Question
stage 3 The application should create a NoItemsEnteredException. The application should create a static method called itemsEntered which returns the total number of items purchased.
stage 3
The application should create a NoItemsEnteredException. The application should create a static method called itemsEntered which returns the total number of items purchased. If there are no items, the method should throw the NoItemsEnteredException.
heres sample output
Peanut Butter Fudge $ 8.98 [Tax: $0.63] 2.25 lbs @ $3.99 Candy Cane $ 0.75 [Tax: $0.05] 0.50 lbs @ $1.50 Oatmeal Raisin Cookies $ 1.33 [Tax: $0.09] 4 cookies @ $3.99 per Dozen Chocolate Chip Cookies $ 4.25 [Tax: $0.30] 12 cookies @ $4.25 per Dozen Vanilla Ice Cream $ 2.75 2 scoop(s) @ $1.05/scoop + $0.65 Cherry Garcia Ice Cream $ 5.29 3 scoop(s) @ $1.33/scoop + $1.30 CANDY [ 2] Cost: $ 9.73 Tax: $0.68 COOKIES [ 2] Cost: $ 5.58 Tax: $0.39 ICE CREAM [ 2] Cost: $ 8.04 ---- -------- ----- Subtotals [ 6] $23.35 $1.07 ======== Total $24.42
here is my code
package desertshop;
import java.util.Scanner;
import java.text.NumberFormat;
enum DesertType {
CANDY , COOKIE ,ICECREAM ;
}
/**
*
* @author wesley v
*/
public class DesertShop {
private Candy candies[] = new Candy[5];
private Cookie cookies[] = new Cookie[5];
private IceCream IceCreams[] = new IceCream[5];
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
/* Candy item1 = new Candy("Penut Butter Fudge", 2.25, 3.99);
Cookie item2 = new Cookie ("Oatmeal Raisin Cookies ", 4, 3.99);
IceCream item3 = new IceCream ( "Vanilla Ice Cream", 2, 1.05, 0.45);
System.out.println( item1 );
System.out.println( item2 );
System.out.println( item3 );*/
DesertType Choice ;
String name;
int number;
double price , toppingPrice = 0 , totalCandy= 0 , totalTaxCandy= 0 , totalIceCream = 0, totalTaxIceCream = 0,
totalCookie = 0 , totalTaxCookie= 0 , numPounds;
int candiesCount = 0 , cookiesCount = 0, iceCreamCount = 0 ;
String choiceStr;
DesertShop desertshop = new DesertShop();
do {
System.out.println(" Enter Desert Type: 1.Candy 2.Cookie 3.Ice Cream 4 Type Enter To Get Reciept ");
Scanner sc = new Scanner(System.in);
choiceStr = sc.nextLine();
if (choiceStr.isEmpty()){
break;
}
Choice = DesertType.valueOf(choiceStr);
switch (Choice){
case CANDY:
System.out.println("Enter Name of The Candy");
name = sc.nextLine();
if ( sc.hasNextLine())
{
sc.nextLine();
}
System.out.println("Enter Number Of Pounds");
numPounds = sc.nextDouble();
System.out.println("Enter price per per pound");
price = sc.nextDouble();
desertshop.candies[candiesCount++] = new Candy(name, numPounds, price);
break;
case COOKIE:
System.out.println("Enter Name of The Cookie");
name = sc.nextLine();
if ( sc.hasNextLine())
{
sc.nextLine();
}
System.out.println("Enter Number Of Cookies");
number = sc.nextInt();
System.out.println("Enter price per Dozen");
price = sc.nextDouble();
desertshop.cookies[cookiesCount++] = new Cookie(name, number, price);
break;
case ICECREAM:
System.out.println("Enter Name of The Ice Cream");
name = sc.nextLine();
if ( sc.hasNextLine())
{
sc.nextLine();
}
System.out.println("Enter Number Of Scoops");
number = sc.nextInt();
System.out.println("Enter price per Scoop");
price = sc.nextDouble();
System.out.println("Enter price per Topping");
toppingPrice = sc.nextDouble();
desertshop.IceCreams[iceCreamCount++] = new IceCream(name, number , price , toppingPrice);
break;
}
} while(true);
for(int i=0;i< cookiesCount;i++){
totalCookie+=(desertshop.cookies[i].getCost());
totalTaxCookie+=desertshop.cookies[i].getTax();
System.out.println(desertshop.cookies[i].toString());
}
for(int i=0;i< candiesCount;i++){
totalCandy+=(desertshop.candies[i].getCost());
