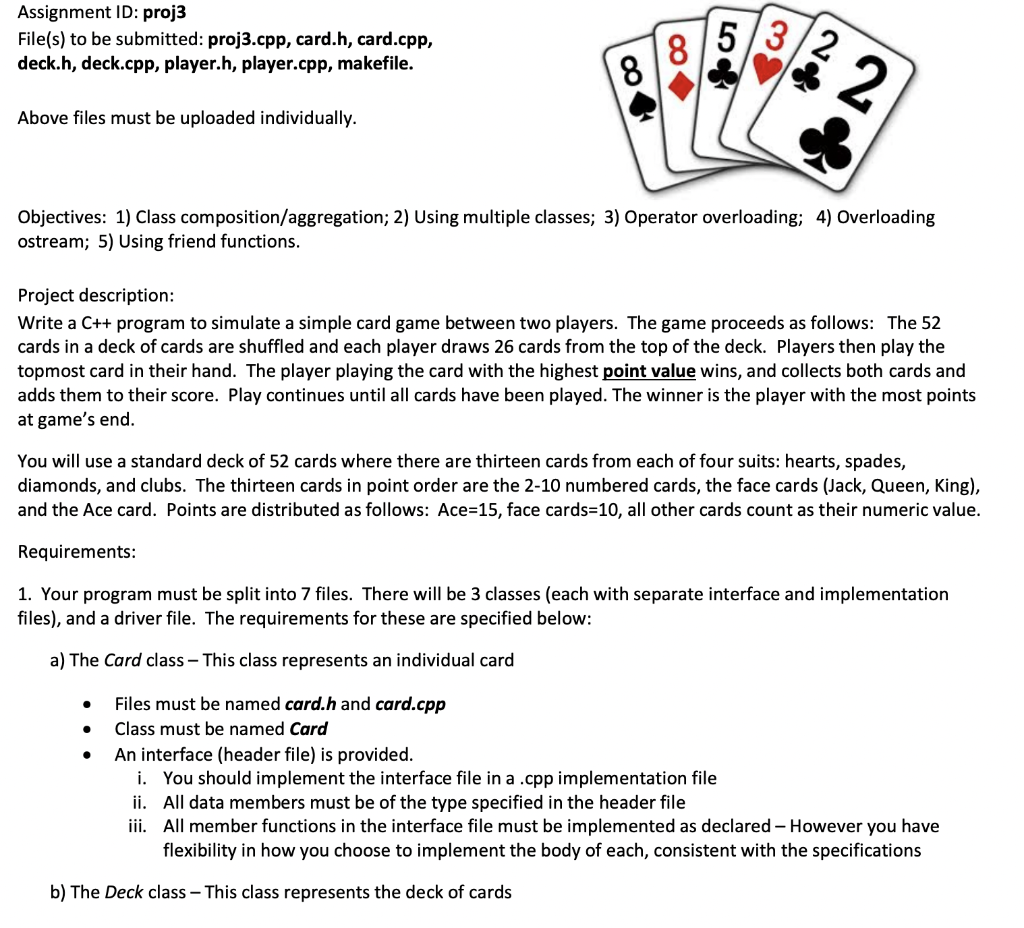
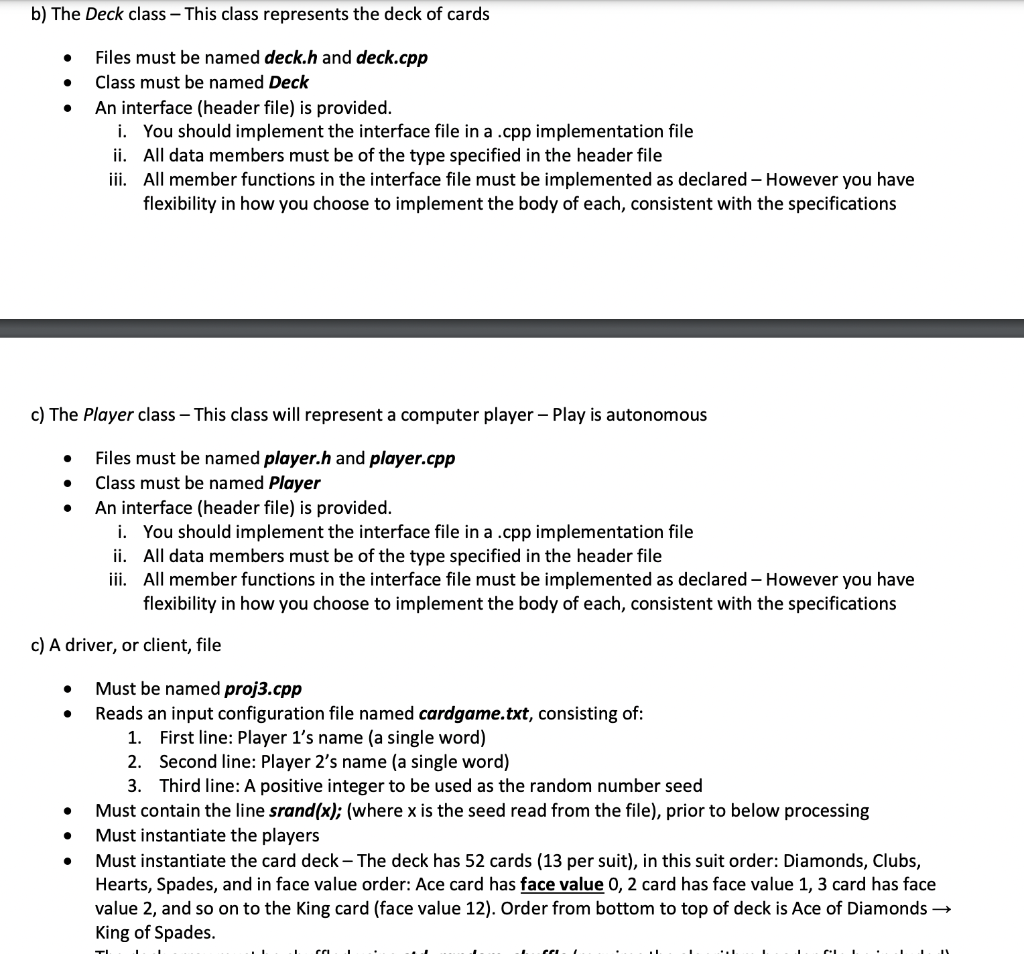
Starter files:
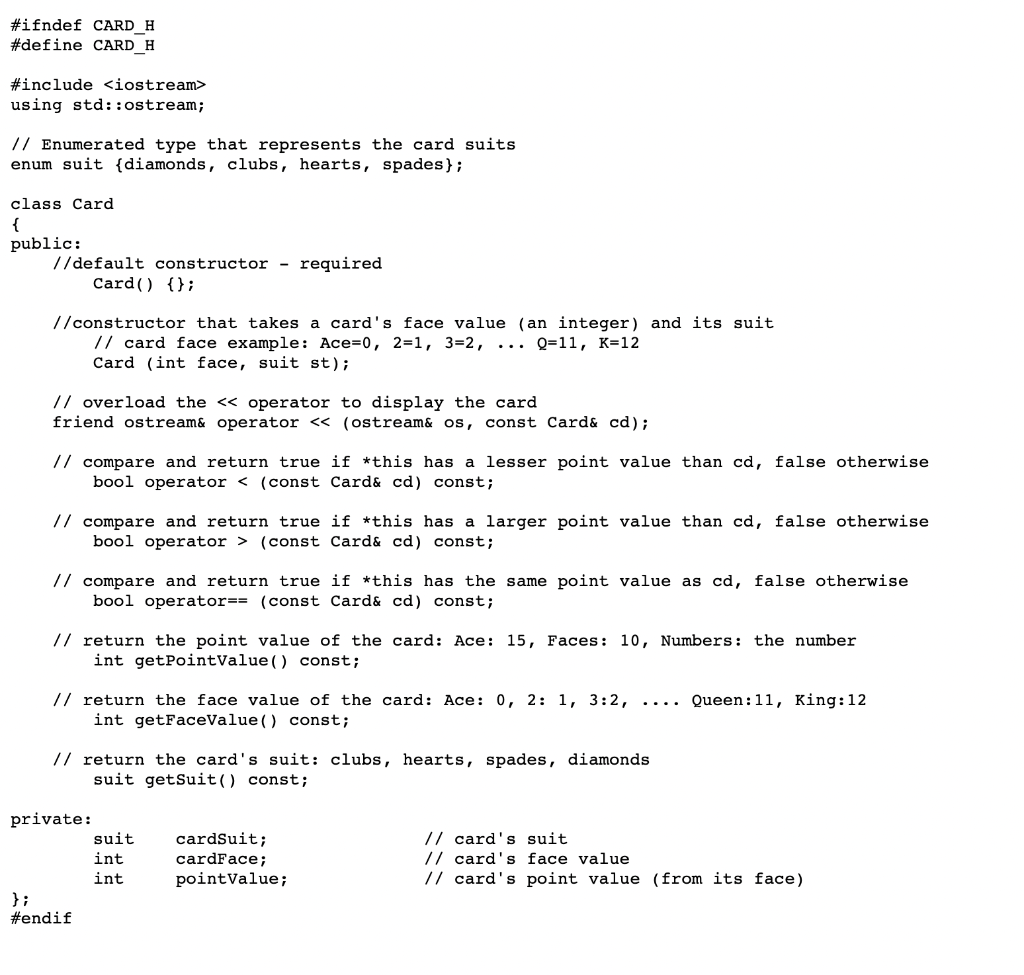
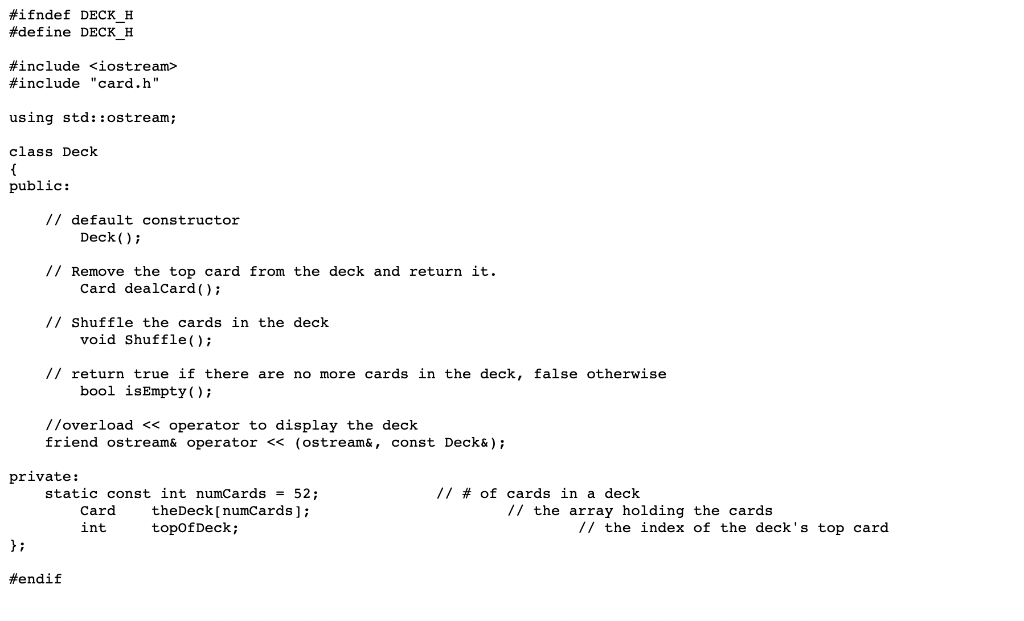
* Proj3.cpp, card.cpp, deck.cpp, player.cpp, and makefile needed*
Assignment ID: proj3 File(s) to be submitted: proj3.cpp, card.h, card.cpp, deck.h, deck.cpp, player.h, player.cpp, makefile. 8 5/3 8 Above files must be uploaded individually. Objectives: 1) Class composition/aggregation; 2) Using multiple classes; 3) Operator overloading; 4) Overloading ostream; 5) Using friend functions. Project description: Write a C++ program to simulate a simple card game between two players. The game proceeds as follows: The 52 cards in a deck of cards are shuffled and each player draws 26 cards from the top of the deck. Players then play the topmost card in their hand. The player playing the card with the highest point value wins, and collects both cards and adds them to their score. Play continues until all cards have been played. The winner is the player with the most points at game's end. You will use a standard deck of 52 cards where there are thirteen cards from each of four suits: hearts, spades, diamonds, and clubs. The thirteen cards in point order are the 2-10 numbered cards, the face cards (Jack, Queen, King), and the Ace card. Points are distributed as follows: Ace=15, face cards=10, all other cards count as their numeric value. Requirements: 1. Your program must be split into 7 files. There will be 3 classes (each with separate interface and implementation files), and a driver file. The requirements for these are specified below: a) The Card class - This class represents an individual card . Files must be named card.h and card.cpp Class must be named Card An interface (header file) is provided. i. You should implement the interface file in a .cpp implementation file ii. All data members must be of the type specified in the header file iii. All member functions in the interface file must be implemented as declared - However you have flexibility in how you choose to implement the body of each, consistent