Question
Step One The most general object in this hierarchy is the Shape. Begin by creating a class for a Shape. It will have data members
-
Step One
The most general object in this hierarchy is the Shape. Begin by creating a class for a Shape. It will have data members xand y, which define the upper left-hand corner of the console where the Shape will be drawn. For example is x is 5 and y is 7, the upper left-hand corner of the Shape is 5 spaces from the left margin and 7 spaces from the top margin. Due to the limited size of the standard console window, plus leaving a little room on the bottom, ensure that 0 <= x <= 20, 0 <= y <= 10.If your constructor parameter violates these bounds, set it to 0.
Your Shape class will also need a boolean data member called filled, to indicate whether the Shape should be filled or hollow when drawn.
Provide a default constructor and a constructor that takes parameters for x, y, and filled. Your Shape class will need a draw function member. Since your Shape class has no specific shape, its specific drawing behavior is undefined, and needs to be redefined for any inherited object types so this should be an abstract method. Because Shape has an abstract method, Shape will be an abstract class.
Step Two
Create a Rectangle class that inherits from Shape. In addition to the class members of Shape, a Rectangle needs to know its height (0 < height, y + height <= 40) and width (0 < width, x + width <= 60). Provide a default constructor that sets height and width to 1, and a constructor that takes parameters for x, y, filled, height and width(utilize the Shape constructor). The Rectangle will, of course, need to override the draw method that it inherits from Shape. Draw your rectangle with asterisks. Your rectangle should be drawn with its upper left-hand corner at position x, y in the console. So begin by printing y blank lines. Then each row in your Rectangle should be indented x spaces.
Step Three
Create a VerticalLine class that inherits from Rectangle. A VerticalLine differs from a Rectangle in that its width must be 1 and it is (trivially) filled. So the constructor only needs x, y, and height (0 < height, y + height <= 40). Provide appropriate methods.
.
Step Four
Create a HorizontalLine class that inherits from Rectangle. A HorizontalLine differs from a Rectangle in that its height must be 1 and it is (trivially) filled. So the constructor only needs x, y, andwidth (0 < width, x + width <= 60). Provide appropriate methods.
Step Five
Create a Triangle class that inherits from Shape. See the example output for how to draw a triangle. You will only need to get the width (0 < width, x + width <= 60). Additionally, width must be odd (it will be easier to draw), so enforce this. The constructor will need x, y, filled, and width. Provide appropriate methods.
Step Six
For this assignment, I will provide a main program, but you will need to add exactly 5 lines of code to this main program. See the comments numbered 1 to 5. Do not modify the main program in any other way. Specifically, do not add any additional variables.
Notes
- For this exercise, the purpose of the class methods is to write to the screen, so this is a situation in which System.out.print statements are appropriate to have in your methods.
- Utilize methods of the superclass wherever possible to avoid duplication of code. No subclass should perform any work that the superclass can do.
- You do not need to provide any getters or setters for this homework.
- You do need to provide JavaDoc comments.
- You do not need to create a package for this assignment.
The main program
import java.util.Scanner;
/**
* This is a program that tests our Shape heirarchy of classes
*
*/
public class TestInheritance
{
/**
* This is the main program
*
* @param args the command line arguments
*/
public static void main (String[] args)
{
Shape myShape;
int x=0, y=0, h=1, w=1, f;
boolean fillIt=true;
int choice;
Scanner in = new Scanner(System.in);
do {
System.out.println ("0) quit program");
System.out.println ("1) draw a rectangle");
System.out.println ("2) draw a horizontal line");
System.out.println ("3) draw a vertical line");
System.out.println ("4) draw a triangle");
choice = in.nextInt();
if (choice == 1 || choice == 2 || choice == 3 || choice == 4) {
System.out.println ("Enter x value of upper left corner: ");
x = in.nextInt();
System.out.println ("Enter y value of upper left corner: ");
y = in.nextInt();
if (choice == 1 || choice == 2 || choice == 4) {
System.out.println ("Enter the width you would like: ");
w = in.nextInt();
}
if (choice == 1 || choice == 3) {
System.out.println ("Enter the height you would like: ");
h = in.nextInt();
}
if (choice == 1 || choice == 4) {
System.out.println ("Enter 1 if you want it filled, 0 otherwise: ");
f = in.nextInt();
fillIt = f == 1;
}
if (choice == 1) {
// 1) Write the statement to create a Rectangle
}
else if (choice == 2) {
// 2) Write the statement to create a HorizontalLine
}
else if (choice == 3) {
// 3) Write the statement to create a VerticalLiine
}
else {
// 4) Write the statement to create a Triangle
}
// 5) Write the statement here to draw the shape
}
} while (choice != 0);
}
}
Example output
0) quit program
1) draw a rectangle
2) draw a horizontal line
3) draw a vertical line
4) draw a triangle
1
Enter x value of upper left corner: 5
Enter y value of upper left corner: 3
Enter the width you would like: 15
Enter the height you would like: 3
Enter 1 if you want it filled, 0 otherwise: 1
***************
***************
***************
Step by Step Solution
There are 3 Steps involved in it
Step: 1
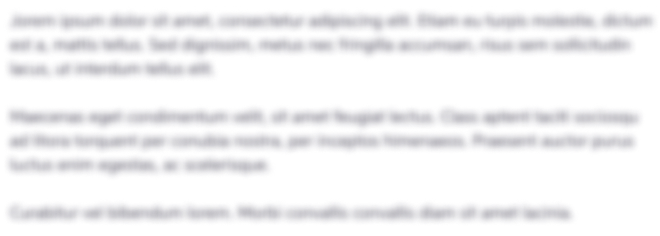
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started