Question
Solve the Consumer/Producer problem using semaphores. A skeleton program (Save it as producer_consumer.c) is provided to you: The main function creates 2 threads: consumer represents
Solve the Consumer/Producer problem using semaphores. A skeleton program (Save it as producer_consumer.c) is provided to you: The main function creates 2 threads: consumer represents the consumer and executes the consume function, and producer represents the producer and executes the produce function. You should declare and initialize 3 semaphores. Those semaphores should be used in the consume(..) and produce(...) functions.
#include #include #include #define BUFFER_SIZE 10 int buffer[BUFFER_SIZE]; int in, out; int num; void *produce( void *ptr ) { int item; while(1){ usleep(rand() % 1000); //sleep printf("Producer wants to produce. "); //critical section below item = rand() % 10; num++; buffer[in] = item; in = (in + 1) % BUFFER_SIZE; //critical section above printf("Producer entered %d. Buffer Size = %d ", item, num); } }
void *consume( void *ptr ) { int item; while(1){ usleep(rand() % 1000); //sleep printf("Consumer wants to consume. "); //critical section below num--; int item = buffer[out]; out = (out + 1) % BUFFER_SIZE; //critical section above printf("Consumer consumed %d. Buffer Size = %d ", item, num); } }
int main() { pthread_t consumer, producer; out = 0; //index of the item to be consumed next in = 0; //index of the item to be produced next num = 0; //number of items in the buffer srand(time(NULL)); pthread_create( &consumer, NULL, consume, NULL); pthread_create( &producer, NULL, produce, NULL); pthread_join( producer, NULL); pthread_join( consumer, NULL); pthread_exit(NULL); } |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
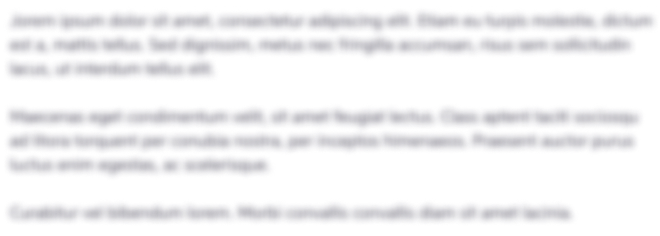
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started