Question
So this is what I need to do..... This assignment consists of writing a program with multiple classes and arrays. Create a Student class with
So this is what I need to do.....
This assignment consists of writing a program with multiple classes and arrays.
- Create a Student class with
- Private instance variables for first and last name, three integer scores, the average ,and letter grade
- A constructor with values
- Methods to set and get the values of the instance variables
- Methods to get the average and letter grade
- Method to calculate the average of the three scores and to assign this value to the instance variable average
- Method to determine the letter grade and to assign this value to the instance variable grade
- Method to format and print the name, scores, average ( to two decimal places), and letter grade. (using printf() method)
- Create a StudentMod3 class
- Declare an array of objects of type Student for three people
- One person at a time, read their first and last name, and three scores, into each of the three elements of the array
- Determine the average and letter grade of all the three. Letter grade is based on the following criteria - average 90-100 grade is A, 80-89.99 grade is B, 70-79.99 grade is C, all others F (use a nested if)
- Output the name, scores, average, and letter grade of the three people
I have the majority of it, I just have errors popping up at the end of it saying the public class studentmod3 is an illegal start to the expression, and thats where my issues start, and this is what I have so far.....
import java.util.Scanner; public class Assignment3 {
// TODO code application logic here public class Student {
private String firstName; private String lastName; private final int score1; private final int score2; private final int score3; private double average; private char letterGrade;
public Student(String firstName, String lastName, int score1, int score2, int score3) { this.firstName = firstName; this.lastName = lastName; this.score1 = score1; this.score2 = score2; this.score3 = score3;
}
public void calculateAverage() { average = (score1 + score2 + score3) / 3.0; }
public String getFirstName() { return firstName; }
public void setFirstName(String firstName) { this.firstName = firstName; }
public String getLastName() { return lastName; }
public void setLastName(String lastName) { this.lastName = lastName; }
public int getScoreOne() { return score1; } public int getScoreTwo() { return score2; } public int getScoreThree() { return score3; } public double getAverage() { return average; } public void calculateLetterGrade() { calculateAverage(); if (average >= 90) { letterGrade = 'A'; } else if (average >= 80) { letterGrade = 'B'; } else if (average >= 70) { letterGrade = 'C'; } else { letterGrade = 'F'; } } public char getLetterGrade() { return letterGrade; }
public void printStudentDetails() { String fullName = firstName + " " + lastName; System.out.printf("%-20s%4d%4d%4d%8.2f%4s ", fullName, score1, score2, score3, average, String.valueOf(letterGrade)); } }
public static Scanner scanner = new Scanner(System.in); public static void main(String[] args) {
public class StudentMod3 {
Student[] students = new Student[3]; for (int i = 0; i < 3; i++) { System.out.println("Student " + (i + 1) + " -"); System.out.print("Enter First Name : "); String fName = scanner.nextLine(); System.out.print("Enter Second Name : "); String lName = scanner.nextLine(); System.out.print("Enter First Score : "); int score1 = scanner.nextInt(); System.out.print("Enter Second Score : "); int score2 = scanner.nextInt(); System.out.print("Enter Third Score : "); int score3= scanner.nextInt(); students[i] = new Student(fName,lName,score1,score2,score3); students[i].calculateAverage(); students[i].calculateLetterGrade(); scanner.nextLine(); }
for(Student student:students){ student.printStudentDetails(); } } } } ---------------------END CODE-----------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
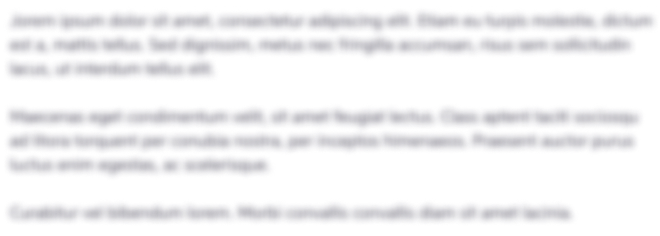
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started