Question
So my question is: Implement the Shape hierarchy create an abstract class called Shape, which will be the parent class to TwoDimensionalShape and ThreeDimensionalShape. The
So my question is:
Implement the Shape hierarchy create an abstract class called Shape, which will be the parent class to TwoDimensionalShape and ThreeDimensionalShape. The classes Circle, Square, and Triangle should inherit from TwoDimensionalShape, while Sphere, Cube, and Tetrahedron should inherit from ThreeDimensionalShape. Each TwoDimensionalShape should have the methods getArea() and getPerimeter(), which calculate the area and perimeter of the shape, respectively. Every ThreeDimensionalShape should have the methods getArea() and getVolume(), which respectively calculate the surface area and volume of the shape. Every class should have a member variable containing its dimensions for example, the Circle class should have a member variable describing its radius, while the Triangle class should have three-member variables describing the length of each side. Note that the Tetrahedron class should describe a regular tetrahedron, and as such, should only have a one-member variable. Create a Driver class with a main method to test your Shape hierarchy. The program should prompt the user to enter the type of shape they'd like to create, and then the shape's dimensions. If the shape is two dimensional, the program should print its area and its perimeter, and if it's a three-dimensional shape, its surface area and volume.
Here is the code I've written so far, followed by the Driver class. My driver class is having a problem accessing the Circle, Square, Triangle, Sphere, Cube, and Tetrahedron, also my main question is is there a way to combine more information into my Shape superclass to make this code more "compact" and precise?
import java.util.*; import java.math.*;
// superclass Shape public abstract class Shape { } // subclass TwoDimensionalShape class TwoDimensionalShape extends Shape { // r is radius; a/b/c are sides; s = (a+b+c)/2 double perimeter () {return 0.0;} double area() {return 0.0;} public String toString() { return String.format("Area: %.2f%nPerimeter: %.2f%n", area(), perimeter()); } public class Circle extends TwoDimensionalShape { // 2 pi r (per) & pi r^2 (area) private double r; public double area() {return Math.PI*this.r*this.r;} public double perimeter() {return Math.PI * 2 * this.r;} } // end circle public class Square extends TwoDimensionalShape { // 4a (per) & a^2 (area) private double side; public Square(double side) {this.side = side;} public double area () {return this.side * this.side;} public double perimeter() {return this.side * 4;} } // end square public class Triangle extends TwoDimensionalShape { //a+b+c (per) & sqrt s(s-a)(s-b)(s-c) (area) private double side1; private double side2; private double side3; public Triangle (double side1, double side2, double side3) { this.side1 = side1; this.side2 = side2; this.side3 = side3; } // end public Triangle this. public double perimeter() {return this.side1 + this.side2 + this.side3;} public double area() { double half = (this.side1 + this.side2 + this.side3)/2; double calc = Math.sqrt(half * (half-this.side1) * (half-this.side2) * (half-this.side3)); return calc; } // end perimeter } // end Triangle } // ends TwoDimensionalShape // subclass ThreeDimensionalShape class ThreeDimensionalShape extends Shape { // a is a side; r is radius public double getVolume() {return 0.0;} public double getSurfaceArea() {return 0.0;} public String toString() { return String.format("Surface area: %.2f%nVolume: %.2f%n", getSurfaceArea(), getVolume()); } // end toString public class Sphere extends ThreeDimensionalShape { // 4 pi r ^2 (area) & 4/3 pi r^3 (vol) private double r; public Sphere(double r) {this.r = r;} public double getVolume() {return (Math.PI * this.r * this.r * this.r * 4)/3.0;} public double getSurfaceArea() {return 4*Math.PI * this.r* this.r;} } // end class Sphere public class Cube extends ThreeDimensionalShape { // 6a^2 (area) & a^3 (vol) private double side; public Cube(double side) {this.side = side;} public double getVolume() {return this.side * this.side * this.side;} public double getSurfaceArea() {return this.side * this.side * 3;} } // end class Cube public class Tetrahedron extends ThreeDimensionalShape { // sqrt of 3a^2 (area) & a^3/6 sqrt 2 (vol) private double side; public Tetrahedron(double side) { this.side = side;} public double getVolume() {return this.side * this.side *this.side/Math.sqrt(2)*(6);} public double getSurfaceArea() {return this.side * this.side *Math.sqrt(3);} } // end class Tetrahedron } // end class ThreeDimensionalShape
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
import java.util.Scanner; public class ShapeDriver { public static void main(String[] args) { Object obj; int first; int second; double r; //side input Scanner scanner = new Scanner(System.in); System.out.print("Enter 1Two dimensional shape 2Three dimensional shape:"); first = scanner.nextInt(); if (first == 1) { // can do nested if statements System.out.printf("Enter 1 Circle\2Square 3Triangle:"); second = scanner.nextInt(); } if (first == 2) { System.out.print("Enter 1 Sphere 2Cube 3Tetrahedron:"); second = scanner.nextInt(); } else { System.out.printf("Invalid Entry."); break; } if(first == 1 & second == 1) { System.out.print("Enter Radius of circle:"); r = scanner.nextDouble(); obj = new Circle(r); System.out.print(obj.toString()); } if (first ==1 & second == 2) { System.out.print("Enter side of square:"); r = scanner.nextDouble(); obj = new Square(r); System.out.print(obj.toString()); } if (first ==1 & second == 3) { System.out.print("Enter side of the Triangle:"); double side1 = scanner.nextDouble(); System.out.print("Enter side of the Triangle:"); double side2 = scanner.nextDouble(); System.out.print("Enter side of the Triangle:"); double side3 = scanner.nextDouble(); obj = new Triangle(side1, side2, side3); System.out.print(obj.toString()); } if (first == 2 & second == 1) { System.out.println("Enter radius of sphere:"); r = scanner.nextDouble(); obj = new Sphere(r); System.out.print(obj.toString()); } if (first ==2 & second == 2) { System.out.print("Enter side of cube:"); r = scanner.nextDouble(); obj = new Cube(r); System.out.println(obj.toString()); } if (first == 2 & second == 3) { System.out.println("Enter side of tetrahedron:"); r = scanner.nextDouble(); obj = new Tetrahedron(r); System.out.println(obj.toString()); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
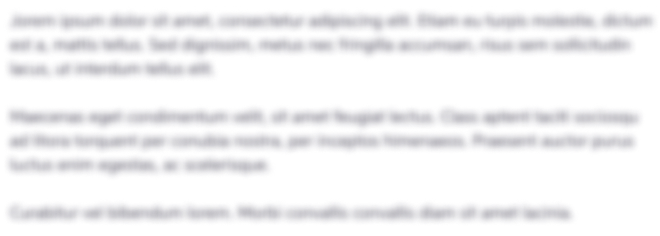
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started