with the specifications b) The Deck class - This class represents the deck of cards b) The Deck class - This class represents the deck of cards . . Files must be named deck.h and deck.cpp Class must be named Deck An interface (header file) is provided. i. You should implement the interface file in a .cpp implementation file ii. All data members must be of the type specified in the header file iii. All member functions in the interface file must be implemented as declared - However you have flexibility in how you choose to implement the body of each, consistent with the specifications c) The Player class - This class will represent a computer player - Play is autonomous . Files must be named player.h and player.cpp Class must be named Player An interface (header file) is provided. i. You should implement the interface file in a .cpp implementation file ii. All data members must be of the type specified in the header file iii. All member functions in the interface file must be implemented as declared - However you have flexibility in how you choose to implement the body of each, consistent with the specifications c) A driver, or client, file . . Must be named proj3.cpp Reads an input configuration file named cardgame.txt, consisting of: 1. First line: Player 1's name (a single word) 2. Second line: Player 2's name (a single word) 3. Third line: A positive integer to be used as the random number seed Must contain the line srand(x); (where x is the seed read from the file), prior to below processing Must instantiate the players Must instantiate the card deck The deck has 52 cards (13 per suit), in this suit order: Diamonds, Clubs, Hearts, Spades, and in face value order: Ace card has face value 0, 2 card has face value 1, 3 card has face value 2, and so on to the King card (face value 12). Order from bottom to top of deck is Ace of Diamonds King of Spades. . . . King of Spades. The deck array must be shuffled using std::random_shuffle (requires the algorithm header file be included) - Use std::begin and std::end on the deck array to pass as iterators to this function. Do not