Question
Step One Create a class called Card that represents a playing card. It should have two integer data members: suit. This represents one of 4
Step One
Create a class called Card that represents a playing card. It should have two integer data members:
- suit. This represents one of 4 suits so it will always have a value of 1, 2, 3, or 4. 1 represents hearts, 2 represents diamonds, 3 represents clubs, and 4 represents spades.
- face. This represents one of 13 faces, and they should be numbered from .14, with 11 representing the Jack, 12 representing the Queen, 13 representing the King and 14 representing the Ace, which is rank order of playing cards. So face will always have a value between 2..14 inclusive
Your Card class needs a default constructor, and a constructor that takes parameters for the two data members. It also should have a toString method that creates a string representation of a Card (e.g. King of Clubs or Three of Spades, etc.).
Step Two
Modify Card so that it implements the Comparable interface. For this exercise, we will compare cards by face only and ignore suit. For example, a Card with a face value of 14 is higher than a Card with a face value of 2.
Step Three
Create a class called Hand that represents a poker hand. It has a single data member that is a 5-element array of Cards. Dont worry about a default constructor, just make a constructor that has 5 parameters, each of type Card. Provider a getter that takes a parameter iand returns Card[i]. Your constructor should use Arrays.sort to sort the hand. It can do this because Card implements the Comparable interface. Having your Hand sorted by face will make the next task much easier.
Step Four
Modify Hand so that it implements the Comparable interface. This means you will have to provide a method compareTo. If c1 is a Card and c2 is a Card, a call to c1.compareTo(c2) will return -1 if c1 has a lower ranking than c2, 0 if they have the same ranking, or 1 if c1 has a higher ranking than c2. This is how poker hands are ranked: https://www.pokerlistings.com/poker-hand-ranking. Note that ties are possible, for example, two royal flushes are a tie and so are two straight flushes, regardless of high card. To simplify things, assume that for any hand except high card, if the two hands have it, it is a tie. For example, if both hands have 3 of a kind, count that as a tie regardless of what the high card is.
main program to test
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
/**
* This program tests a Poker class
* with a simplified ranking system
*
*/
public class PokerTester
{
public static void main(String args[])
{
int suit, face, n;
Random r = new Random();
Hand[] hands = new Hand[10];
Scanner in = new Scanner(System.in);
Card[] deck = new Card[52];
Card c1, c2, c3, c4, c5;
boolean[] dealt = new boolean[52];
n = 0;
for (int i = 1; i <= 4; i++) {
for (int j = 2; j <= 14; j++) {
deck[n++] = new Card(i, j);
}
}
for (int i = 0; i < 52; i++) dealt[i] = false;
// generate 10 random hands, this will use 50 cards
for (int i = 0; i < 10; i++) {
n = r.nextInt(52);
while (dealt[n] == true) n = r.nextInt(52);
c1 = deck[n];
dealt[n] = true;
n = r.nextInt(52);
while (dealt[n] == true) n = r.nextInt(52);
c2 = deck[n];
dealt[n] = true;
n = r.nextInt(52);
while (dealt[n] == true) n = r.nextInt(52);
c3 = deck[n];
dealt[n] = true;
n = r.nextInt(52);
while (dealt[n] == true) n = r.nextInt(52);
c4 = deck[n];
dealt[n] = true;
n = r.nextInt(52);
while (dealt[n] == true) n = r.nextInt(52);
c5 = deck[n];
dealt[n] = true;
hands[i] = new Hand(c1, c2, c3, c4, c5);
}
// sort the hands
Arrays.sort(hands);
// print them out
for (int i = 9; i >= 0; i--) {
for (int j = 0; j < 5; j++) {
System.out.print (hands[i].getCard(j));
System.out.print (" ");
}
System.out.println ("");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
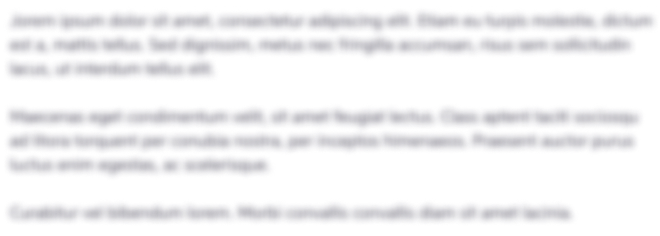
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started