Question
Start with the file AList.java and add the following methods: Add the method 1.public int getLastIndex(T item) which returns the index of the last entry
Start with the file AList.java and add the following methods:
Add the method
1.public int getLastIndex(T item)
which returns the index of the last entry which is equal to item. If item is not found, it returns -1. (15 points)
Add the method
2.public int removeEvery(T item) that removes all occurrences of item and returns the number of removed items. (15 points)
Add the method
3.public boolean equals(Object other)
that returns true when the contents of 2 AList objects are the same. Note that 2 AList objects are the same if they have the same number of items and each item in one object is equal to the item in its corresponding location in the other object (15 points)
AList.Java
import java.util.Arrays; /** A class that implements the ADT list by using an array. The list is never full. @author Frank M. Carrano */ public class AList
if ((givenPosition >= 1) && (givenPosition <= numberOfEntries)) { assert !isEmpty(); result = list[givenPosition - 1]; // get entry to be removed // move subsequent entries towards entry to be removed, // unless it is last in list if (givenPosition < numberOfEntries) removeGap(givenPosition); numberOfEntries--; } // end if return result; // return reference to removed entry, or // null if either list is empty or givenPosition // is invalid } // end remove
public void clear() { numberOfEntries = 0; } // end clear
public boolean replace(int givenPosition, T newEntry) { boolean isSuccessful = true; if ((givenPosition >= 1) && (givenPosition <= numberOfEntries)) { // test catches empty list assert !isEmpty(); list[givenPosition - 1] = newEntry; } else isSuccessful = false; return isSuccessful; } // end replace public T getEntry(int givenPosition) { T result = null; // result to return if ((givenPosition >= 1) && (givenPosition <= numberOfEntries)) { assert !isEmpty(); result = list[givenPosition - 1]; } // end if return result; } // end getEntry public boolean contains(T anEntry) { boolean found = false; for (int index = 0; !found && (index < numberOfEntries); index++) { if (anEntry.equals(list[index])) found = true; } // end for return found; } // end contains public int getLength() { return numberOfEntries; } // end getLength public boolean isEmpty() { return numberOfEntries == 0; // or getLength() == 0 } // end isEmpty public T[] toArray() { // the cast is safe because the new array contains null entries @SuppressWarnings("unchecked") T[] result = (T[])new Object[numberOfEntries]; for (int index = 0; index < numberOfEntries; index++) { result[index] = list[index]; } // end for return result; } // end toArray // Doubles the size of the array list if it is full. private void ensureCapacity() { if (numberOfEntries == list.length) list = Arrays.copyOf(list, 2 * list.length); } // end ensureCapacity private void makeRoom(int newPosition) { assert (newPosition >= 1) && (newPosition <= numberOfEntries + 1); int newIndex = newPosition - 1; int lastIndex = numberOfEntries - 1; // move each entry to next higher index, starting at end of // list and continuing until the entry at newIndex is moved for (int index = lastIndex; index >= newIndex; index--) list[index + 1] = list[index]; } // end makeRoom private void removeGap(int givenPosition) { assert (givenPosition >= 1) && (givenPosition < numberOfEntries); int removedIndex = givenPosition - 1; int lastIndex = numberOfEntries - 1; for (int index = removedIndex; index < lastIndex; index++) list[index] = list[index + 1]; } // end removeGap // Lab 1 public int getIndex(T item) { } public int removeEvery(T item) { } public int getLastIndex(T item) { } public boolean equals(Object other) { } private boolean isTooBig() { } private void reduce() { } } // end AList
ListInterface
/** An interface for the ADT list. Entries in the list have positions that begin with 1.
@author Frank M. Carrano @version 3.0 */ public interface ListInterface
ListClient
public class ListClient { public static void main(String[] args) { testList(); } // end main
public static void testList() { ListInterface
runnerList.add("16"); // winner runnerList.add(" 4"); // second place runnerList.add("33"); // third place runnerList.add("27"); // fourth place displayList(runnerList); } // end testList public static void displayList(ListInterface
Step by Step Solution
There are 3 Steps involved in it
Step: 1
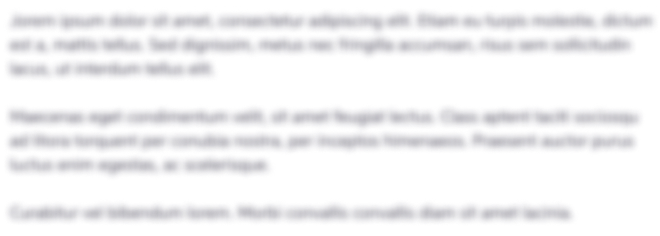
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started