Question
Socket programming client.c : /* client.c - code for example client program that uses TCP */ #ifndef unix #define WIN32 #include #include #else #define closesocket
Socket programming
client.c :
/* client.c - code for example client program that uses TCP */
#ifndef unix
#define WIN32
#include
#include
#else
#define closesocket close
#include
#include
#include
#include
#include
#endif
#include
#include
#define PROTOPORT 5193 /* default protocol port number */
extern int errno;
char localhost[] = "localhost"; /* default host name */
/*------------------------------------------------------------------------
* * Program: client
* *
* * Purpose: allocate a socket, connect to a server, and print all output
* * Syntax: client [ host [port] ]
* *
* * host - name of a computer on which server is executing
* * port - protocol port number server is using
* *
* * Note: Both arguments are optional. If no host name is specified,
* * the client uses "localhost"; if no protocol port is
* * specified, the client uses the default given by PROTOPORT.
* *
* *------------------------------------------------------------------------
* */
main(argc, argv)
int argc;
char *argv[];
{
struct hostent *ptrh; /* pointer to a host table entry */
struct protoent *ptrp; /* pointer to a protocol table entry */
struct sockaddr_in sad; /* structure to hold an IP address */
int sd; /* socket descriptor */
int port; /* protocol port number */
char *host; /* pointer to host name */
int n; /* number of characters read */
char buf[1000]; /* buffer for data from the server */
#ifdef WIN32
WSADATA wsaData;
WSAStartup(0x0101, &wsaData);
#endif
memset((char *)&sad,0,sizeof(sad)); /* clear sockaddr structure */
sad.sin_family = AF_INET; /* set family to Internet */
/* Check command-line argument for protocol port and extract */
/* port number if one is specified. Otherwise, use the default */
/* port value given by constant PROTOPORT */
if (argc > 2) { /* if protocol port specified */
port = atoi(argv[2]); /* convert to binary */
} else {
port = PROTOPORT; /* use default port number */
}
if (port > 0) /* test for legal value */
sad.sin_port = htons((u_short)port);
else { /* print error message and exit */
fprintf(stderr,"bad port number %s ",argv[2]);
exit(1);
}
/* Check host argument and assign host name. */
if (argc > 1) {
host = argv[1]; /* if host argument specified */
} else {
host = localhost;
}
/* Convert host name to equivalent IP address and copy to sad. */
ptrh = gethostbyname(host);
if ( ((char *)ptrh) == NULL ) {
fprintf(stderr,"invalid host: %s ", host);
exit(1);
}
memcpy(&sad.sin_addr, ptrh->h_addr, ptrh->h_length);
/* Map TCP transport protocol name to protocol number. */
if ( ((int)(ptrp = getprotobyname("tcp"))) == 0) {
fprintf(stderr, "cannot map \"tcp\" to protocol number");
exit(1);
}
/* Create a socket. */
sd = socket(PF_INET, SOCK_STREAM, ptrp->p_proto);
if (sd
fprintf(stderr, "socket creation failed ");
exit(1);
}
/* Connect the socket to the specified server. */
if (connect(sd, (struct sockaddr *)&sad, sizeof(sad))
fprintf(stderr,"connect failed ");
exit(1);
}
/* Repeatedly read data from socket and write to user.s screen. */
n = recv(sd, buf, sizeof(buf), 0);
while (n > 0) {
write(1,buf,n);
printf("n: %d ", n);
n = recv(sd, buf, sizeof(buf), 0);
}
printf("n: %d ", n);
/* Close the socket. */
closesocket(sd);
/* Terminate the client program gracefully. */
exit(0);
} server.c :
/* server.c - code for example server program that uses TCP */
#ifndef unix
#define WIN32
#include
#include
#else
#define closesocket close
#include
#include
#include
#include
#endif
#include
#include
#define PROTOPORT 5193 /* default protocol port number */
#define QLEN 6 /* size of request queue */
int visits = 0; /* counts client connections */
/*------------------------------------------------------------------------
* * Program: server
* *
* * Purpose: allocate a socket and then repeatedly execute the following:
* * (1) wait for the next connection from a client
* * (2) send a short message to the client
* * (3) close the connection
* * (4) go back to step (1)
* * Syntax: server [ port ]
* *
* * port - protocol port number to use
* *
* * Note: The port argument is optional. If no port is specified,
* * the server uses the default given by PROTOPORT.
