Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Source code and pictures below for implementations and requirements. Please help I will upvote without hesitation. import java.util.Scanner; /** * This program performs numerical calculations
Source code and pictures below for implementations and requirements. Please help I will upvote without hesitation.Your job for this project is to provide the implementation for the Number class. You can find the documentation for its public methods here. Requirements and restrictions - The class has to use a linked list to represent the object. In fact, Number is a linked list. You can implement it as a singly linked list of nodes that contain single digits. You can use dummy nodes at the start and end of the list, if you wish (but this is not something we discussed in class). - The class should have an internal private Node class. - You are not allowed to use any kind of List implementation provided by Java libraries. - You are not allowed to use the BigInteger class provided by Java Libraries. - All manipulations required to implement addition, multiplication and comparisons have to be performed on nodes of the list. (You cannot convert the objects to their integer or string equivalents and perform the operations on int, Integer or on characters of a string.) Any kind of numerical computations have to be performed on single digits only (although in some cases the result may have more than one digit). In order to implement the addition and multiplication you should recall the methods of performing these operations by hand (or long hand operations). If you do not remember how to do this, here are some tutorials: - Long Hand Addition - Long Hand Multiplication (There are many others you can find online.) Hint: the function multiplyBydigit ( ) in the Number class may come in handy in implementing the general multiply operation. DATA STORAGE AND ORGANIZATION You do not need to implement any other classes, but you may if you wish. PROGRAMMING RULES - You should follow the rules outlined in the document code conventions posted on the course website. - You may not use any of the collection classes provided in Java libraries. Do not use LinkedList, stack, Queue, or any classes implementing the List or queue interfaces). - You may not use the Biginteger class. - You may use any exception-related classes. Sample Run of The Program 123+987 1110 1231111111111111111111111111111111111111 136666666666666666666666666666666666653 1111111111111111111111111111111111+99999999999999999999999999999999999999999999999999999 100000000000000011111111111111111111111111111111110 123=9876 false 123==123 true 123123 false 3333333333333333333333311 366666666666666666663 33333333333333333333311 Illegal expression found: 3333333333333333333333311 7+3 Illegal expression found: 7+3 1000000000000000 - 999999999999999 Illegal operator found: 10000000000000009999999999999999 hunisis Almphareriesinkricces: Derpuzenduchumear'a v.le elasa Mrebez e: ide tifimi. 4.44 ix rzasalsti as a Lins: ruke lid: Paiatstera: auzer-a slrine erpenaline if tis auabe This: Bulleunteknoz-ien- if auxe is ndl![]()
import java.util.Scanner; /** *
This program performs numerical calculations based on user's input. * An operation needs to be provided using a three-token format: * operand1 operator operand2 * in which the three tokens are separated by one of more white spaces. * The program terminates when the end of file (EOF) character is found. * (Note, this character is entered in the console by typing Ctrl+D sequence.) * *
Valid operands are any positive integers (no length limit). * *
Valid operators are: *
* + addition * * multiplication * greater than * >= greater than or equal * == equal * not equal **/ public class LongNumbers { public static void main(String[] args) { Scanner in = new Scanner (System.in); String line = null; String[] tokens; Number n1=null, n2=null, n3=null; while (in.hasNextLine() ) { //read input from user and break it into expected three tokens: // operand1 operator operand2 line = in.nextLine(); tokens = line.split("\\s+"); try { //get two operands n1 = new Number(tokens[0]); n2 = new Number(tokens[2]); //perform the action of the operator switch (tokens[1]) { case "+": n3 = n1.add(n2); System.out.println(n3); break; case "*": n3 = n1.multiply(n2); System.out.println(n3); break; case "==": System.out.println(n1.equals(n2) ? "true" : "false"); break; case "": System.out.println(n1.compareTo(n2) > 0 ? "true" : "false"); break; case ">=": System.out.println(n1.compareTo(n2) >= 0 ? "true" : "false"); break; case "": System.out.println(n1.compareTo(n2) != 0 ? "true" : "false"); break; default: //deals with unsupported and illegal operators System.out.println("Illegal operator found: " + line); continue; } } catch (IllegalArgumentException ex) { //deals with illegal and misformatted requests System.out.println("Illegal expression found: " + line); continue; } catch (ArrayIndexOutOfBoundsException ex) { //deals with illegal and misformatted requests System.out.println("Illegal expression found: " + line); continue; } } in.close(); } //prevent class instantiation (otherwise compiler generates default constructor) private LongNumbers( ) { } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
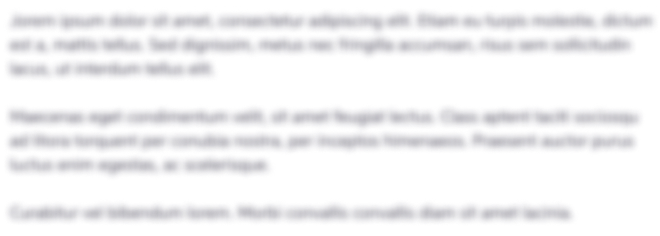
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started