Question
''' SortCount.py ''' from random import randint class SortCount: ''' Part 1 ''' def removeDups(self, alist): uniques = 0 i = 0 while i <
''' SortCount.py ''' from random import randint
class SortCount: ''' Part 1 ''' def removeDups(self, alist): uniques = 0 i = 0 while i < (len(alist) - 1): if alist[i] == alist[i+1]: alist.remove(alist[i]) #alist.append(0) else: i = i + 1 #Add 0s ot the end of the list alist += [0] * 3 return ''' Part 2 ''' ''' findMedian - "wrapper" method for your recursive median-finding method. It just makes the initial call to that method, passing it whatever initial parameters make sense. ''' def findMedian(alist): SortCount.findMedianHelper(alist,0,len(alist)-1) def findMedianHelper(alist,first,last): if first < last: splitpoint = partition(alist,first,last) SortCount.findMedianHelper(alist,first,splitpoint-1) SortCount.findMedianHelper(alist,splitpoint+1,last) # Remove the pass command and add your "wrapper method" here. # Put the definition of your recursive median-finding method below. ''' Part 3 '''
# the integers in the test arrays are drawn from the range 0, ..., MAX_VAL MAX_VAL = 65536 compares = 0 # total number of comparisons moves = 0 # total number of moves
''' compare - a wrapper that allows us to count comparisons. ''' def compare(self, comparison): compares = compares + 1 return comparison
''' move - moves an element of the specified array to a different location in the array. move(arr, dest, source) is equivalent to arr[dest] = arr[source]. Using this method allows us to count the number of moves that occur. ''' def move(self, alist, dest, source): moves = moves + 1 alist[dest] = alist[source];
''' swap - swap the values of two variables. Used by several of the sorting algorithms below. ''' def swap(self, alist, a, b): alist[a], alist[b] = alist[b], alist[a] moves = moves + 3
''' randomArray - creates an array of randomly generated integers with the specified number of elements and the specified maximum value ''' def randomArray(n, maxVal): alist = [] for i in range(len(alist)): alist.append(random.randint(0, maxVal+1)) return alist """ def randomArray(self, n): return randomArray(n, MAX_VAL) """ ''' almostSortedArray - creates an almost sorted array of integers with the specified number of elements ''' def almostSortedArray(self, n): # Produce a random list and sort it.
alist = randomArray(n) quickSort(alist) # Move one quarter of the elements out of place by between 1 and 5 places.
for i in range(n // 8): j = int((random.random() * n)) displace = -5 + int((random.random() * 11)) k = j + displace if k < 0: k = 0 if k > n - 1: k = n - 1; swap(arr, j, k) return arr # Prints the current counts of moves and comparisons. def printStats(): if compares == 0: spaces = 0 else: spaces = int((math.log(compares)/math.log(10))) for i in range(10 - spaces): print(" ") print(compares + " comparisons\t")
if moves == 0: spaces = 0 else: spaces = int((math.log(moves)/math.log(10))) for i in range(10 - spaces): print(" ") print(moves + " moves")
# quickSort def quickSort(self, alist): quickSortHelper(alist,0,len(alist)-1)
def quickSortHelper(self, alist,first,last): if first < last: splitpoint = partition(alist,first,last) quickSortHelper(alist,first,splitpoint-1) quickSortHelper(alist,splitpoint+1,last)
def partition(self,alist,first,last): pivotvalue = alist[first] moves = moves + 1 leftmark = first+1 rightmark = last
done = False while not done:
while compare(leftmark <= rightmark and alist[leftmark] <= pivotvalue): leftmark = leftmark + 1
while compare(alist[rightmark] >= pivotvalue and rightmark >= leftmark): rightmark = rightmark -1
if rightmark < leftmark: done = True else: swap(alist, leftmark, rightmark) swap(alist, first, rightmark) temp = alist[first] alist[first] = alist[rightmark] alist[rightmark] = temp return rightmark def findMedian(alist): if len(alist) % 2 == 0: Evenmedian = (alist[int(len(alist) / 2)] + alist[int(len(alist) / 2) +1]) / 2 print( "The median value for the even was: " , Evenmedian); else: Oddmedian = alist[int(len(alist) / 2)]; print("the median value for the odd number was: " , Oddmedian); alist = [54,26, 93,17,77,31,44,55,20] quickSort(alist) print(alist) findMedian(alist)
# Prints the specified list in the following form: [ arr[0] arr[1] ... ] def printArray(self, alist): # Don't print it if it's more than 10 elements. if length(alist) > 10: return print("[ ") for i in alist: print(i + " ") print("]") def Swapsort (list): for num in range(len(list)-1,0,-1): for i in range(num): if list[i]>list[i+1]: temp = list[i] list[i] = list[i+1] list[i+1] = temp list = [ 15 , 8, 20,5,12] Swapsort(list) print(list)
def main(): #Instatiate class object s = SortCount()
''' Part 1. Add your test cases for the removeDups() method here ''' test1 = [2,5,5,5,10,12,12] s.removeDups(test1) print(test1)
''' Part 2. Add your test cases for the findMedian() method here ''' import statistics #findMedian(alist) # the median of this array is 15 oddLength = [4, 18, 12, 34, 7, 42, 15, 22, 5] # the median of this array is the average of 15 and 18 = 16.5 evenLength = [4, 18, 12, 34, 7, 42, 15, 22, 5, 27] xOdd = statistics.median(oddLength) yEven = statistics.median(evenLength)
print(xOdd) print(yEven) alist = [] # Put code to test your method here. count = 1000 for i in range(10): count = 2^i*count for n in range(count): alist.append(randint(2, 99)) #analysis code
''' Part 3. ''' numItems = 5 a = numItems * [0] # the array asave = numItems * [0] # a copy of the original unsorted array
