Question
Start by creating a Maven project and importing Mockito. Mockito will be used in roder to test double objects, etc. Your goal for this assignment
Start by creating a Maven project and importing Mockito. Mockito will be used in roder to test double objects, etc.
Your goal for this assignment is to create a method that sysouts "Starting...", waits 1 second, then sysouts "Waited 1 second." then waits 1 second, then sysouts "Waited 2 second.", then waits 1 second, then sysouts "Waited 3 second", then waits 1 second, then sysouts "Waited 4 second.", then waits 1 second, then sysouts "Waited 5 second."
Without TDD, the code is:
System.println("Starting..."); for (int i = 0; i < 5; i++) { Thread.sleep(1000); System.println("Waited " + (i + 1) + " second."); }
You will only need 1 @Test (you're welcome to use more, but that's the bare minimum).
You will need a class called ConsoleThread and test class ConsoleThreadTest.
ConsoleThread will have a single void method: start()
You will need 2 wrappers.
May want a main method somewhere to test the method manually. The main method should just create a new ConsoleThread and call the start() method. Nothing more!
Remember to TDD - one line at a time, forced by the test.
To verify the thread sleep was called 5 times: Mockito.verify(threadWrapper, Mockito.times(5)).sleep(1000);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
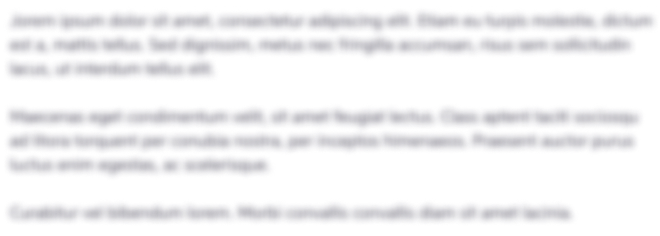
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started