Question
Source Code: Box.java public class Box > implements Comparable > { private T thing; private int thingCount; private T[] oldThings; public Box(T firstThing) { this.thing
Source Code:
Box.java
public class Box
private T thing;
private int thingCount;
private T[] oldThings;
public Box(T firstThing) {
this.thing = firstThing;
this.thingCount = 1;
//oldThings = new T[5]; // not allowed
oldThings = (T[]) (new Comparable[5]); // allowed
}
public T getContents() {
return thing;
}
public int getCount() {
return thingCount;
}
public void replaceContents(T newThing) {
this.thing = newThing;
thingCount++;
}
@Override
public String toString() {
return thing.toString() + " (item " + thingCount + ")";
}
@Override
public boolean equals(Object other) {
if(other instanceof Box>) {
Box> otherBox = (Box>) other;
boolean sameThing = this.thing.equals(otherBox.thing);
boolean sameCount = this.thingCount==otherBox.thingCount;
return sameThing && sameCount;
} else {
return false;
}
}
@Override
public int compareTo(Box
if(this.thing.compareTo(otherBox.thing)==0) {
return Integer.compare(this.thingCount, otherBox.thingCount);
} else {
return this.thing.compareTo(otherBox.thing);
}
}
}
--------------------------------
BoxR.java
public class BoxR {
private Object thing;
private int thingCount;
public BoxR(Object firstThing) {
this.thing = firstThing;
this.thingCount = 1;
}
public Object getContents() {
return thing;
}
public int getCount() {
return thingCount;
}
public void replaceContents(Object newThing) {
this.thing = newThing;
thingCount++;
}
@Override
public String toString() {
return thing.toString() + " (item " + thingCount + ")";
}
@Override
public boolean equals(Object other) {
if(other instanceof BoxR) {
BoxR otherBoxR = (BoxR) other;
boolean sameThing = this.thing.equals(otherBoxR.thing);
boolean sameCount = this.thingCount==otherBoxR.thingCount;
return sameThing && sameCount;
} else {
return false;
}
}
}
------------------------
BoxTester.java
import java.util.*;
public class BoxTester {
public static void main(String[] args) {
BoxR numbersBoxNotReally = new BoxR(new Integer(4));
numbersBoxNotReally.replaceContents(new Integer(5));
/umbersBoxNotReally.replaceContents(new String("hello"));
Integer currentContents = (Integer) numbersBoxNotReally.getContents();
Box
numbersBox1.replaceContents(new Integer(5));
Box
wordBox.replaceContents("bye");
Box
wordBox2.replaceContents("bye");
System.out.println("same boxes? " + wordBox.equals(wordBox2));
System.out.println("same boxes? " + wordBox.equals(numbersBox1));
Box
Box
numbersBox3.replaceContents(5);
numbersBox3.replaceContents(2);
Box
numbersBox4.replaceContents(12);
numbersBox4.replaceContents(5);
Box
ArrayList
listOfNumberBoxes.add(numbersBox1);
listOfNumberBoxes.add(numbersBox2);
listOfNumberBoxes.add(numbersBox3);
listOfNumberBoxes.add(numbersBox4);
listOfNumberBoxes.add(numbersBox5);
Collections.sort(listOfNumberBoxes);
System.out.println(listOfNumberBoxes);
}
}
----------------------
NameInput.java
import java.util.*;
public class NameInput {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
ArrayList
System.out.println("Enter names or \"quit\" to quit:");
String name = scan.nextLine();
while(!name.equalsIgnoreCase("quit")) {
nameList.add(name);
System.out.println("Enter names or \"quit\" to quit:");
name = scan.nextLine();
}
Collections.sort(nameList);
String firstName = nameList.get(0);
System.out.println(nameList);
}
-------------------
NumericInput.java
import java.util.*;
public class NumericInput {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
ArrayList
System.out.println("How many numbers?");
int numberNumbers = Integer.parseInt(scan.nextLine());
for(int i=0; i int userNumber = Integer.parseInt(scan.nextLine()); numberList.add(userNumber); } int total = 0; for(Integer i : numberList) { total += i; } double average = (total * 1.0) / ( (double) numberList.size() ); System.out.println("The average is " + average); } } ----------------- Student.java public class Student implements Comparable { private String firstName, lastName; private int studentID; public Student(String firstName, String lastName, int studentID) { this.firstName = firstName; this.lastName = lastName; this.studentID = studentID; } public String getFirstName() { return firstName; } public String getLastName() { return lastName; } public int getStudentID() { return studentID; } public void setFirstName(String firstName) { this.firstName = firstName; } public void setLastName(String lastName) { this.lastName = lastName; } public void setStudentID(int studentID) { if(studentID >=0 && studentID this.studentID = studentID; } } @Override public String toString() { return lastName + ", " + firstName + " (" + studentID + ")"; } @Override public boolean equals(Object other) { if(other instanceof Student) { Student otherStudent = (Student) other; return this.studentID == otherStudent.studentID; } else { return false; } } @Override public int compareTo(Object other) { if(other instanceof Student) { Student otherStudent = (Student) other; if(this.studentID return -1; } else if(this.studentID == otherStudent.studentID){ return 0; } else { assert this.studentID > otherStudent.studentID; return 1; } } else { return -99; } } /* @Override public int compareTo(Object other) { if(other instanceof Student) { Student otherStudent = (Student) other; if(this.lastName.equals(otherStudent.lastName)) { return this.firstName.compareTo(otherStudent.firstName); } else { return this.lastName.compareTo(otherStudent.lastName); } } else { return -99; } } */ }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
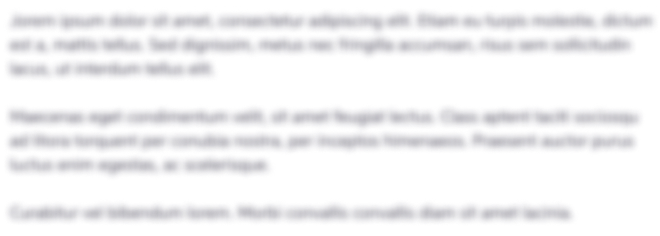
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started