Question
Socket Programming, Client-Server Programming, Concurrent Server with Threads WORTH 10 QUESTIONS! POSTED 10 TIMES!!! ANSWER ONE SCORE ALL!!!!! ANSWER ACCORDING TO FORMAT!!!!! ANSWER EVERYTHING CORRECTLY
Socket Programming, Client-Server Programming, Concurrent Server with Threads
WORTH 10 QUESTIONS! POSTED 10 TIMES!!! ANSWER ONE SCORE ALL!!!!! ANSWER ACCORDING TO FORMAT!!!!! ANSWER EVERYTHING CORRECTLY AND NO NONSENSE PLS!!!!!
Note. You should use the sample code (conServerThread.c) in Week11 Activity & Week12 Activity, Thread Prog in Week10, and the sqlite3 programs in Week05 Activity.
A Concurrent Server is up and run, waiting for any client to connect and send a series of transactions (each request is a Unix/Linux command to be processed by the server). When a client is connected to the server, the server will create a worker thread to serve the connected client and thus to free the server to wait for any other incoming clients.
Each worker thread (assigned to serve a client) of the server is in a loop to: (1) receive a transaction (a command) from its client, (2) process each transaction (a command), (3) send the outcome of the transaction back to the client and (4) go back to the loop, until it receives a command "exit" from the client to terminate its loop (serving the current client).
The server and client codes are incrementally built by adding more code from the previous parts.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Client.c sample code -------------------------------------------------------------------------------------------------------------------------------------------------------------
#include
#include
#include
#include
#include
#include
#include
#define MAXLINE 4096 /*max text line length*/
#define SERV_PORT 10010 /*port*/
int
main(int argc, char **argv)
{
int sockfd;
struct sockaddr_in servaddr;
char sendline[MAXLINE], recvline[MAXLINE];
// alarm(300); // to terminate after 300 seconds
//basic check of the arguments
//additional checks can be inserted
if (argc !=2) {
perror("Usage: TCPClient
exit(1);
}
//Create a socket for the client
//If sockfd<0 there was an error in the creation of the socket
if ((sockfd = socket (AF_INET, SOCK_STREAM, 0)) <0) {
perror("Problem in creating the socket");
exit(2);
}
//Creation of the socket
memset(&servaddr, 0, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_addr.s_addr= inet_addr(argv[1]);
// servaddr.sin_port = htons(argv[2]);
servaddr.sin_port = htons(SERV_PORT); //convert to big-endian order
//Connection of the client to the socket
if (connect(sockfd, (struct sockaddr *) &servaddr, sizeof(servaddr))<0) {
perror("Problem in connecting to the server");
exit(3);
}
while (fgets(sendline, MAXLINE, stdin) != NULL) {
send(sockfd, sendline, strlen(sendline), 0);
if (recv(sockfd, recvline, MAXLINE,0) == 0){
//error: server terminated prematurely
perror("The server terminated prematurely");
exit(4);
}
printf("%s", "String received from the server: ");
fputs(recvline, stdout);
}
exit(0);
}
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
conServerThread.c sample code -------------------------------------------------------------------------------------------------------------------------------------------------------------
#include
#include
#include
#include
#include
/*
CONCURRENT SERVER: THREAD EXAMPLE
Must be linked with the "pthread" library also, e.g.:
cc -o example example.c -lnsl -lsocket -lpthread
This program creates a connection socket, binds a name to it, then
listens for connections to the sockect. When a connection is made,
it accepts messages from the socket until eof, and then waits for
another connection...
This is an example of a CONCURRENT server -- by creating threads several
clients can be served at the same time...
This program has to be killed to terminate, or alternately it will abort in
120 seconds on an alarm...
