Question
SOURCE CODE: public class Tunes { public static void main(String[] args) { // TODO Auto-generated method stub CDCollection music = new CDCollection (); music.addCD(Storm Front,
SOURCE CODE:
public class Tunes {
public static void main(String[] args) {
// TODO Auto-generated method stub
CDCollection music = new CDCollection ();
music.addCD("Storm Front", "Billy Joel", 14.95, 10);
music.addCD("Come On Over", "Shania Twain", 14.95, 16);
music.addCD("Soundtrack", "Les Miserables", 17.95, 33);
music.addCD("Graceland", "Paul Simon", 13.90, 11);
System.out.println(music);
music.addCD("Double Live", "Garth Brooks", 19.99, 26);
music.addCD("Greatest Hits", "Jimmy Buffet", 15.95, 13)
System.out.println(music);
}
import java.text.NumberFormat;
public class CDCollection
{
private CD[] collection;
private int count;
private double totalCost;
public CDCollection()
{
collection = new CD[100];
count = 0;
totalCost = 0.0;
}
public void addCD(String title, String artist, double cost,
int tracks)
{
if (count == collection.length)
increaseSize();
collection[count] = new CD(title, artist, cost, tracks);
totalCost += cost;
count++;
}
public String toString()
{
NumberFormat fmt = NumberFormat.getCurrencyInstance();
String report = "~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ ";
report += "My CD Collection ";
report += "Number of CDs: " + count + " ";
report += "Total cost: " + fmt.format(totalCost) + " ";
report += "Average cost: " + fmt.format(totalCost/count);
report += " CD List: ";
for (int cd = 0; cd < count; cd++)
report += collection[cd].toString() + " ";
return report;
}
private void increaseSize()
{
CD[] temp = new CD[collection.length * 2];
for (int cd = 0; cd < collection.length; cd++)
temp[cd] = collection[cd];
collection = temp;
}
public String getTitle()
{
return title;
}
import java.text.NumberFormat;
public class CD
{
private String title, artist;
private double cost;
private int tracks;
public CD(String name, String singer, double price, int numTracks)
{
title = name;
artist = singer;
cost = price;
tracks = numTracks;
}
public String toString()
{
NumberFormat fmt = NumberFormat.getCurrencyInstance();
String description;
description = fmt.format(cost) + "\t" + tracks + "\t";
description += title + "\t" + artist;
return description;
}
}
I need to make these modifications:
Arrays of objects are a difficult topic at first. In this lab, you will be changing code in programs discussed in Chapter Seven (Section 7.3 Arrays of Objects). I have provided snippet of code in some cases to simplify, but it is essentially the same. This is the example program starting with Listing 7-7 in book.
Use the 3 files attached to this lab for these changes.
Run Tunes.java to view the output.
(5 points)
Add two more cds of your choice to the collection. Print out again.
----------------------------------------------------------------------------------------------------------------------------------
Create a int variable index in Tunes.java. This value will be used to get one title from the collection.
------------------------------------------------------------------------------
Add this code to CD.java (a getter):
(2 points)
public String getTitle()
{
return title;
}
------------------------------------------------------------------------------
Add this code to CDCollection.java:
(2 points)
public String findTitle (int i)
{
return (collection[i].getTitle());
}
-----------------------------------------------------------------------------
Add this code to Tunes.java:
(10 points)
Using a loop to allow user to enter 0 thru number of CDs Added to select and list/display a Title of CD in the list. (example below is using the number entered called index)
If value entered is valid then do this line of code System.out.println(music.findTitle(index));
Index is used as the value entered, if value entered not valid try again. Allow loop to end!
If the value = 1, then Come on Over will displayed.
--------------------------------------------------------------------------------------------------------------------------------
(15 points)
Add the code to add up the costs in the collection and display the value. Yes, there is already a totalCost but do this for 15 points. All three java classes need code added, code not completely given like above so THINK!
Place this code in a for loop in CDCollection.java and add the necessary code to the other two java files shown below. This will be similar to what you did in bullet 2 above. Also look at the for loop in toString()
total = total + (collection[cd].getCost());
In Tunes.java will ask for the total and print the total at end of program Total cost for collection:
In CD.java will give the the cost hence [hint:] getCost()
--------------------------------------------------------------------------------------------------------------------------------
(6 points)
(3 pts) Write a method to find most expensive CD.
(3 pts) Write a method to find CD with most tracks
Print out results.
(10 points)
Modify current output and add prints. Program works!
-------------------------------------
My CD Collection
Number of CDs: 4
Total Cost: $61.75
Average Cost: $15.44
CD Listing:
$14.95 10 Storm Front Billy Joel
$14.95 16 Come on Over Shania Twain
$17.95 33 SoundTrack Les Miserables
$13.90 11 GraceLand Paul Simon
-------------------------------------
My CD Collection
Number of CDs: 6
Total Cost: $97.69
Average Cost: $16.28
CD Listing:
$14.95 10 Storm Front Billy Joel
$14.95 16 Come on Over Shania Twain
$17.95 33 SoundTrack Les Miserables
$13.90 11 GraceLand Paul Simon
$19.99 26 Double Live Garth Brooks
$15.95 13 Greatest Hits Jimmy Buffet
-------------------------------------
My CD Collection
Number of CDs: 8
Total Cost: $134.59
Average Cost: $16.82
CD Listing:
$14.95 10 Storm Front Billy Joel
$14.95 16 Come on Over Shania Twain
$17.95 33 SoundTrack Les Miserables
$13.90 11 GraceLand Paul Simon
$19.99 26 Double Live Garth Brooks
$15.95 13 Greatest Hits Jimmy Buffet
$16.95 10 Divide Ed Sheeran
$19.95 12 Reputation Taylor Swift
enter number (-1 to quit) :
9
not valid, try again
enter number (-1 to quit) :
5
Greatest Hits
enter number (-1 to quit) :
3
GraceLand
enter number (-1 to quit) :
2
SoundTrack
enter number (-1 to quit) :
2
SoundTrack
enter number (-1 to quit) :
-1
Total cost for collection is: 134.59
Most expensive cd: Double
CD with most tracks: SoundTrack
The end folks!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
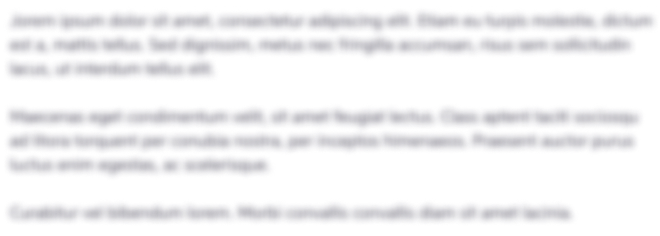
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started