Question
---------START OF linked_list.c----------- #include #include #include linked_list.h // Creates a new List struct and initializes it (the node pointers are NULL and // the count
---------START OF linked_list.c-----------
#include
// Creates a new List struct and initializes it (the node pointers are NULL and // the count = 0) and then returns a pointer to it. List *list_create(void) {
}
// Creates a new ListNode struct and initializes it (the node pointers are NULL // and the value is set to the passed parameter) and then returns a pointer to // it. ListNode *list_create_node(int value) {
}
// Finds a node in the list with the passed value and returns a pointer to the // node. If no matching node is found, returns NULL. ListNode *list_find(List *list, int value) {
}
// Inserts a node in the list after the node containing value. If no node has // the passed value insert at the end of the list. void list_insert_after(List *list, ListNode *node, int value) { }
// Returns the count in the list structure. int list_count(List *list) { return list->count; }
// Returns the first node in the list. ListNode *list_first(List *list) {
}
// Returns the last node in the list. ListNode *list_last(List *list) {
}
// Removes the specified node from the list and frees it. The node's value // is saved and returned. int list_remove_node(List *list, ListNode* node) {
}
// Removes the node with the specified value from the list and return true. If // a node with the value is not found in the list, return false. bool list_remove_value(List* list, int value) {
}
// Inserts a node in the list before the node containing value. If no node has // the passed value insert at the beginning of the list. void list_insert_before(List *list, ListNode *node, int value) { }
// Frees any nodes in the list but leaves the list structure. void list_clear(List *list) {
}
// Frees any nodes in the list and then frees the list structure. List *list_destroy(List *list) {
}
------------START OF linked_list.h------------
#ifndef __libllist_llist_h__
#define __libllist_llist_h__
#include
// ----- List and list node declarations ----------------------------------- //
struct ListNode;
typedef struct ListNode {
struct ListNode *next;
struct ListNode *prev;
int value;
} ListNode;
typedef struct List {
int count;
ListNode *first;
ListNode *last;
} List;
// ----- Function prototypes ----------------------------------------------- //
List *list_create();
ListNode *list_create_node(int);
List *list_destroy(List*);
void list_clear(List*);
int list_count(List*);
ListNode *list_first(List*);
ListNode *list_last(List*);
ListNode *list_find(List*, int);
void list_insert_after(List*, ListNode*, int);
void list_insert_before(List*, ListNode*, int);
int list_remove_node(List*, ListNode*);
bool list_remove_value(List*, int);
#endif
-----------START OF tests.c----------
#include
#include
#include
#include "linked_list.h"
static List *list = NULL;
int test_val1 = 1;
int test_val2 = 2;
int test_val3 = 17;
int value_not_in_list = 161;
ListNode* temp = NULL;
void do_test(bool test, char *success_msg, char *failure_msg) {
if(test) {
printf("%s ", success_msg);
} else {
printf("%s ", failure_msg);
exit(EXIT_FAILURE);
}
}
// The following test functions are all executed in main() in the order they are defined here
void test_create(void) {
list = list_create();
do_test(list != NULL,
"List creation succeeded",
"List creation failed; list_create() returned NULL");
do_test(list->first == NULL,
"list->first set to NULL on creation (as it should be)",
"List creation failed; list->first was not NULL");
do_test(list->last == NULL,
"list->last set to NULL on creation (as it should be)",
"List creation failed; list->last was not NULL");
}
void test_create_node(void) {
temp = list_create_node(test_val1);
do_test(temp != NULL,
"Node creation succeeded",
"Node creation failed; list_create_node() returned NULL");
do_test(temp->value == test_val1,
"Node creation succeeded",
"Node creation failed; node->value not set to passed parameter");
}
void test_insert_after(void) {
list_insert_after(list, list_create_node(test_val1), value_not_in_list);
do_test(list->first->value == test_val1,
"list->first set correctly after first call to list_insert_after()",
"list->first set incorrectly after first call to list_insert_after()");
do_test(list->last->value == test_val1,
"list->last set correctly after first call to list_insert_after()",
"list->last set incorreclty after first call to list_insert_after()");
do_test(list->count == 1,
"list->count set to 1 after first call to list_insert_after()",
"list->count set incorreclty after first call to list_insert_after()");
}
void test_insert_after2(void) {
list_insert_after(list, list_create_node(test_val3), value_not_in_list);
do_test(list->first->value == test_val1,
"list->first set correctly after second call to list_insert_after()",
"list->first set incorrectly after second call to list_insert_after()");
do_test(list->first->next->value == test_val3,
"list->first->next set correctly after second call to list_insert_after()",
"list->first->next set incorrectly after second call to list_insert_after()");
do_test(list->last->value == test_val3,
"list->last->value set correctly after second call to list_insert_after()",
"list->last->value set incorrectly after second call to list_insert_after()");
do_test(list->last->prev->value == test_val1,
"list->last->prev set correctly after second call to list_insert_after()",
"list->last->prev set incorrectly after second call to list_insert_after()");
do_test(list->count == 2,
"list->count set correctly after second call to list_insert_after()",