*/
#define PORTNUMBER 10010
struct serverParm {
int connectionDesc;
};
void *serverThread(void *parmPtr) {
#define PARMPTR ((struct serverParm *) parmPtr)
int recievedMsgLen;
char messageBuf[1025];
/* Server thread code to deal with message processing */
printf("DEBUG: connection made, connectionDesc=%d ",
PARMPTR->connectionDesc);
if (PARMPTR->connectionDesc < 0) {
printf("Accept failed ");
return(0); /* Exit thread */
}
/* Receive messages from sender... */
while ((recievedMsgLen=
read(PARMPTR->connectionDesc,messageBuf,sizeof(messageBuf)-1)) > 0)
{
recievedMsgLen[messageBuf] = '\0';
printf("Message: %s ",messageBuf);
if (write(PARMPTR->connectionDesc,"GOT IT\0",7) < 0) {
perror("Server: write error");
return(0);
}
}
close(PARMPTR->connectionDesc); /* Avoid descriptor leaks */
free(PARMPTR); /* And memory leaks */
return(0); /* Exit thread */
}
main () {
int listenDesc;
struct sockaddr_in myAddr;
struct serverParm *parmPtr;
int connectionDesc;
pthread_t threadID;
/* For testing purposes, make sure process will terminate eventually */
alarm(120); /* Terminate in 120 seconds */
/* Create socket from which to read */
if ((listenDesc = socket(AF_INET, SOCK_STREAM, 0)) < 0) {
perror("open error on socket");
exit(1);
}
/* Create "name" of socket */
myAddr.sin_family = AF_INET;
myAddr.sin_addr.s_addr = INADDR_ANY;
myAddr.sin_port = htons(PORTNUMBER);
if (bind(listenDesc, (struct sockaddr *) &myAddr, sizeof(myAddr)) < 0) {
perror("bind error");
exit(1);
}
/* Start accepting connections.... */
/* Up to 5 requests for connections can be queued... */
listen(listenDesc,5);
while (1) /* Do forever */ {
/* Wait for a client connection */
connectionDesc = accept(listenDesc, NULL, NULL);
/* Create a thread to actually handle this client */
parmPtr = (struct serverParm *)malloc(sizeof(struct serverParm));
parmPtr->connectionDesc = connectionDesc;
if (pthread_create(&threadID, NULL, serverThread, (void *)parmPtr)
!= 0) {
perror("Thread create error");
close(connectionDesc);
close(listenDesc);
exit(1);
}
printf("Parent ready for another connection ");
}
}
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part 1. -------------------------------------------------------------------------------------------------------------------------------------------------------------
#1. Design and implement the Server (a3p1Server1.c) and the Client#1 (a3p1Client1.c). The concurrent server is up and listening. The Client#1 connects to the server to do each command from client in a loop. The server (when it starts) takes one argument (its port number) to bind and listen for client's connection. The client (when it starts) takes two arguments (IP address and Port number of the server) to be used to connect.
#2. The Client#1 is up and running a loop to: (1) send a command to the server, (2) wait and receive the result of the command from the server, (3) display the result, (4) go into a sleep for 3 seconds, and then (5) wake up, go back in the loop. For this example, the Client#1 keeps one command ("date") each time.
The Client #1 sends a command string (e.g., "ls") to the server to be processed. Once the Client #1 is connected to the server, the server creates a worker thread (Thread #1). The server receives a command string to be run and sends its result back to the client. The server lets a worker thread receive (read via socket) the command string from the client (e.g., "date"). Then it runs the command and sends the result back to the client.
#3. The Client #1 then receives the result from the server and displays it to the console.
In addition, the client outputs (appends) the result to a log file (a3Client1.log). The log file of the client is opened in the beginning of the client program, writing each result from the server, with a proper heading. The log file is closed at the end of the client program, and then the client displays a message (with a time of the day and its process ID or thread ID) to the console.
#4. Then the Client#1 goes into sleep for 3 seconds. And then the client wakes up and displays a message (with a time of the day and its process ID or thread ID) to the console. Then the client repeats this cycle until it is killed by the user (via CTRL+C or kill command).
#5. In addition, the Server opens its log file (a3p1Server.log) to be shared by all of its threads. Then each server's thread displays a message (with a time of the day with PID and its thread ID) to the console saying that it is going to process a client's request with a proper heading (Process ID or Thread ID with the time of day).
