Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Specification Write a program that enables a user to play number guessing games. The following is a nutshell description of a number guessing game. +
Specification
Write a program that enables a user to play number guessing games. The following is a nutshell description of a number guessing game.
+ a random number is generated
+ loop prompting the user to enter guesses
until the user guesses the number or hits
the maximum number of allowed guesses or
enters the "quit" sentinel value
The rest of this specification documents number guessing games in more detail. The documentation uses manifest constants that can be used by your program.
Playing a Guessing Game
Use a class Random object to get a random number between MIN_NUMBER (1) and MAX_NUMBER (205), inclusive.
Print the ENTER_GUESS_PROMPT and read the user input. Loop until one of the following are true.
+ the user enters the random number
- print WINNER_MSG along with number of guesses
+ the user enters MAX_GUESSES (10) wrong guesses
- print LOSER_MSG along with the random number
+ the user enters QUIT_VALUE (-1)
- print QUITTER_MSG
The current game ends in all three of these cases.
(see "End of Game Processing" section)
If the user enters BACKDOOR_VALUE (-314), print the random number and re-prompt the user to enter a guess. BACKDOOR_VALUE input is not counted as a wrong guess.
Print a INPUT_TOO_SMALL_MSG or INPUT_TOO_LARGE_MSG message when the user enters a number that is either less than MIN_NUMBER or greater than MAX_NUMBER, respectively. Re-prompt the user to enter a guess after printing the message. Invalid inputs are not counted as wrong guesses.
Print a NOPE_NOPE_MSG message when the user enters a wrong guess more than once and re-prompt the user to enter a guess. Duplicate wrong guesses are not counted as wrong guess.
Print a NOPE_MSG when the user enters a wrong guess. At HINT_THRESHOLD (5) wrong guesses, print a HIGHER_MSG or LOWER_MSG message. Re-prompt the user to enter a guess.
End of Game Processing
If number of games played equals MAX_GAMES (4), then do "Post Game Playing Processing." Otherwise, issue the PLAY_AGAIN_PROMPT. Do "Post Game Playing Processing" if user enters 'n', else start new game.
Post Game Playing Processing
Print the following prior to exiting the program.
number of games played
number of games won
number of games lost
number of games quit
winning percentage
Also print game summaries for each game played.
games played: 3; won: 1; lost: 1; quit: 1; winning pct.: 33.33%
game 1: Won; the number was: 145; #guesses: 6; backdoored: true
...guesses in ascending order: 1,2,3,4,5,
game 2: Quit; the number was: 49; #guesses: 3; backdoored: false
...guesses in ascending order: 1,2,3,
game 3: Lost; the number was: 182; #guesses: 10; backdoored: true
...guesses in ascending order: 1,5,144,150,181,183,191,199,200,201,
Manifest Constants
Your program can implements I_GG to use the manifest constants defined in the interface. I_GG can be copied/pasted after (or before) the declaration of your class.
To Support Testing (i.e. test mode)
If the program is executed with a command-line argument, then the random number for each game is DFLT_NUMBER (60).
The motivation for supporting test mode is to play games without having to play games.
shell-prompt: cat gg.in
-314 10 20 30 foo 40 50 70 60 y
10 20 10 0 210 -1 y
1 2 3 4 5 6 7 8 9 10 11 12 y
1 2 3 1 2 60 w y
shell-prompt: cat gg.in | java GG testing > gg.out
Example Games
*** Hello! Have fun playing the CSC205AA guessing game. ***
enter a guess between 1 and 205 (-1 to quit): 1 // ENTER_GUESS_PROMPT
nope... // NOPE_MSG
enter a guess between 1 and 205 (-1 to quit): 2
nope...
enter a guess between 1 and 205 (-1 to quit): 3
nope...
enter a guess between 1 and 205 (-1 to quit): 4
nope...