use std::shuffle. Each player alternates taking a card from the top of the deck until all cards are dealt. The game begins now: Game play proceeds as follows: 1. Each player plays the top card in their hand 2. The player playing the card with the highest point value wins and collects both cards, adding their point values to their score. If both cards played have the same point value, the hand is a draw (tie) and no points are accumulated by either player. 3. Play continues until all card have been played. Output for this program must clearly and neatly show that the program works and that it works correctly. Your output must match the one provided. The suits, Clubs, Hearts, Diamonds, and Spades are shown as (C, H, D, and S) respectively, immediately following the card face. Point values follow in brackets. Your code must: 1. Display the entire deck of cards after it is instantiated (before shuffling it) - Top of deck displayed first. 2. Display the shuffled deck (prior to players picking their first 3 cards) - Top of deck displayed first. 3. Players alternate drawing the card at the top of the deck - player 1 then player 2 - to fill their hand 4. For each turn of play a single line is output (see sample output) showing the following: Turn #, and for each player (player 1 first) the player's name, card played, and post-play score (in parentheses) The winner of the turn is identified by an asterisk next to the player name (see sample output) Turn output lines should be separated by a blank line (see sample output) 5. At the end of the game, output one of the following: The word Winner" followed by the name of the player with the highest score, and their score The word "Tie", followed by the tie score A sample output file of a complete game is provided. Your output must match this format exactly, as far as spacing and content. You are not required to create this output file. It is an example for you to follow. . . . - Test your program - Use different seed values for random initialization. #ifndef CARD H #define CARD_H #include