#6. Let both client and server run together for 10 to 30 seconds. Then the client sends "exit" to terminate its request and to terminate itself (or you can kill it manually). The server will be killed manually by you after the client is terminated.
As the server returns the output to the client each time, the client displays the output (with a heading of the output) to the console. To terminate the client, you should use kill command or CTRL+C to kill each client first and then the server.
** Clean up any process (server or client) with kill command
After all are done, kill the server (ps to find the pid of the server, and kill command to kill it).
Use ps and netstat commands to check the server terminated and the port is now freed (available).
(Or you may set up a timer for some time (e.g., 5 minutes) to be interrupted and terminate itself.)
** For this task, you should have two separate login-sessions (e.g., ssh or MobaXterm sessions login for one session to run one client and the other session to run the server). With this setup, you can show one client working with one server concurrently.
Part 2. -------------------------------------------------------------------------------------------------------------------------------------------------------------
Once all tasks in Part1 are working with one server and one client, you will do the same tasks (with a few exceptions) with one server and two clients (Client#1 and Client#2) running concurrently.
#1. The Client#1 goes in a loop of sending a few commands and receiving its results. The loop is:
send the command: " date; ls -l junk.txt; touch junk.txt; ls -l junk.txt "
sleep 3
#2. The Client#2 goes in a loop of sending a few commands and receiving its results. The loop is:
send the command: " date; ls -l junk.txt; rm -f junk.txt; ls -l junk.txt "
sleep 2
#3. The server will do the same as in Part1 for each client. Each client will be served by a worker-thread of the server (Thread#1 for Client#1 and Thread#2 for Client#2). The clients and the server do the same and output (1) command sent or received and (2) its result being sent or received, output to console and also to a log file (of server and of clients) as in Part1.
#4. Let two clients and one server be run together for 10 to 30 seconds. Then each client sends "exit" to terminate its request and to terminate itself (or you can kill it manually). The server will be killed manually by you after all clients are terminated.
As the server returns the output to the client each time, the client displays the output (with a heading of the output and time of day) to the console. To terminate the client, you should use kill command or CTRL+C to kill each client first and then the server.
** Clean up any process (server or client) with kill command
After all done, kill the server (ps to find the pid of the server, and kill command to kill it).
Use ps and netstat commands to check the server terminated and the port is now freed (available).
(Or you may set up a timer for some time (e.g., 5 minutes) to be interrupted and terminate itself.)
** For this task, you should have three separate login sessions (e.g., ssh or MobaXterm sessions login to each session to run each client and one session to run the server). With this setup, you can show two clients working with one server concurrently.
Part 3. -------------------------------------------------------------------------------------------------------------------------------------------------------------
Once all tasks in Part2 are working with one server and two clients, you will do the same tasks (with a few exceptions) with one server and two clients (Client#1 and Client#2 and Client#3) running concurrently.
#1. The Client#1 goes in a loop of sending a few commands and receiving its results. The loop is:
send the command: " date; ls -l junk.txt; touch junk.txt "
sleep 2
#2. The Client#2 goes in a loop of sending a few commands and receiving its results. The loop is:
send the command: "date; ls -l junk.txt; rm -f junk.txt "
sleep 3
#3. The Client#3 goes in a loop of sending a few commands and receiving its results. The loop is:
send the command: "date; ls -l junk.txt; uname -a "
sleep 1
#4. The server will do the same as in Part2 for each client. Each client will be served by a child-process or a worker-thread of the server. The clients and the server do the same and to output (1) command sent or received and (2) its result being sent or received, output to console and also to a log file (of server and of clients) as in Part2.
#5. Let three clients and one server be run together for 10 to 30 seconds. Then each client sends "exit" to terminate its request and to terminate itself (or you can kill it manually). The server will be killed manually by you after all clients are terminated.
As the server returns the output to the client each time, the client displays the output (with a heading of the output and time of day) to the console. To terminate the client, you should use kill command or CTRL+C to kill each client first and then the server.
** Clean up any process (server or client) with kill-command
After all done, kill the server (ps to find the pid of the server, and kill command to kill it).