"list->count set incorrectly after second call to list_insert_after()");
}
void test_insert_after3(void) {
list_insert_after(list, list_create_node(test_val2), test_val1);
do_test(list->first->value == test_val1,
"list->first set correctly after third call to list_insert_after()",
"list->first set incorrectly after third call to list_insert_after()");
do_test(list->first->next->value == test_val2,
"list->first->next set correctly after third call to list_insert_after()",
"list->first->next set incorrectly after third call to list_insert_after()");
do_test(list->first->next->next->value == test_val3,
"list->first->next->next set correctly after third call to list_insert_after()",
"list->first->next->next set incorrectly after third call to list_insert_after()");
do_test(list->first->next->prev->value == test_val1,
"list->first->next->prev set correctly after third call to list_insert_after()",
"list->first->next->prev set incorrectly after third call to list_insert_after()");
do_test(list->last->value == test_val3,
"list->last set correctly after third call to list_insert_after()",
"list->last set incorrectly after third call to list_insert_after()");
do_test(list->last->prev->value == test_val2,
"list->last->prev set correctly after third call to list_insert_after()",
"list->last->prev set incorrectly after third call to list_insert_after()");
do_test(list->count == 3,
"list->count set correctly after third call to list_insert_after()",
"list->count set incorrectly after third call to list_insert_after()");
}
void test_getters(void) {
do_test(list_count(list) == list->count,
"list_count() correctly returns list->count",
"list_count() does not return list->count");
do_test(list_first(list) == list->first,
"list_first() correctly returns list->first",
"list_first() does not return list->first");
do_test(list_last(list) == list->last,
"list_last() correctly returns list->last",
"list_last() does not return list->last");
do_test(list_find(list, value_not_in_list) == NULL,
"list_find() returns NULL when called with a value not in the list",
"list_find() does not return NULL when called with a value not in the list");
do_test(list_find(list, test_val2)->value == test_val2,
"list_find() returns the appropriate node when called with a value present in the list",
"list_find() does not correctly find the requested value's node");
}
void test_remove(void) {
temp = list_find(list, test_val2);
do_test(list_remove_node(list, temp) == test_val2,
"list_remove_node() returns the value of the deleted node",
"list_remove_node() does not return the value of the deleted node");
do_test(list->first->next->value == test_val3,
"list->first->next set correctly after first call to list_remove_node()",
"list->first->next set incorrectly after first call to list_remove_node()");
do_test(list->last->prev->value == test_val1,
"list->last->prev set correctly after first call to list_remove_node()",
"list->last->prev set incorrectly after first call to list_remove_node()");
do_test(list->count == 2,
"list->count set correctly after first call to list_remove_node()",
"list->count set incorrectly after first call to list_remove_node()");
}
void test_remove2(void) {
do_test(list_remove_value(list, test_val3),
"list_remove_value() returns true when deleting a node (2nd call)",
"list_remove_value() does not return true when deleting a node (2nd call)");
do_test(list->first->next == NULL,
"list->first set correctly after second call to list_remove_value()",
"list->first set incorrectly after second call to list_remove_value()");
do_test(list->last->value == test_val1,
"list->last set correctly after second call to list_remove_value()",
"list->last set incorrectly after second call to list_remove_value()");
do_test(list->count == 1,
"list->count set correctly after second call to list_remove_value()",
"list->count set incorrectly after second call to list_remove_value()");
}
void test_remove3(void) {
do_test(list_remove_value(list, test_val1),
"list_remove_value() returns true when deleting a node (3rd call)",
"list_remove_value() does not return true when deleting a node (3rd call)");
do_test(list->first == NULL,
"list->first set correctly after third call to list_remove_value()",
"list->first set incorrectly after third call to list_remove_value()");
do_test(list->last == NULL,
"list->last set correctly after third call to list_remove_value()",
"list->last set incorrectly after third call to list_remove_value()");
do_test(list->count == 0,
"list->count set correctly after third call to list_remove_value()",
"list->count set incorrectly after third call to list_remove_value()");
}
void test_insert_before(void) {
list_insert_before(list, list_create_node(test_val3), value_not_in_list);
do_test(list->first->value == test_val3,
"list->first set correctly after first call to list_insert_before()",
"list->first set incorrectly after first call to list_insert_before()");
do_test(list->last->value == test_val3,
"list->last set correctly after first call to list_insert_before()",
"list->last set incorrectly after first call to list_insert_before()");
do_test(list->count == 1,
"list->count set correctly after first call to list_insert_before()",
"list->count set incorrectly after first call to list_insert_before()");
}
void test_insert_before2(void) {
list_insert_before(list, list_create_node(test_val1), value_not_in_list);
do_test(list->first->value == test_val1,
"list->first set correctly after second call to list_insert_before()",
"list->first set incorrectly after second call to list_insert_before()");
do_test(list->first->next->value == test_val3,
"list->first->next set correctly after second call to list_insert_before()",
