Question
Steps to making your Netbeans IDE (Interactive Development Environment): Download and install Netbeans 8.2 IDE (Java SE version only). If you do not have the
Steps to making your Netbeans IDE (Interactive Development Environment):
Download and install Netbeans 8.2 IDE (Java SE version only). If you do not have the latest Java installation to match, Netbeans will direct you to download the needed version of Java.
If needed, download and install the latest Oracle Official Java Software Development Kit Standard Edition (Java SDK SE), and JUnit. I suggest you do this via the bundle referred to as The Java Development Kit (JDK). Download the architecture specific bundle for your computer. (When in doubt of your computers architecture (on a PC) its probably Windows x86)
Part 1: Create a new Java application Lab2Part1 and then enter the code below in the main routine. Be careful about cutting and pasting. The character representations from MS Word or other editors may not all work the same in NetBeans.
int item1 = 5;
int item2;
double num1 = 3.0;
double num2 = 24;
item2 = 6;
int titem = item2;
System.out.println("Printing values");
System.out.println("int item1 value = "+item1);
System.out.println("int item2 value = "+item2);
System.out.println("double num1 value = "+num1);
System.out.println("double num2 value = "+num2);
System.out.println();
item2 = item2 / item1;
System.out.println( "orig item2/item1 "+titem+"/"+item1+" = item2 = "+item2 );
item2 = 15;
System.out.println("resetting item2 value = "+item2);
System.out.println();
num2 = item2 / num1;
System.out.println( "item2/num1 "+item2+"/"+num1+" = num2 = "+num2 );
item1 = 8;
num2 = item1 * num1 / 2;
System.out.println( "item1* num1/2 "+item1+"*"+num1+"/2 = num2 = "+num2 );
System.out.println();
System.out.println("int item2 value = +item2);
System.out.println("double num1 value = "+num1);
item2 = (item2 1) / 3;
num1 *= item2 + 2.1;
System.out.println(after item2=(item2-1) / 3: item2 value = "+item2);
System.out.println("after num1 *= item2+2.1: num1 value = "+num1);
System.out.println();
System.out.println("num1 < item1 is "+( num1 < item1));
System.out.println( "num2 != item2 is "+(num2 != item2));
System.out.println();
System.out.println("Printing values");
System.out.println("int item1 value = "+item1);
System.out.println("int item2 value = "+item2);
System.out.println("double num1 value = "+num1);
System.out.println("double num2 value = "+num2);
1.a) If you execute this program, what will be printed in the output window? (5 points)
1.b) Rewrite the statement num1 *= item2 + 2.1; to use only assignment and arithmetic operators. (5 pts)
1.c) Give three examples of shortcut arithmetic operators that are NOT used in the program above. Write the symbols for the operators and the names of the operations they are shortcuts for. (5 pts)
Put your answers for 1.a, 1.b, and 1.c in your answers file.
Part 2: Use the code below, including the comment section, in the main method of a new Java application called Lab2Part2a.
/** ** **
Declare the needed input object here using the names shown below in the program.
Also add any other needed lines to make the input object function.
** ** **/
//input here should be "1310 or 1320"
System.out.print("Please enter a 4-digit course number of 1310 or 1320: ");
String courseNum = input.next();
System.out.print("Please enter the current semester (Fall, Spring, or Summer): ");
String sem = input.next();
String dept = "CSE";
char comma = ',';
String sectionNum = "005";
char dash = '-';
int year = 2017;
String sp = " ";
String course; // output should be semester, year, comma, space,
// then dept, course number then section number
course = sem+sp+year+comma+sp+dept+courseNum+dash+sectionNum;
System.out.println("The course is "+course);
2.a) Add the needed code to the program above to make it run. There is one missing line of code in the main method and you need to add it at the location of the first comment. There will also be a line that needs to be added above the class declaration (NetBeans will help you with this). Save the program as Lab2Part2a.java . (5 pts)
2.b) In the answers file, show two of the six possible outputs produced by the program if all instructions in the program are followed. (4 pts)
2.c) What is different about variables dash and comma from the other variables in the program? How are they like Strings and how are they different from Strings? Put your answer in the answers file. (4 pts)
2.d) Rewrite the program so that it accepts the same inputs and produces the same output but variable course is removed from the program. Do not create a new variable to replace course. Save this modified program as Lab2Part2d.java . (7 pts)
Part 3: The code below does not work. Create a new Java program called Lab2Part3.java starting with this code (and answering questions 3.a and 3.b) and then modify the code so that it correctly calculates the area of a right triangle which has a vertex at (4, 5) however :