totalTaxCandy+=desertshop.candies[i].getTax();
System.out.println(desertshop.candies[i].toString());
}
for(int i=0;i< iceCreamCount;i++){
totalIceCream+=(desertshop.IceCreams[i].getCost());
System.out.println(desertshop.IceCreams[i].toString());
}
NumberFormat formatter = NumberFormat.getCurrencyInstance();
System.out.println("CANDY ["+candiesCount+"] Cost: "+formatter.format(totalCandy) + " Tax: "+ formatter.format(totalTaxCandy));
System.out.println("COOKIES ["+cookiesCount+"] Cost: "+formatter.format(totalCookie) + " Tax: "+ formatter.format(totalTaxCookie));
System.out.println("ICECREAM ["+iceCreamCount+"] Cost: "+formatter.format(totalIceCream));
System.out.println("------------------------------------------------");
System.out.println("SubTotals ["+(candiesCount+iceCreamCount+cookiesCount)+"] "+formatter.format(totalCandy+totalCookie+totalIceCream ) + " "+ formatter.format(totalTaxCandy+ totalTaxCookie));
System.out.println("================================================");
System.out.println("Total "+ formatter.format(totalCandy+totalCookie+totalIceCream+totalTaxCandy+ totalTaxCookie));
}
}
import java.text.NumberFormat;
/**
*
* @author wesley v
*/
class Candy extends DesertItem {
private double weight;
private double priceperPound;
public static final double tax = .07;
public Candy (String name, double unitWeight,double unitPrice){
super(name);
this.weight = unitWeight;
this.priceperPound= unitPrice;
}
@Override
public double getCost(){
return (weight * priceperPound);
}
public double getTax(){
return getCost()* tax;
}
public String toString (){
NumberFormat formatter = NumberFormat.getCurrencyInstance();
double cost = this.getCost();
return (name + "\t\t\t" + formatter.format(cost) + " [Tax:"+formatter.format(cost * tax)+ "]"+" \t" +
this.weight +" lbs @ "+ formatter.format(this.priceperPound));
}
class Cookie extends DesertItem {
private int NumberOfCookies;
private double priceperDozen;
public static final double tax = .07;
public static float cost;
public Cookie (String name, int numberUnits,double unitPrice){
super(name);
this.NumberOfCookies = numberUnits;
this.priceperDozen= unitPrice;
}
@Override
public double getCost(){
return( NumberOfCookies * priceperDozen/12);
}
public double getTax(){
return getCost()* tax;
}
public String toString (){
NumberFormat formatter = NumberFormat.getCurrencyInstance();
double cost = this.getCost();
return (name + "\t\t\t" + formatter.format(cost) + " [Tax:"+formatter.format(cost * tax) +"]" +" \t" +
this.NumberOfCookies +" Cookies @ "+ formatter.format(this.priceperDozen))+" per Dozen";
}
}
abstract class DesertItem {
protected String name;
public DesertItem (){
this.name = "";
}
public DesertItem (String name ){
this.name = name;
}
public final String getName(){
return name;
}
public abstract double getCost();
}
class IceCream extends DesertItem {
private int numberOfSccops;
private double pricePerScoop;
private double toppingPrice;
public IceCream (String name, int numberUnits ,double unitPrice, double toppings){
super(name);
this.numberOfSccops = numberUnits;
this.pricePerScoop = unitPrice;
this.toppingPrice = toppings;
}
@Override
public double getCost(){
return( numberOfSccops * pricePerScoop + toppingPrice);
}
public String toString (){
NumberFormat formatter = NumberFormat.getCurrencyInstance();
return (name +"\t\t\t" + formatter.format(this.getCost())+ " \t" +
this.numberOfSccops+ "scoops@ " + formatter.format(this.pricePerScoop)
+ " + "+ formatter.format(this.toppingPrice) );
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
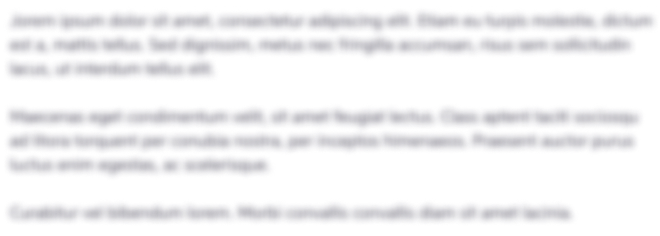
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started