Question
Solve this in python please class Fraction: def __init__(self, top: int, bottom: int) -> None: Initialize self with numerator top and denominator bottom. Raise a
Solve this in python please
class Fraction: def __init__(self, top: int, bottom: int) -> None: """Initialize self with numerator top and denominator bottom.
Raise a ValueError exception if bottom is 0.
When __init__ returns, this fraction will be in reduced form. This means that: (1) if the numerator is equal to 0, the denominator is always 1. (2) if the numerator is not equal to 0, the denominator is always positive. This means that negative fractions always have a negative numerator and a positive denominator. (3) the numerator and denominator have no common divisors other than 1.
>>> Fraction(3, 4) Fraction(3, 4) >>> Fraction(6, 8) Fraction(3, 4) >>> Fraction(-3, 4) Fraction(-3, 4) >>> Fraction(3, -4) Fraction(-3, 4) >>> Fraction(-3, -4) Fraction(3, 4) >>> Fraction(0, 5) Fraction(0, 1) """
# This method must be modified to match the specification # in the docstring.
self._num = top self._den = bottom
def __str__(self) -> str: """Return a string representation of self in the format: top/bottom.
>>> f = Fraction(3, 4) >>> str(f) '3/4' """ return "{0}/{1}".format(self._num, self._den)
def __repr__(self) -> str: """Return a string representation of self in the format: Fraction(top, bottom).
>>> f = Fraction(3, 4) >>> f Fraction(3, 4) >>> f = Fraction(3, -4) >>> f Fraction(-3, 4) """ raise NotImplementedError("__repr__ hasn't been implemented.")
def numerator(self) -> int: """Return the numerator of self.
>>> f = Fraction(3, 4) >>> f.numerator() 3 """ raise NotImplementedError("numerator hasn't been implemented.")
def denominator(self) -> int: """Return the denominator of self.
>>> f = Fraction(3, 4) >>> f.denominator() 4 """ raise NotImplementedError("denominator hasn't been implemented.")
def __eq__(self, other_fraction) -> bool: """Return True if self equals other_fraction.
>>> f1 = Fraction(3, 4) >>> f2 = Fraction(6, 8) >>> f1 == f2 True >>> f2 = Fraction(1, 2) >>> f1 == f2 False """ first_num = self._num * other_fraction._den second_num = other_fraction._num * self._den
return first_num == second_num
def __lt__(self, other_fraction) -> bool: """Return True if self is less than other_fraction.
>>> f1 = Fraction(3, 4) >>> f2 = Fraction(6, 8) >>> f1 < f2 False >>> f2 = Fraction(7, 8) >>> f1 < f2 True """ raise NotImplementedError("__lt__ hasn't been implemented.")
def __le__(self, other_fraction) -> bool: """Return True if self is less than or equal to other_fraction.
>>> f1 = Fraction(3, 4) >>> f2 = Fraction(6, 8) >>> f1 <= f2 True >>> f2 = Fraction(7, 8) >>> f1 <= f2 True >>> f2 <= f1 False """ raise NotImplementedError("__le__ hasn't been implemented.")
def __add__(self, other_fraction): """Return the sum of self and other_fraction.
>>> f1 = Fraction(3, 4) >>> f2 = Fraction(1, 8) >>> f1 + f2 Fraction(7, 8) >>> f1 = Fraction(1, 4) >>> f2 = Fraction(1, 2) >>> f1 + f2 Fraction(3, 4) """ new_num = (self._num * other_fraction._den + self._den * other_fraction._num) new_den = self._den * other_fraction._den common = gcd(new_num, new_den) return Fraction(new_num // common, new_den // common)
def __sub__(self, other_fraction): """Return the difference of self and other_fraction; that is, the Fraction that is obtained by subtracting other_fraction from sub.
>>> f1 = Fraction(3, 4) >>> f2 = Fraction(1, 8) >>> f1 - f2 Fraction(5, 8) >>> f1 = Fraction(1, 4) >>> f2 = Fraction(1, 2) >>> f1 - f2 Fraction(-1, 4) """ raise NotImplementedError("__sub__ hasn't been implemented.")
def __mul__(self, other_fraction): """Return the product of self and other_fraction; that is, self - other_fraction.
>>> f1 = Fraction(3, 4) >>> f2 = Fraction(1, 8) >>> f1 * f2 Fraction(3, 32) >>> f2 = Fraction(6, 8) >>> f1 * f2 Fraction(9, 16) """ raise NotImplementedError("__mul__ hasn't been implemented.")
def __truediv__(self, other_fraction): """Return the result of dividing self by other_fraction.
>>> f1 = Fraction(3, 4) >>> f2 = Fraction(1, 8) >>> f1 / f2 Fraction(6, 1) >>> f2 = Fraction(2, 1) >>> f1 * f2 Fraction(3, 8) """ raise NotImplementedError("__truediv__ hasn't been implemented.")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
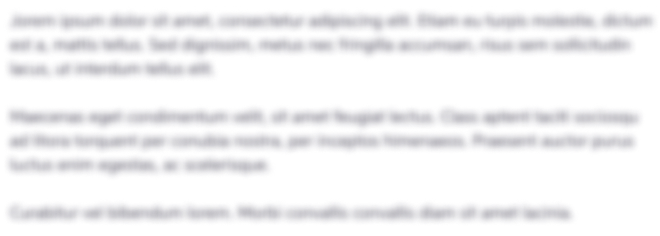
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started