using std::ostream; // Enumerated type that represents the card suits enum suit {diamonds, clubs, hearts, spades}; class Card { public: //default constructor - required Card() {}; //constructor that takes a card's face value (an integer) and its suit // card face example: Ace=0, 2=1, 3=2, ... Q=11, K=12 Card (int face, suit st); 1/ overload the operator to display the card friend ostream& operator (const Card& cd) const; Il compare and return true if *this has the same point value as cd, false otherwise bool operator== (const Card& cd) const; // return the point value of the card: Ace: 15, Faces: 10, Numbers: the number int getPointValue() const; Queen:11, King:12 // return the face value of the card: Ace: 0, 2:1, 3:2, int getFaceValue() const; // return the card's suit: clubs, hearts, spades, diamonds suit getSuit() const; private: suit int int cardSuit; cardFace; pointValue; // card's suit // card's face value // card's point value (from its face) #endif #ifndef DECK H #define DECK_H #include #include "card.h" using std::ostream; class Deck { public: // default constructor Deck(); // Remove the top card from the deck and return it. Card dealCard(); // Shuffle the cards in the deck void Shuffle(); // return true if there are no more cards in the deck, false otherwise bool isEmpty(); 1/overload #include #include "deck.h" #include "card.h" using std::ostream; using std::string; class Player { public: static const int Max_Cards = 26; // # of cards a player can have in a hand 1/ constructor - player's name defaults to "unknown" if not supplied Player (string pname="unknown"); // draw top card from the deck to fill the hand void drawCard (Deck& dk); // Simulates player playing a card - returns the top card in player's hand Card playCard(); // add point value of th card to the player's score void addScore (Card acard); // return the score the player has earned so far int getScore() const; // return the name of the player string getName() const; // return true if the player's hand is out of cards bool emptyHand() const; // overload the operator to display cards in player's hand friend std::ostream& operator using std::ostream; // Enumerated