Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Stack ADT The C++ Standard Template Library comes with a stack ADT. The member functions of the stack ADT are as follows: stack s -
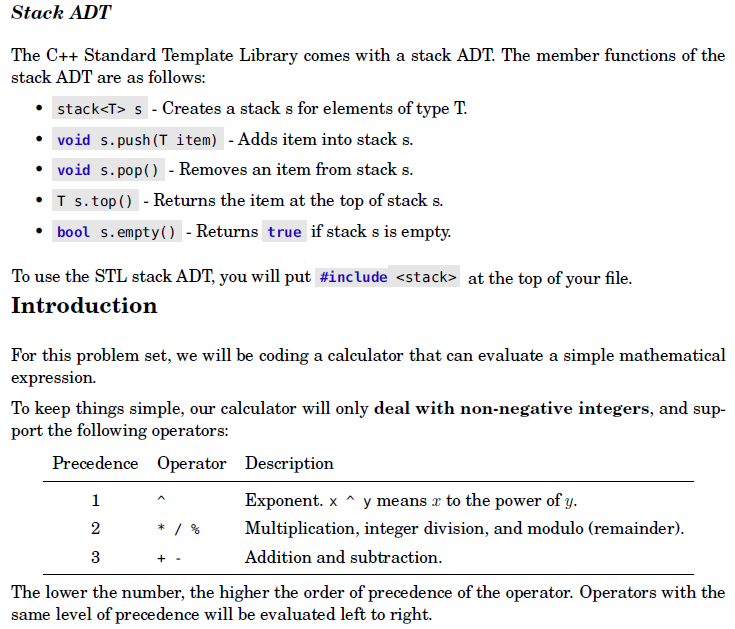
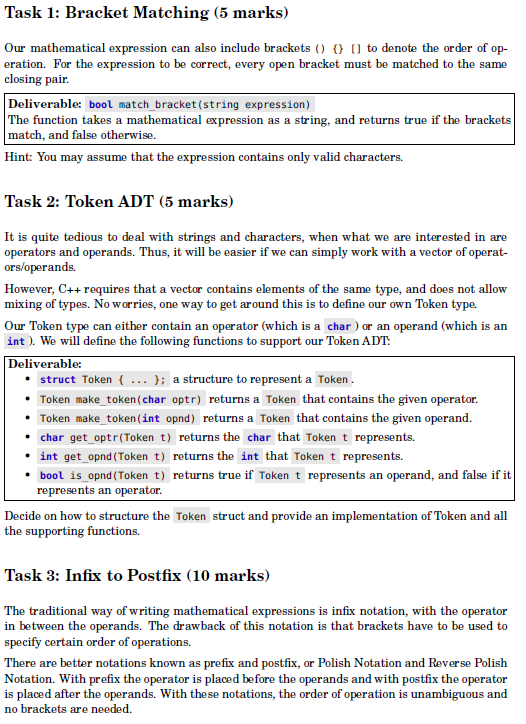
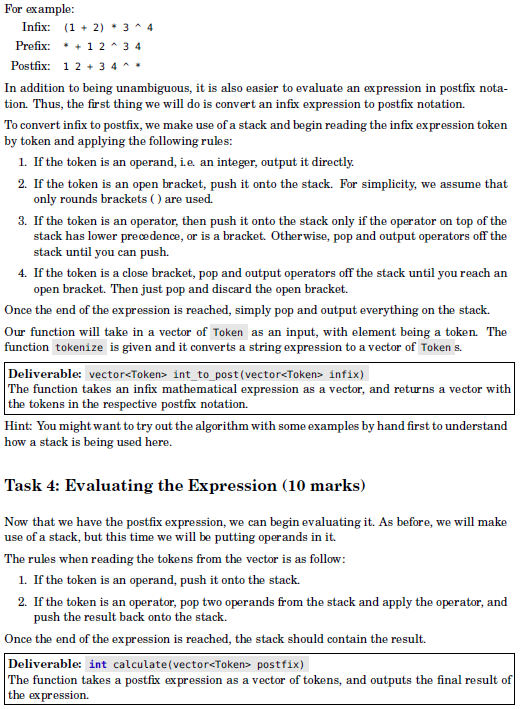
Step by Step Solution
There are 3 Steps involved in it
Step: 1
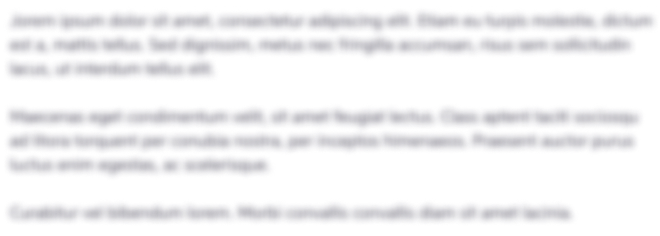
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started