Question
Spell Checking (12 Points). Written Hawaiian also has fairly simple spelling rules for determining if a word is a valid word in the language (even
Spell Checking (12 Points).
Written Hawaiian also has fairly simple spelling rules for determining if a word is a valid word in the language (even if the meaning is unknown). They are:
All words contain only vowels and Hawaiian consonants.
All words end in a vowel.
Within a word, two consonants NEVER appear adjacent.
Write a program which reads lines of Hawaiian text from a file (using redirection, so you will not need to prompt), and lists each word found on a separate line indicating whether it is a valid Hawaiian spelling or it is invalid. Any non-letter characters (e.g. white space or punctuation characters) in the file should be treated as delimiters, but otherwise ignored and not appear in the output.
You should think about your algorithm before beginning to code this function, and you might want to look at the program wds.c from lecture (and on wiliki in ~ee160/Code.lect/Chars/wds.c) for guidance. (That file is similar, but better, than the code in Chapter 4). The general algorithm for your program will be similar to the word counting program, but the details will vary. Implement your algorithm in the file spchk.c and use the functions in letters.c to test for the appropriate letters.
(Hint: You might want to write another function similar to delimitp() used in wds.c, but your code will be different from the delimitp() in the text. You can put any additional functions and/or macros you use in your letters.c and letters.h files).
I have provided you with a sample data file in ~ee160/Homework/Hw3/moolelo, but you should test your program first with your own data. The makefile in that directory can also be used to compile this program with the command:
make spchk
Your executable will be called spchk.
WDS.C
/* Program File: wds.c Other Source Files: chrutil.c Header Files: tfdef.h, chrutil.h This program reads standard input characters and prints each word on a separate line. It also counts the number of lines, words, and characters. All characters are counted including the newline and other control characters, if any. */ #include
int is_vowel(char c){ return c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U' || c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u'; }
int is_h_consonant(char c){ return c == 'H' || c == 'h' || c == 'K' || c == 'k' || c == 'L' || c == 'l' || c == 'M' || c == 'm' || c == 'N' || c == 'n' || c == 'P' || c == 'p' || c == 'W' || c == 'w'; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
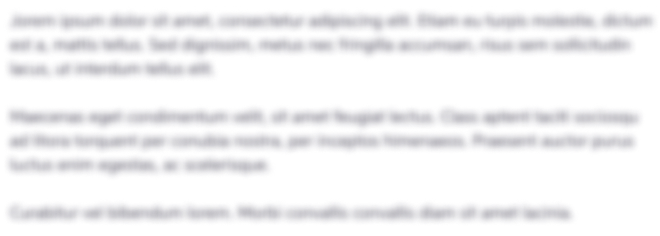
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started