type that represents the card suits enum suit {diamonds, clubs, hearts, spades}; class Card { public: //default constructor - required Card() {}; //constructor that takes a card's face value (an integer) and its suit // card face example: Ace=0, 2=1, 3=2, ... Q=11, K=12 Card (int face, suit st); 1/ overload the operator to display the card friend ostream& operator (const Card& cd) const; Il compare and return true if *this has the same point value as cd, false otherwise bool operator== (const Card& cd) const; // return the point value of the card: Ace: 15, Faces: 10, Numbers: the number int getPointValue() const; Queen:11, King:12 // return the face value of the card: Ace: 0, 2:1, 3:2, int getFaceValue() const; // return the card's suit: clubs, hearts, spades, diamonds suit getSuit() const; private: suit int int cardSuit; cardFace; pointValue; // card's suit // card's face value // card's point value (from its face) #endif #ifndef DECK H #define DECK_H #include #include "card.h" using std::ostream; class Deck { public: // default constructor Deck(); // Remove the top card from the deck and return it. Card dealCard(); // Shuffle the cards in the deck void Shuffle(); // return true if there are no more cards in the deck, false otherwise bool isEmpty(); 1/overload #include #include "deck.h" #include "card.h" using std::ostream; using std::string; class Player { public: static const int Max_Cards = 26; // # of cards a player can have in a hand 1/ constructor - player's name defaults to "unknown" if not supplied Player (string pname="unknown"); // draw top card from the deck to fill the hand void drawCard (Deck& dk); // Simulates player playing a card - returns the top card in player's hand Card playCard(); // add point value of th card to the player's score void addScore (Card acard); // return the score the player has earned so far int getScore() const; // return the name of the player string getName() const; // return true if the player's hand is out of cards bool emptyHand() const; // overload the operator to display cards in player's hand friend std::ostream& operator