 1:
Sorted Doubly Linked list
Re-do Program 1(the Unsorted-Optimized Array structure) but this time store the nodes in a sorted, doubly linked list. First convert the singly linked list class to a sorted singly linked list (70% see below). Then convert to a doubly linked (20% + 10% see below and read the footnote).
Client node definition class changes:
Add a getKey method to it..
Application class changes:
Change the class of the data structure object and, eliminate the asking of maximum number of nodes.
Data structure class SinglyLinkedList (pg 189) changes:
************************ 70% Sorted Singly linked *******************
Change the data structure class to the class on pages 189-190, and change the class name and the clients node definition class name from Listing to StudentListings (allready done)
insert (sorted)
Add a getKey mehod to the StudentListings class
search list for insertion point: while (not at end of list && key < newNodesKey)
Insert a newNode and the deepCopy at insertion point
fetch and delete (sorted speed-up)
Same search as unsorted, but should return an error when the node is not in the list or we get to a node that is greater than given key (speed-up).
*********************** 20% Doubly Linked insert and showAll**********
inner Node class:
Add the back pointer
insert (doubly linked):
Add the setting of the back pointer on all inserts (new last node a special case)
showAllBackward output the nodes in last to first order:
Make q trail p down the list till q points to the last node. Then use q to traverse the list backward.
********************** 10% delete*******************************
delete:
reset the back pointer on all deletes (delete last node a special case)
here is the project 1 program:
package database; import javax.swing.JOptionPane; public class StudentListing {//start StudentListing class private String name; private String idnumber; private String gpa; public StudentListing(String n, String id, String grd) {//start StudentListing name = n; idnumber = id; gpa = grd; }//end StudentListing StudentListing() { } public String toString( ) {//start toString return("Name: " + name + " ID number: " + idnumber + " GPA: " + gpa + " "); }//end toString public StudentListing deepCopy() {//start deepCopy StudentListing clone = new StudentListing(name, idnumber, gpa); return clone; }//end deepCopy public int compareTo(String targetKey) {//Start compareTo return(name.compareTo(targetKey)); }//End compareTo public void setIdnum(String id) {//Start setIdnum idnumber = id;// coded to demonstrate encapsulation }//end setIdnum public void inputNode() {//start inputNode name = JOptionPane.showInputDialog("Enter a name"); idnumber = JOptionPane.showInputDialog("Enter the ID Number"); gpa = JOptionPane.showInputDialog("Enter the GPA"); }//end inputNode }//end studentListing class *****************************************************************************
package database; import javax.swing.*; public class UOAUtilities {//start UOAUtilities class private int next; private int size; private StudentListing[] data; public UOAUtilities() {//Start of constructor next = 0; size = 100; data = new StudentListing[size]; }//end of constructor public UOAUtilities (int s) {//Start of constructor next = 0; data = new StudentListing[s]; size = s; }//end of constructor public boolean insert(StudentListing newStudentListing) { //insert method starts if(next >= size) // if the structure is full return false; data[next]= newStudentListing.deepCopy( ); // store a deep copy of the clients node if(data[next] == null) return false; next = next + 1; // prepare for the next insert return true; }// end of insert method public StudentListing fetch(String targetKey) {//start of fetch method StudentListing node; StudentListing temp; // access the node using a sequential search int i = 0; while ( i < next && !(data[i].compareTo(targetKey) == 0)) { i++; } if(i == next) // if node not found {JOptionPane.showMessageDialog(null, "Node was not found"); return null; } //deep copy the node's information into the client's node node = data[i].deepCopy( ); // move the node up one position in the array, unless it is the first node if(i != 0) // bubble-up accessed node { temp = data[i-1]; data[i-1] = data[i]; data[i] = temp; } return node; }// end of fetch method
