Question
Software versioning is the process of assigning either unique version names or unique version numbers to unique states of computer software (Links to an external
Software versioning is the process of assigning either unique version names or unique version numbers to unique states of computer software (Links to an external site.)Links to an external site.. Software companies typically use incrementing sequences to version their software products. For example, they may have:
major#.minor#.revision#
In principle the major number is increased when there are significant jumps in functionality such as changing the framework which could cause incompatibility with interfacing systems, the minor number is incremented when only minor features or significant fixes have been added, and the revision number is incremented when minor bugs are fixed (for more information see http://en.wikipedia.org/wiki/Software_versioning (Links to an external site.)Links to an external site.).
Write the following function (please follow the EXACT function signature) that compares the two version strings, ver1 and ver2, of a software product to determine which product version is the latest:
int compareVersions(string ver1, string ver2)
If ver1 > ver2: return 1
if ver1 < ver2: return -1
otherwise, return 0.
Examples:
7.1.1 is the 7th major production with the first minor release and revision number 1.
0.1 < 0.2
0.13.1 < 0.13.2
1.0 = 1.0
6 = 6
0 = 0
1.1.0 < 1.1.1 < 1.1.2 < 1.2.0 < 1.2.1 < 1.3.1 < 8.8.8
1 < 1.1
1.1 = 1.1.0
256 < 300.2.1
0.1 < 1
3 < 3.1.1
3.1.1 < 4
.0.4 < .1
.2 > .1.1
Constraints / Assumptions:
The version strings are non-empty
The version strings contain only digits '0'-'9' and the '.' (dot) character
The '.' (dot) character is not a decimal point; it is used to separate major/minor/build sequences (see Wiki above for more information
The major # is assumed to be 0 when followed by a '.' dot (namely .1 is the same as 0.1, .3.1 is the same as 0.3.1)
Your main() function isn't graded; only the above referenced function is.
Failure to follow the same exact function signature receives -1 logic point deduction
You may test your function in the following fashion, for example:
int main() { cout << compareVersions("1.0", "1.1") << endl; cout << compareVersions("2.0", "2.0.1") << endl; ... return 0; }
Extend your compareVerisions(string, string) function to support long version numbers containing more than major, minor, and release #s. For example, you may have 2.3.2.2.3.1.1.5.3.5.6.2 or 1.1.1.1.1.1.1 - the list goes on.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
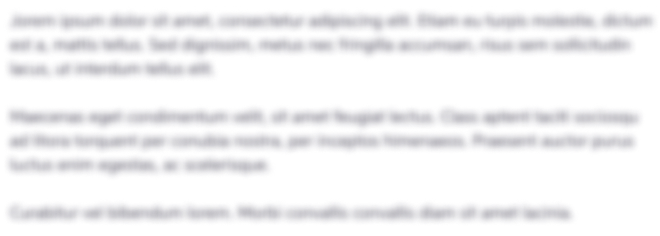
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started