# Get various parameters from the user.
print() numItems = int(input(("How many items in the array? "))) arrayType = input("Random (r), almost sorted (a), or fully sorted (f)? ") print() # Create the if arrayType == "a": if arrayType == "a": a = SortCount.almostSortedArray(numItems) else: a = SortCount.randomArray(numItems) if arrayType == "f": SortCount.quickSort(a)
asave = copy.copy(a) printArray(a)
print("swapSort") # initStats() swapSort(a) printStats() printArray(a) #if __name__ == "__main__": main() # print .findMedianSortedArrays([4, 18, 12, 34], [7, 42, 15, 22, 5])
I have an error on this line
''' Sameh Fazli Comsc 132 SortCount.py ''' from random import randint
class SortCount: ''' Part 1 ''' def removeDups(self, alist): uniques = 0 i = 0 while i < (len(alist) - 1): if alist[i] == alist[i+1]: alist.remove(alist[i]) #alist.append(0) else: i = i + 1 #Add 0s ot the end of the list alist += [0] * 3 return ''' Part 2 ''' ''' findMedian - "wrapper" method for your recursive median-finding method. It just makes the initial call to that method, passing it whatever initial parameters make sense. ''' def findMedian(alist): SortCount.findMedianHelper(alist,0,len(alist)-1) def findMedianHelper(alist,first,last): if first < last: splitpoint = partition(alist,first,last) SortCount.findMedianHelper(alist,first,splitpoint-1) SortCount.findMedianHelper(alist,splitpoint+1,last) # Remove the pass command and add your "wrapper method" here. # Put the definition of your recursive median-finding method below. ''' Part 3 '''
# the integers in the test arrays are drawn from the range 0, ..., MAX_VAL MAX_VAL = 65536 compares = 0 # total number of comparisons moves = 0 # total number of moves
''' compare - a wrapper that allows us to count comparisons. ''' def compare(self, comparison): compares = compares + 1 return comparison
''' move - moves an element of the specified array to a different location in the array. move(arr, dest, source) is equivalent to arr[dest] = arr[source]. Using this method allows us to count the number of moves that occur. ''' def move(self, alist, dest, source): moves = moves + 1 alist[dest] = alist[source];
''' swap - swap the values of two variables. Used by several of the sorting algorithms below. ''' def swap(self, alist, a, b): alist[a], alist[b] = alist[b], alist[a] moves = moves + 3
''' randomArray - creates an array of randomly generated integers with the specified number of elements and the specified maximum value ''' def randomArray(n, maxVal): alist = [] for i in range(len(alist)): alist.append(random.randint(0, maxVal+1)) return alist """ def randomArray(self, n): return randomArray(n, MAX_VAL) """ ''' almostSortedArray - creates an almost sorted array of integers with the specified number of elements ''' def almostSortedArray(self, n): # Produce a random list and sort it.
alist = randomArray(n) quickSort(alist) # Move one quarter of the elements out of place by between 1 and 5 places.