* *
* *------------------------------------------------------------------------
* */
main(argc, argv)
int argc;
char *argv[];
{
struct hostent *ptrh; /* pointer to a host table entry */
struct protoent *ptrp; /* pointer to a protocol table entry */
struct sockaddr_in sad; /* structure to hold server.s address */
struct sockaddr_in cad; /* structure to hold client.s address */
int sd, sd2; /* socket descriptors */
int port; /* protocol port number */
int alen; /* length of address */
char buf[1000]; /* buffer for string the server sends */
#ifdef WIN32
WSADATA wsaData;
WSAStartup(0x0101, &wsaData);
#endif
memset((char *)&sad,0,sizeof(sad)); /* clear sockaddr structure */
sad.sin_family = AF_INET; /* set family to Internet */
sad.sin_addr.s_addr = INADDR_ANY; /* set the local IP address */
/* Check command-line argument for protocol port and extract */
/* port number if one is specified. Otherwise, use the default */
/* port value given by constant PROTOPORT */
if (argc > 1) { /* if argument specified */
port = atoi(argv[1]); /* convert argument to binary */
} else {
port = PROTOPORT; /* use default port number */
}
if (port > 0) /* test for illegal value */
sad.sin_port = htons((u_short)port);
else { /* print error message and exit */
fprintf(stderr,"bad port number %s ",argv[1]);
exit(1);
}
/* Map TCP transport protocol name to protocol number */
if ( ((int)(ptrp = getprotobyname("tcp"))) == 0) {
fprintf(stderr, "cannot map \"tcp\" to protocol number");
exit(1);
}
/* Create a socket */
sd = socket(PF_INET, SOCK_STREAM, ptrp->p_proto);
if (sd
fprintf(stderr, "socket creation failed ");
exit(1);
}
/* Bind a local address to the socket */
if (bind(sd, (struct sockaddr *)&sad, sizeof(sad))
fprintf(stderr,"bind failed ");
exit(1);
}
/* Specify size of request queue */
if (listen(sd, QLEN)
fprintf(stderr,"listen failed ");
exit(1);
}
/* Main server loop - accept and handle requests */
while (1) {
alen = sizeof(cad);
if ( (sd2=accept(sd, (struct sockaddr *)&cad, &alen))
fprintf(stderr, "accept failed ");
exit(1);
}
visits++;
sprintf(buf,"This server has been contacted %d time%s ",
visits,visits==1?".":"s.");
send(sd2,buf,strlen(buf),0);
closesocket(sd2);
}
}
Programming Question (50 points) Please read chapter 3 to have a basic understanding about socket programming. After this, please download "example socket program" (socket program.tar.gz) in the Blackboard website. Please upload socket program.tar.gz to a Linux machine, then run 1. gunzip socket _program.tar.gz 2. tar xvf socket program.tax ill obtain the list of how to use these socket programs. You w socket programs. Please refer to video "Socket Demo" to see two programs and make them work. Please a. b. Explain the basic functionality of server.c and client.c Run server and client. C. Turn in a screenshot to show how you run them. Programming Question (50 points) Please read chapter 3 to have a basic understanding about socket programming. After this, please download "example socket program" (socket program.tar.gz) in the Blackboard website. Please upload socket program.tar.gz to a Linux machine, then run 1. gunzip socket _program.tar.gz 2. tar xvf socket program.tax ill obtain the list of how to use these socket programs. You w socket programs. Please refer to video "Socket Demo" to see two programs and make them work. Please a. b. Explain the basic functionality of server.c and client.c Run server and client. C. Turn in a screenshot to show how you run themStep by Step Solution
There are 3 Steps involved in it
Step: 1
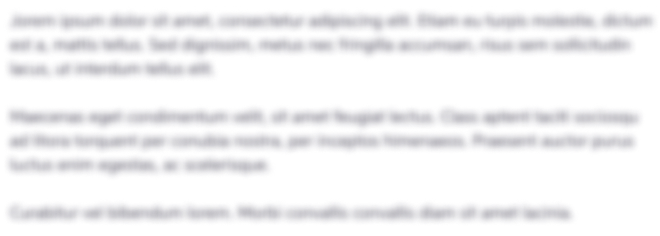
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started