enter a guess between 1 and 205 (-1 to quit): 5
nope... higher // NOPE_MSG with hint
enter a guess between 1 and 205 (-1 to quit): 3
you've already guessed that wrong guess... // NOPE_NOPE_MSG
enter a guess between 1 and 205 (-1 to quit): 314
*** invalid input -- must be less than 206 // INPUT_TOO_LARGE_MSG
enter a guess between 1 and 205 (-1 to quit): -5
*** invalid input -- must be greater than 0 // INPUT_TOO_SMALL_MSG
enter a guess between 1 and 205 (-1 to quit): -314
...the number is 145
enter a guess between 1 and 205 (-1 to quit): 145
you're a winner... # of guesses: 6 // WINNER_MSG
Do you want to play again (n or y)? y // PLAY_AGAIN_PROMPT
enter a guess between 1 and 205 (-1 to quit): 1
nope...
enter a guess between 1 and 205 (-1 to quit): 2
nope...
enter a guess between 1 and 205 (-1 to quit): 3
nope...
enter a guess between 1 and 205 (-1 to quit): -1
you're a quitter... the number was 49 // QUITTER_MSG
Do you want to play again (n or y)? what
*** invalid input -- must be n or y // NOT_YN_MSG
Do you want to play again (n or y)? y
enter a guess between 1 and 205 (-1 to quit): -314
...the number is 182
enter a guess between 1 and 205 (-1 to quit): 150
nope...
enter a guess between 1 and 205 (-1 to quit): 200
nope...
enter a guess between 1 and 205 (-1 to quit): 1
nope...
enter a guess between 1 and 205 (-1 to quit): 5
nope...
enter a guess between 1 and 205 (-1 to quit): 200
you've already guessed that wrong guess...
enter a guess between 1 and 205 (-1 to quit): 206
*** invalid input -- must be less than 206
enter a guess between 1 and 205 (-1 to quit): 0
*** invalid input -- must be greater than 0
enter a guess between 1 and 205 (-1 to quit): 144
nope... higher
enter a guess between 1 and 205 (-1 to quit): foo
*** invalid input -- must be an whole number
enter a guess between 1 and 205 (-1 to quit): 201
nope... lower
enter a guess between 1 and 205 (-1 to quit): 183
nope... lower
enter a guess between 1 and 205 (-1 to quit): 200
you've already guessed that wrong guess...
enter a guess between 1 and 205 (-1 to quit): 199
nope... lower
enter a guess between 1 and 205 (-1 to quit): -314
...the number is 182
enter a guess between 1 and 205 (-1 to quit): 181
nope... higher
enter a guess between 1 and 205 (-1 to quit): 191
too many guesses entered... the number was 182 // LOSER_MSG
Do you want to play again (n or y)? n
*** Thanks for playing the CSC205AA guessing game. ***
games played: 3; won: 1; lost: 1; quit: 1; winning pct.: 33.33%
game 1: Won; the number was: 145; #guesses: 6; backdoored: true
...guesses in ascending order: 1,2,3,4,5,
game 2: Quit; the number was: 49; #guesses: 3; backdoored: false
...guesses in ascending order: 1,2,3,
game 3: Lost; the number was: 182; #guesses: 10; backdoored: true
...guesses in ascending order: 1,5,144,150,181,183,191,199,200,201,
Example Games (second round to test MAX_GAMES played)
*** Hello! Have fun playing the CSC205AA guessing game. ***
enter a guess between 1 and 205 (-1 to quit): -1
you're a quitter... the number was 157
Do you want to play again (n or y)? y
enter a guess between 1 and 205 (-1 to quit): -1
you're a quitter... the number was 4
Do you want to play again (n or y)? y
enter a guess between 1 and 205 (-1 to quit): -314
...the number is 35
enter a guess between 1 and 205 (-1 to quit): 35
you're a winner... # of guesses: 1
Do you want to play again (n or y)? y
enter a guess between 1 and 205 (-1 to quit): -1
you're a quitter... the number was 118
Maximum number (4) of games have been played.