public boolean delete(String targetKey) {// access the node using a sequential search int i = 0; while (i < next && !(data[i].compareTo(targetKey) == 0)) { i++; } if(i == next) // if node not found { JOptionPane.showMessageDialog(null, "The node could not be delteted becasue it was not found"); }
//move the last node into the deleted node's position data[ i] = data[ next -1]; data[next-1] = null; next = next - 1; return true; // if node found and deleted }//end of the delete method
public boolean update(String targetKey, StudentListing StudentListings) {//start update method if(delete(targetKey) == false) {JOptionPane.showMessageDialog(null, "Node not in the structure, update was unsucessful"); return false; } else if( insert(StudentListings) == false) { JOptionPane.showMessageDialog(null, "Insufficient memory update was unsucessful"); return false; } else { JOptionPane.showMessageDialog(null, "The student was updated succesffuly"); return true; // node found and updated } }// end of update method public void showAll( ) {// Start showAll method for(int i = 0; i< next; i++) System.out.println( data[i].toString());//print out all data }// end showAll method }//end of class UOAUtilities *****************************************************************************
package database; import javax.swing.*; public class Main {//START CLASS public static void main(String[] args) {//START MAIN UOAUtilities s = new UOAUtilities(); StudentListing f = new StudentListing(); int choice; String name; StudentListing c = new StudentListing(); do {//START DO choice = Integer.parseInt(JOptionPane.showInputDialog( "1 to insert a new student's information "+ "2 to fetch and output a student's information "+ "3 to delete a student's information "+ "4 to update a student's information "+ "5 to output all the student information in sorted order "+ "6 to quit the program")); if(choice < 1 || choice > 6) {//if out of range JOptionPane.showMessageDialog(null, "Number entered is out of range."); } switch(choice) { //start switch statement case 1: f.inputNode(); s.insert(f); break; case 2: JOptionPane.showMessageDialog(null, s.fetch(name = JOptionPane.showInputDialog("search for name:"))); break; case 3: JOptionPane.showMessageDialog(null, s.delete(name = JOptionPane.showInputDialog("student to delete"))); break; case 4: c.inputNode(); s.update(JOptionPane.showInputDialog(null, "student name to update:"), c); break; case 5: s.showAll(); break; }//end switch statement }//END DO while(choice != 6);//quit the program }//END MAIN }//END CLASS *****************************************************************************
package database; public class SinglyLinkedList {//START SinglyLinkedList CLASS private Node h; // list header public SinglyLinkedList() { h = new Node(); // dummy node h.l = null; h.next = null; } public boolean insert(StudentListing newListing) {//start insert Node n = new Node(); if(n == null) // out of memory return false; else {//start else n.next = h.next; h.next = n; n.l = newListing.deepCopy(); return true; }//end else }//end insert public StudentListing fetch(String targetKey) {//start fetch Node p = h.next; while (p != null && !(p.l.compareTo(targetKey) == 0)) { p = p.next; } if(p != null) return p.l.deepCopy(); else return null; }//end fetch public boolean delete(String targetKey) {//start delete Node q = h; Node p = h.next; while (p != null && !(p.l.compareTo(targetKey) == 0)) {//start while q = p; p = p.next; }//end while if(p != null) {//start if q.next = p.next; return true; }//end if else return false; }//end delete public boolean update(String targetKey, StudentListing newListing) {//start update if(delete(targetKey) == false) return false; else if(insert(newListing) == false) return false; return true; }//end update public void showAll() {//start showAll Node p = h.next; while (p != null) //continue to traverse the list {// start while System.out.println(p.l.toString( )); p = p.next; }//end while }//end showAll public class Node {//start of inner class node private StudentListing l; private Node next; public Node() {//start public Node }//end public Node }// end of inner class Node }//end SinglyLinkedList outer class
I know this is a lot of work but any help is appreciated Thank You
Step by Step Solution
There are 3 Steps involved in it
Step: 1
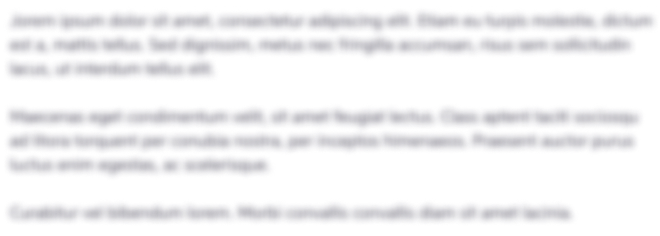
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started