Question
Stack calculator I want make a Stack calculator. My code is #ifndef STACK_H #define STACK_H #include #include #include template class Stack { public: // Default
Stack calculator
I want make a Stack calculator.
My code is
#ifndef STACK_H
#define STACK_H
#include
#include
#include
template
class Stack
{
public:
// Default constructor
Stack(){
capacity = 10;
top = -1;
array = new type[capacity];
};
// Destructor
~Stack();
// Return top element of stack
type& Top();
void Push(const type& item);
void Pop();
bool IsEmpty() const;
void Print() {
for(int i=0; i<=top; i++) std::cout << array[i] << std::endl;
};
type getpop();
int Size() { return top+1; }
private:
// Data
type *array;
int capacity;
int top;
};
#ifndef STACK_TXX
#define STACK_TXX
#include "stack.txx"
#endif
#endif
stack.txx
template
Stack
{
delete [] array;
}
template
type& Stack
{
if(top < 0) assert(false);
return array[top];
}
template
void Stack
{
if(top == capacity-1) // double capacity
{
type* temp = new type[capacity*2];
for(int i=0; i { temp[i] = array[i]; } type* ret = array; array = temp; delete [] ret; capacity *= 2; } array[ ++top ] = item; } template void Stack { if(top >= 0) top--; } template bool Stack { if(top < 0) return true; else return false; } My main calculator.h #ifndef CALCULATOR_H #define CALCULATOR_H #include "stack.h" #include "string" #include #include template type Stack { if(!IsEmpty()) return array[top--]; } bool isoperator(char ch) { if(ch == '+' || ch == '-' || ch == '*' || ch == '/') return true; else return false; } bool number(char n) { if(n >= '0' && n <= '9') return true; else return false; } int order(char a) { switch(a) { case '+': case '-': return 1; case '*': case '/': return 2; default: return 0; } } void Postfix(char* st, char* oper) { char* result; Stack char a; int i =0, j=0, top=0; for(; st[i]!='\0'; i++) { a=st[i]; if(a=='(') { stacks.Push(a); i++; } else if(a==')') { while(!stacks.IsEmpty()&&stacks.Top()!='(') { oper[j++] = stacks.getpop(); } stacks.Pop(); i++; } else if(isoperator(a)) { while(!stacks.IsEmpty()&&order(stacks.Top())>=order(a)) { oper[j++] = stacks.getpop(); } stacks.Push(a); } else { oper[j++]=a; } while(top > 0) { oper[j++] = stacks.getpop(); top--; } int k =0; while(!stacks.IsEmpty()) { st[k]=stacks.Top(); stacks.Pop(); } } while(!stacks.IsEmpty()) { result += stacks.getpop(); } } float calculator(char a, double b, double c) { switch(a) { case '+': return b + c; case '-': return b - c; case '*': return b * c; case '/': return b / c; } } double Eval(char* in) { char* oper; Postfix(in, oper); char temp[2]; int p=0, q=0; for(; oper[p]!= '\0'; p++) { switch(oper[p]) { case '+': case '-': case '*': case '/': in[q-1]=calculator(oper[p],in[q-1],in[q]); q--; break; default: temp[0] = temp[p]; in[++q] = atof(temp); } } return in[q]; } #endif I want to make right code for calculator.h But, it outs segementation fault. Change my code please. And stack.h and stack.txx can't change
Step by Step Solution
There are 3 Steps involved in it
Step: 1
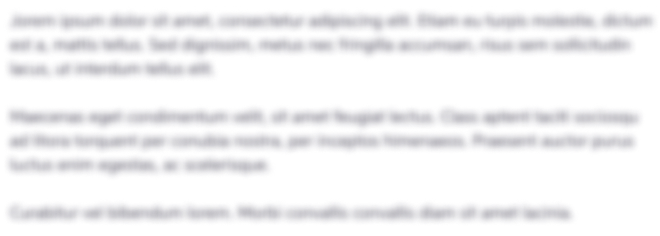
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started