for i in range(n // 8): j = int((random.random() * n)) displace = -5 + int((random.random() * 11)) k = j + displace if k < 0: k = 0 if k > n - 1: k = n - 1; swap(arr, j, k) return arr # Prints the current counts of moves and comparisons. def printStats(): if compares == 0: spaces = 0 else: spaces = int((math.log(compares)/math.log(10))) for i in range(10 - spaces): print(" ") print(compares + " comparisons\t")
if moves == 0: spaces = 0 else: spaces = int((math.log(moves)/math.log(10))) for i in range(10 - spaces): print(" ") print(moves + " moves")
# quickSort def quickSort(self, alist): quickSortHelper(alist,0,len(alist)-1)
def quickSortHelper(self, alist,first,last): if first < last: splitpoint = partition(alist,first,last) quickSortHelper(alist,first,splitpoint-1) quickSortHelper(alist,splitpoint+1,last)
def partition(self,alist,first,last): pivotvalue = alist[first] moves = moves + 1 leftmark = first+1 rightmark = last
done = False while not done:
while compare(leftmark <= rightmark and alist[leftmark] <= pivotvalue): leftmark = leftmark + 1
while compare(alist[rightmark] >= pivotvalue and rightmark >= leftmark): rightmark = rightmark -1
if rightmark < leftmark: done = True else: swap(alist, leftmark, rightmark) swap(alist, first, rightmark) temp = alist[first] alist[first] = alist[rightmark] alist[rightmark] = temp return rightmark def findMedian(alist): if len(alist) % 2 == 0: Evenmedian = (alist[int(len(alist) / 2)] + alist[int(len(alist) / 2) +1]) / 2 print( "The median value for the even was: " , Evenmedian); else: Oddmedian = alist[int(len(alist) / 2)]; print("the median value for the odd number was: " , Oddmedian); alist = [54,26, 93,17,77,31,44,55,20] quickSort(alist) print(alist) findMedian(alist)
# Prints the specified list in the following form: [ arr[0] arr[1] ... ] def printArray(self, alist): # Don't print it if it's more than 10 elements. if length(alist) > 10: return print("[ ") for i in alist: print(i + " ") print("]") def Swapsort (list): for num in range(len(list)-1,0,-1): for i in range(num): if list[i]>list[i+1]: temp = list[i] list[i] = list[i+1] list[i+1] = temp list = [ 15 , 8, 20,5,12] Swapsort(list) print(list)
def main(): #Instatiate class object s = SortCount()
''' Part 1. Add your test cases for the removeDups() method here ''' test1 = [2,5,5,5,10,12,12] s.removeDups(test1) print(test1)
''' Part 2. Add your test cases for the findMedian() method here ''' import statistics #findMedian(alist) # the median of this array is 15 oddLength = [4, 18, 12, 34, 7, 42, 15, 22, 5] # the median of this array is the average of 15 and 18 = 16.5 evenLength = [4, 18, 12, 34, 7, 42, 15, 22, 5, 27] xOdd = statistics.median(oddLength) yEven = statistics.median(evenLength)
print(xOdd) print(yEven) alist = [] # Put code to test your method here. count = 1000 for i in range(10): count = 2^i*count for n in range(count): alist.append(randint(2, 99)) #analysis code
''' Part 3. ''' numItems = 5 a = numItems * [0] # the array asave = numItems * [0] # a copy of the original unsorted array
# Get various parameters from the user.
print() numItems = int(input(("How many items in the array? "))) arrayType = input("Random (r), almost sorted (a), or fully sorted (f)? ") print() # Create the if arrayType == "a": if arrayType == "a": a = SortCount.almostSortedArray(numItems) else: a = SortCount.randomArray(numItems) if arrayType == "f": SortCount.quickSort(a)
asave = copy.copy(a) printArray(a)
print("swapSort") # initStats() swapSort(a) printStats() printArray(a) #if __name__ == "__main__": main() # print .findMedianSortedArrays([4, 18, 12, 34], [7, 42, 15, 22, 5])
I have an error on this line 263, in main a = SortCount.randomArray(numItems) TypeError: randomArray() missing 1 required positional argument: 'maxVal' and i need help completing my swapsort. Currently I have finished the Find The median and Remove the Duplicates now i need the swap sort.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
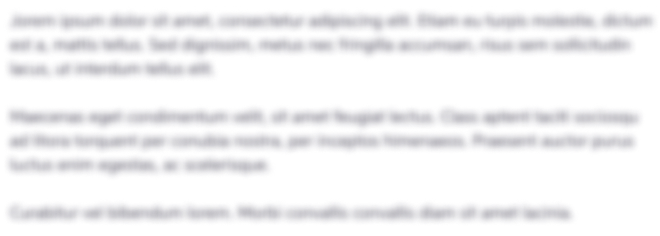
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started