Question
Solve this in Python please import random class Bag: def __init__(self): Initialize an empty bag. >>> bag = Bag() >>> bag {} raise NotImplementedError(__init__
Solve this in Python please
import random
class Bag:
def __init__(self): """Initialize an empty bag.
>>> bag = Bag() >>> bag {} """ raise NotImplementedError("__init__ hasn't been implemented.")
def __str__(self) -> str: """Return a string representation of this bag.
>>> bag = Bag() >>> for x in ['a', 'b', 'c', 'd', 'c', 'b', 'a']: ... bag.add(x) ... >>> str(bag) "{'a': 2, 'b': 2, 'c': 2, 'd': 1}"
# Note: the order in which the items are listed may be different # from what is shown in this example. """ return str(self._items)
def __repr__(self) -> str: """Return a string representation of this bag. This string is identical to the one returned by __str__.
>>> bag = Bag() >>> for x in ['a', 'b', 'c', 'd', 'c', 'b', 'a']: ... bag.add(x) .... >>> repr(bag) "{'a': 2, 'b': 2, 'c': 2, 'd': 1}" >>> bag {'a': 2, 'b': 2, 'c': 2, 'd': 1}
# Note: the order in which the items are listed may be different # from what is shown in these examples. """ raise NotImplementedError("__repr__ hasn't been implemented.")
def __iter__(self): """Return an iterator for this bag.
>>> bag = Bag() >>> for x in ['a', 'b', 'c', 'd', 'c', 'b', 'a']: ... bag.add(x) ... >>> for x in bag: ... print(x) ... ('a', 2) # bag has two a's ('b', 2) # bag has two b's ('c', 2) # bag has two c's ('d', 1) # bag has one d """ # The iterator returned by this method produces a sequence of 2-tuples. # The first element in each tuple is an item in the bag, and the second # element is the number of occurrences of that item. return iter(self._items.items())
def add(self, item: str) -> None: """Add item to this bag.
>>> bag = Bag() >>> for x in ['c', 'a', 'b', 'c', 'd']: ... bag.add(x) ... >>> bag {'c': 2, 'a': 1, 'b': 1, 'd': 1}
# Note: the order in which the items are listed may be different # from what is shown in this example. """ raise NotImplementedError("add hasn't been implemented.")
def __len__(self) -> int: """Return the number of items in this bag.
>>> bag = Bag() >>> len(bag) 0 >>> for x in ['a', 'b', 'c', 'd', 'c', 'b', 'a']: ... bag.add(x) ... >>> len(bag) 7 """ raise NotImplementedError("__len__ hasn't been implemented.")
def __contains__(self, item: str) -> bool: """Return True if item is in the bag.
>>> bag = Bag() >>> for x in ['a', 'b', 'c', 'd']: ... bag.add(x) ... >>> 'b' in bag True >>> 'k' in bag False """ raise NotImplementedError("__contains__ hasn't been implemented.")
def count(self, item: str) -> int: """Return the total number of occurrences of item in this bag.
>>> bag = Bag() >>> for x in ['c', 'a', 'b', 'c', 'd']: ... bag.add(x) ... >>> bag {'c': 2, 'a': 1, 'b': 1, 'd': 1}
>>> bag.count('c') 2 >>> bag.count('k') 0 """ raise NotImplementedError("count hasn't been implemented.")
def remove(self, item: str) -> str: """Remove and return one instance of item from this bag.
Raises KeyError if the bag is empty. Raises ValueError if item is not in the bag.
>>> bag = Bag() >>> for x in ['c', 'a', 'b', 'c', 'd']: ... bag.add(x) ... >>> bag {'c': 2, 'a': 1, 'b': 1, 'd': 1}
>>> bag.remove('c') 'c' >>> bag {'c': 1, 'a': 1, 'b': 1, 'd': 1}
>>> bag.remove('c') 'c' >>> bag {'a': 1, 'b': 1, 'd': 1}
# Note: the order in which the items are listed may be different # from what is shown in these examples. """ raise NotImplementedError("remove hasn't been implemented.")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
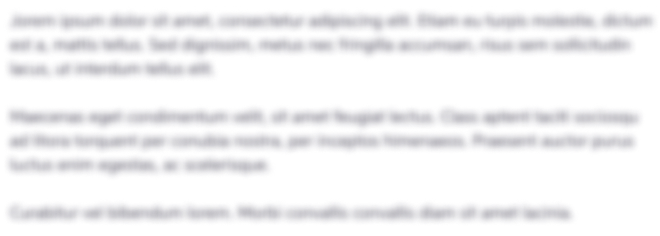
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started