"list->first->next set incorrectly after second call to list_insert_before()");
do_test(list->last->value == test_val3,
"list->last set correctly after second call to list_insert_before()",
"list->last set incorrectly after second call to list_insert_before()");
do_test(list->last->prev->value == test_val1,
"list->last->prev set correctly after second call to list_insert_before()",
"list->last->prev set incorrectly after second call to list_insert_before()");
do_test(list->count == 2,
"list->count set correctly after second call to list_insert_before()",
"list->count set incorrectly after second call to list_insert_before()");
}
void test_insert_before3(void) {
list_insert_before(list, list_create_node(test_val2), test_val3);
do_test(list->first->value == test_val1,
"list->first set correctly after third call to list_insert_before()",
"list->first set incorrectly after third call to list_insert_before()");
do_test(list->first->next->value == test_val2,
"list->first->next set correctly after third call to list_insert_before()",
"list->first->next set incorrectly after third call to list_insert_before()");
do_test(list->first->next->next->value == test_val3,
"list->first->next->next set correctly after third call to list_insert_before()",
"list->first->next->next set incorrectly after third call to list_insert_before()");
do_test(list->first->next->prev->value == test_val1,
"list->first->next->prev set correctly after third call to list_insert_before()",
"list->first->next->prev set incorrectly after third call to list_insert_before()");
do_test(list->last->value == test_val3,
"list->last set correctly after third call to list_insert_before()",
"list->last set incorrectly after third call to list_insert_before()");
do_test(list->last->prev->value == test_val2,
"list->last->prev set correctly after third call to list_insert_before()",
"list->last->prev set incorrectly after third call to list_insert_before()");
do_test(list->count == 3,
"list->count set correctly after third call to list_insert_before()",
"list->count set incorrectly after third call to list_insert_before()");
}
void test_list_clear(void) {
list_clear(list);
do_test(list->first == NULL,
"list->first set to null after calling list_clear()",
"list->first not set to null after calling list_clear()");
do_test(list->last == NULL,
"list->last set to null after calling list_clear()",
"list->last not set to null after calling list_clear()");
do_test(list->count == 0,
"list->count set to zero after calling list_clear()",
"list->count not set to zero after calling list_clear()");
}
void test_list_destroy(void) {
// Put things back in the list before destroying it.
list_insert_before(list, list_create_node(test_val3), value_not_in_list);
list_insert_before(list, list_create_node(test_val2), value_not_in_list);
list_insert_before(list, list_create_node(test_val1), value_not_in_list);
list = list_destroy(list);
do_test(list == NULL,
"list_destroy() returned NULL (as it should)",
"list_destroy() did not return NULL (this is a problem)");
}
int main(void)
{
test_create();
test_create_node();
test_insert_after();
test_insert_after2();
test_insert_after3();
test_getters();
test_remove();
test_remove2();
test_remove3();
test_insert_before();
test_insert_before2();
test_insert_before3();
test_list_clear();
test_list_destroy();
printf(" Yay, your implementation passed all the tests! ");
return 0;
}
thanks!!
Overview In this project you will exercise your understanding of structs and pointers to build a useful data structure called a linked list. You will not be writing a program to utilize the linked list library that you write; Instead I have provided you with a test program that will exercise your linked list library to ensure that it is working as it should. These tests will give you immediate feedback implementation is correct and where it is not about your progress showing you where your nked You will be provided with a specification in the form of a .h file which will describe the behaviors your li list must provide Submission Instructions For this project, you should only submit your "linked_list.c" file as all other necessary files are provided for you You do not need to submit a Makefile for this project as the one that was provided to you will be used during grading Technical Description and Instructions What is a Linked List? A linked list is a simple data structure comprised of nodes. Each node holds a piece of data and a connection to the node in front of it and the node behind it. These connections allow the list to be traversed easiljy without requiring the nodes to be stored next to each other in memory. New nodes may be inserted into the list at any point by reassigning the connections of the nodes at the point of insertion. Likewise, nodes may be removed from the list while leaving no 'gaps' by simply ensuring that the nodes next to the removed node are connected to one another. Thus, unlike an arrray, a linked list can expand and contract freely. For this project a linked list will be represented with two structs whose definitions are provided for you inn "linked_list.h". The first, called List, simply tracks the first and last nodes in the list as well as a count of how many nodes the list contains. The second, called ListNode, plays the role of the nodes as discussed above Your Job Your job for this project is to implement the functions whose signatures are declared in the 'linked_list.c" file provided. Once your implementation passes the supplied tests, you're finishedStep by Step Solution
There are 3 Steps involved in it
Step: 1
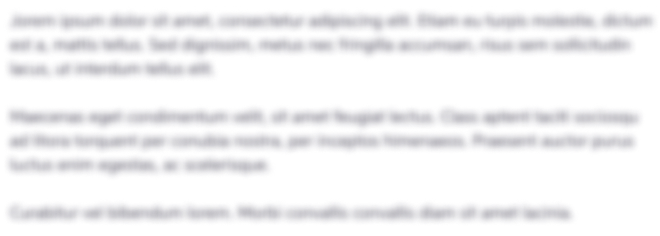
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started