Use ps and netstat commands to check the server terminated and the port is now freed (available).
(Or you may set up a timer for some time (e.g., 5 minutes) to be interrupted and terminate itself.)
** For this task, you should have four separate login sessions (e.g., ssh or MobaXterm sessions login to each session to run each client and one session to run the server). With this setup, you can show three clients working with one server concurrently.
Part 4. Makefile -------------------------------------------------------------------------------------------------------------------------------------------------------------
Provide Makefile
ANSWER FORMAT-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part1 Server code.
Your server program code listing here - a3p1Server.c
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part1 Client code.
Your client program code listing here - a3p1Client1.c
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part1 Client#1 Log
Run log of Client#1. Client to put its output to a log file (a3p1Client1Log.txt).
Your program runs for the server mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part1 Server Log
Part1 Server Output Run-log of Server with Client#1 to a log file (a3p1ServerLog.txt)
Server (a Thread serving a client) to put (append) the output to a log file.
Your program runs for the client mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part1 Clean-up any server or client still running.
** Clean up any process (server or client) with kill-command
After all are done, kill the server (ps to find the pid of the server, and kill command to kill it).
Use ps and netstat commands to check the server terminated and the port is now freed (available).
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part2 Server Code a3p2Server.c
Your server program code listing here - a3p2Server.c
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part2 Client#2 Code a3p2Client1.c
Your client program code listing here - a3p2Client2.c
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part2 Client#2 Code a3p2Client2.c
Your client program code listing here - a3p2Client2.c
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part2 Client#1 Log (from Part1)
Run log of Client#1. Client to put its output to a log file (a3p2Client1.log).
This is the same client program in Part1.
Your program runs for the server mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part2 Client#2 Log
Run log of Client#2. Client to put its output to a log file (a3p2Client2.log).
Your program runs for the server mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part2 Server Log
Part2 Server Output Run log of Server with Client#1 and Client#2 to a log file (a3p2Server.log)
Server (a Thread serving a client) to put (append) the output to a log file. Your program runs for the client mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part2 Clean-up any server or client still running.
** Clean up any process (server or client) with kill-command
After all done, kill the server (ps to find the pid of the server, and kill command to kill it).
Use ps and netstat commands to check the server terminated and the port is now freed (available).
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part3 Server Code a3p3Server.c
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part3 Client#3 Code a3p3Client3.c
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part3 Client#1 Log (from Part1)
Run log of Client#1. Client to put its output to a log file (a3p3Client1.log).
This is the same client program in Part1.
Your program runs for the server mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part3 Client#2 Log
Run log of Client#2. Client to put its output to a log file (a3p3Client2.log).
Your program runs for the server mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part3 Client#3 Log
Run log of Client#3. Client to put its output to a log file (a3p3Client3.log).
Your program runs for the server mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part3 Server Log
Part2 Server Output Run log of Server with Client#1 and Client#2 to a log file (a3p3Server.log)
Server (a Thread serving a client) to put (append) the output to a log file. Your program runs for the client mentioned above. Place here only the relevant run log only, and for each task with a proper heading. Copy and paste the console-log here. Your listing here should show only the relevant and essential ones to show that you have done your work.
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part3 Clean-up any server or client still running.
** Clean up any process (server or client) with kill-command
After all done, kill the server (ps to find the pid of the server, and kill command to kill it).
Use ps and netstat commands to check the server terminated and the port is now freed (available).
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Part 4 Makefile
Part4 Makefile listing
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
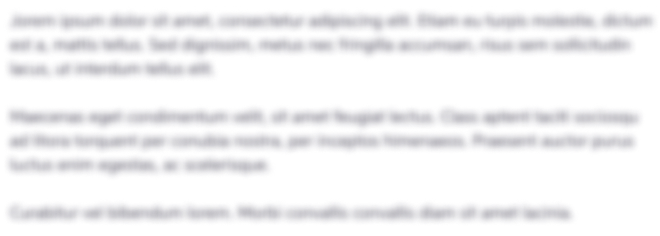
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started