*** Thanks for playing the CSC205AA guessing game. ***
games played: 4; won: 1; lost: 0; quit: 3; winning pct.: 25.00%
game 1: Quit; the number was: 157; #guesses: 0; backdoored: false
game 2: Quit; the number was: 4; #guesses: 0; backdoored: false
game 3: Won; the number was: 35; #guesses: 1; backdoored: true
game 4: Quit; the number was: 118; #guesses: 0; backdoored: false
Example Games (test mode)
*** Hello! Have fun playing the CSC205AA guessing game. ***
enter a guess between 1 and 205 (-1 to quit): -314
...the number is 60
enter a guess between 1 and 205 (-1 to quit): 10
nope...
enter a guess between 1 and 205 (-1 to quit): 20
nope...
enter a guess between 1 and 205 (-1 to quit): 30
nope...
enter a guess between 1 and 205 (-1 to quit): foo
*** invalid input -- must be an whole number
enter a guess between 1 and 205 (-1 to quit): 40
nope...
enter a guess between 1 and 205 (-1 to quit): 50
nope... higher
enter a guess between 1 and 205 (-1 to quit): 70
nope... lower
enter a guess between 1 and 205 (-1 to quit): 60
you're a winner... # of guesses: 7
Do you want to play again (n or y)? y
enter a guess between 1 and 205 (-1 to quit): 10
nope...
enter a guess between 1 and 205 (-1 to quit): 20
nope...
enter a guess between 1 and 205 (-1 to quit): 10
you've already guessed that wrong guess...
enter a guess between 1 and 205 (-1 to quit): 0
*** invalid input -- must be greater than 0
enter a guess between 1 and 205 (-1 to quit): 210
*** invalid input -- must be less than 206
enter a guess between 1 and 205 (-1 to quit): -1
you're a quitter... the number was 60
Do you want to play again (n or y)? y
enter a guess between 1 and 205 (-1 to quit): 1
nope...
enter a guess between 1 and 205 (-1 to quit): 2
nope...
enter a guess between 1 and 205 (-1 to quit): 3
nope...
enter a guess between 1 and 205 (-1 to quit): 4
nope...
enter a guess between 1 and 205 (-1 to quit): 5
nope... higher
enter a guess between 1 and 205 (-1 to quit): 6
nope... higher
enter a guess between 1 and 205 (-1 to quit): 7
nope... higher
enter a guess between 1 and 205 (-1 to quit): 8
nope... higher
enter a guess between 1 and 205 (-1 to quit): 9
nope... higher
enter a guess between 1 and 205 (-1 to quit): 10
too many guesses entered... the number was 60
Do you want to play again (n or y)? 11
*** invalid input -- must be n or y
Do you want to play again (n or y)? 12
*** invalid input -- must be n or y
Do you want to play again (n or y)? y
enter a guess between 1 and 205 (-1 to quit): 1
nope...
enter a guess between 1 and 205 (-1 to quit): 2
nope...
enter a guess between 1 and 205 (-1 to quit): 3
nope...
enter a guess between 1 and 205 (-1 to quit): 1
you've already guessed that wrong guess...
enter a guess between 1 and 205 (-1 to quit): 2
you've already guessed that wrong guess...
enter a guess between 1 and 205 (-1 to quit): 60
you're a winner... # of guesses: 4
Maximum number (4) of games played.
*** Thanks for playing the CSC205AA guessing game. ***
games played: 4; won: 2; lost: 1; quit: 1; winning pct.: 50.00%
game 1: Won; the number was: 60; #guesses: 7; backdoored: true
...guesses in ascending order: 10,20,30,40,50,70,
game 2: Quit; the number was: 60; #guesses: 2; backdoored: false
...guesses in ascending order: 10,20,
game 3: Lost; the number was: 60; #guesses: 10; backdoored: false
...guesses in ascending order: 1,2,3,4,5,6,7,8,9,10,
game 4: Won; the number was: 60; #guesses: 4; backdoored: false
...guesses in ascending order: 1,2,3,










Step by Step Solution
There are 3 Steps involved in it
Step: 1
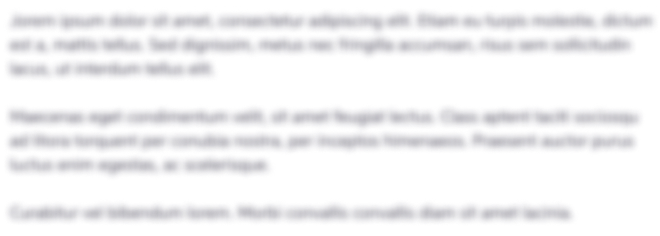
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started