Question
Starting Point Our Old MacDonald farm will have chicks, cows, dogs (strays that run on the farm), pigs, and farm dogs. We will create a
Starting Point Our Old MacDonald farm will have chicks, cows, dogs (strays that run on the farm), pigs, and farm dogs. We will create a verse for each of the animals. There is a Zip file in Blackboard which accompanies this assignment. Download and save the Zip file on your computer. Start by looking at Animal.java. This is an interface. It contains 2 methods that you will need for each animal: getAnimalSound() so that we know what sound the animal makes, and getAnimalType() so that we know the type of animal we have. Next, you will build a class structure of farm animals. Each farm animal class will implement Animal. Take a look at Chick.java as an example. Notice the class header that has the phrase implements Animal. That means this class must contain a definition for the two methods in the Animal interface. Chick has 2 instance variables: (1) type and (2) sound. You can see how these are given values in the constructor. You can also see how Animal is implemented in Chick by defining the two methods: (1) getAnimalSound() and (2) getAnimalType(). Most of the other animal classes you create will mimic Chick. Look at MacDonaldFarm.java. This is a class which establishes an array of the farm animals. Its toString() method builds the text for the song. Look at Song.java. This is a driver (application) which displays the song by instantiating the MacDonaldFarm class. Test this by: Compiling Animal, Chick, MacDonaldFarm, and Song Executing Song
Your Homework Create the other farm animal classes (remember they implement Animal): Create a Cow class (cows moo) Create a Dog class (dogs woof) Create a Pig class (pigs oink) Update the MacDonaldFarm class so that: The array can hold 4 animals Instantiate one of each of the animals (the Chick is already done) Does the toString() method need to be updated? Why or why not? Test your new classes and debug until the song is displayed correctly Important You need to understand what has happend: The array is an array of Animal objects. Yet we instantiated and placed Chick, Cow, Dog, and Pig objects in the array. When the toString() method uses getAnimalSound() and getAnimalType(), Java has to figure which method to use (the one from the Chick class, the one from the Cow class, etc.). Java does this dynamically using a feature called polymorphism when the program executes (not when the program is compiled into bytecode). This is also known as late binding because the decision is not known or made until run-time. Now we will add some inheritance Create a new class called farmDog (they woof like any other dog). This new class extends Dog (Dog is the parent) and also implements Animal. Order is important always extend a class then implement an interface on the class header. Add an instance variable to the class called name. (Because we always name our farm dogs). The constructor has one parameter (the name of the dog). Remember how inheritance works! The constructor should call the parent constructor (using super()). This will establish the type and sound the farmDog has. The constructor should update the name of the dog (the instance variable in the farmDog class) using the constructors parameter. The constructor should update type for a farmDog so that the type is the dogs name + the type of animal. For example, a farm dog name Phoebe would now have a type of Phoebe dog. Remember type is defined in the parent (Dog) but accessible to farmDog. Create an accessor for name. Update the MacDonaldFarm class so that: The array can now hold 5 animals Instantiate a farmDog object and add to the array. Test and debug all of your code again.
Animal.java
//-----------------------------------------
// Animal interface for Old MacDonald
//-----------------------------------------
public interface Animal {
public String getAnimalSound();
public String getAnimalType();
}
Chick.java
//--------------------------------------
// Chick.java
//
// Implements Animal interface
//--------------------------------------
public class Chick implements Animal {
//--------------------------------------
// Instance data
//--------------------------------------
private String type;
private String sound;
//--------------------------------------
// Constructor sets type and sound
// for a chick.
//--------------------------------------
public Chick () {
type = "chick";
sound = "cheep";
}
//--------------------------------------
// Accessors for Chick
// These two are required because this
// class implements the Animal interface
//--------------------------------------
public String getAnimalSound() {
return sound;
}
public String getAnimalType() {
return type;
}
}
MacDonaldFarm.java
//--------------------------------------
// MacDonaldFarm
//
// Class of all animals on Old
// MacDonald's farm
//--------------------------------------
public class MacDonaldFarm
{
private Animal[] hisFarm;
//-----------------------------------------------------------------
// Constructor: Sets up the list of staff members.
//-----------------------------------------------------------------
public MacDonaldFarm () {
hisFarm = new Animal[1];
hisFarm[0] = new Chick();
}
public String toString() {
Animal currentAnimal;
String song = "";
String sound = "";
song = "Old MacDonald had a farm E I E I E ";
for (int i = 0; i < hisFarm.length; i++)
{ currentAnimal = hisFarm[i];
sound = currentAnimal.getAnimalSound();
song += "And on the farm he has a " + currentAnimal.getAnimalType() + " E I E I O! With a ";
song += sound + "-" + sound + " here and a " + sound + "-" + sound + " there Here a " + sound;
song += " -- there a " + sound + " Everywhere a " + sound + "-" + sound + " Old MacDonald had a farm E I E I O ";
}
return song;
}
}
Song.java
//******************************************************************** // Song.java
//********************************************************************
public class Song { //----------------------------------------------------------------- // Creates a staff of employees for a firm and pays them. //----------------------------------------------------------------- public static void main (String[] args) { MacDonaldFarm theSong = new MacDonaldFarm();
System.out.println (theSong); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
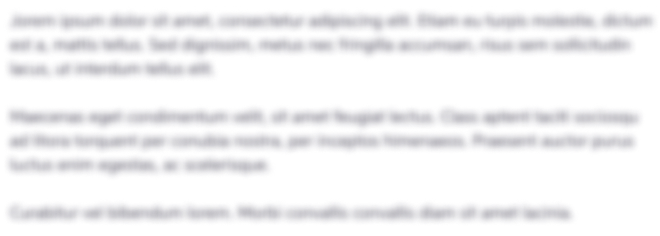
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started