You may not ADD any lines (if there are needed import statements, they may be added)
You may only modify Lines 1, 2, 3, and 4 as labeled below
you may NOT change the data type of variable base in Line 1
you may NOT change the data type of height in Line 2
you must add the area formula for Line 3
you must modify Line 4 so that is works and calculates the circumference
// code starting here should be put in main
double x1 = 4;
double y1 = 1;
double x2 = 1;
double y2 = 5;
double x3, y3;
int base = (x1 - x2); // Line 1
int height = (y1 - y2); // Line 2
double hypotenuse = Math.sqrt(Math.pow(base,2)+ Math.pow(height,2));
double area;
area = ; // Line 3 - Look up the formula for area and insert it here
System.out.printf("The base is length %d and the height is %3d ", base, height);
System.out.print("The distance between ("+x1+","+y1+") and" );
System.out.printf("(%.0f,%2.0f) is %4.1f ", x2, y2, hypotenuse);
System.out.print("The area of the right triangle is "+area);
circumference = ; // Line 4 - Insert correct calculation here and other as needed
System.out.print("The circumference of the right triangle is "+ circumference);
3.a) List each different type of error, giving the NetBeans error message, that you find after typing this program into Netbeans exactly as given. (Do not copy and paste.) Put these in your answer file. (4 pts)
3.b) Also, in your answers file, for each error, explain exactly (only a few words are needed) why the code, as given above, does not work, i.e. why did each error occur? (4 pts)
3.c) Now correct all the errors and run the program, debugging until it works properly. Only four lines of the program need to be modified to remove the compiling errors including one of the output statements. Be sure to follow any restrictions specified in the program comments. Once the program works, save it as Lab2Part3.java . (8 pts)
3.d) In your answers file, show the EXACT output that is produced by the program after it is fixed. (4 pts)
Part 4: Given the information below, write a Java program to implement this algorithm.
Define a variable called boxHeightInFeet and give it the value 1.5 .
Define a variable called boxWidthInFeet and give it the value 0.5 .
Define a variable called boxDepthInFeet and give it the value 0.75 .
Define a variable called boxWeightInKilograms and give it the value 4.5.
Using a built-in Math function, increase the box height to the next higher whole number
value. [Hint: look at round, floor, and ceiling functions.]
// Remove the requirement below
//Using a built-in Math function change the box depth to the closest whole number.
Calculate the volume of the box in inches using the modified height, depth, and width.
Calculate the weight in Kg per cubic inch of the box. Also calculate other values as needed to produce the output below.
Your program should produce the following output (dont worry about the indentation level of the output) :
Box height in feet is 2.0 and Box height in inches is 24.0
Box width in feet is 0.5 and Box width in inches is 6.0
Box depth in feet is 0.75 and Box depth in inches is 9.0
Box volume in inches is 1296.00
Box weight in Kg is 4.50
Box weight in Kg per inch is 0.003472
Box weight in Lb is 9.90
4) Save your working program as Lab2Part4.java . (20 pts)
Part 5: Given the program you created for Part 4, modify that program in the following ways.
a. Accept user input values for height, depth, width and weight then do the same modifications and calculations.
b. Produce output that has the same titles and is spaced EXACTLY like the output below: [Your font does NOT have to be the same but it helps if your font is a monospaced or fixed-width font.] The first title is in a 10 char space length then has spaces separating it from the next 10 char title.
c. Have the user input values such that the output below is produced exactly. This means that the values that are put in the program must produce the table below:
Unit Height Width Depth Volume
Inches 24.0 6.0 9.0 1296.0
Feet 2.00 0.50 0.75 0.75
Weight in Pounds Kilograms Kg per In
9.9000 4.5000 0.0035
5.a) Save your working program as Lab2Part5.java . (13 pts)
5.b) Give two different sets of height, depth, and width input values that will produce the output shown above for volume where the height, depth, and width are not the same values as shown above. This means that the height cannot be 2.00, width cannot be 0.50, and the depth cannot be 0.75. Put your two sets of input values in your answers file. Write one set of three values for H, W, and D on one line then write the second set of three values (using a different height, width, and depth) on the next line in the answers file. (12 pts)
--------------------------------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
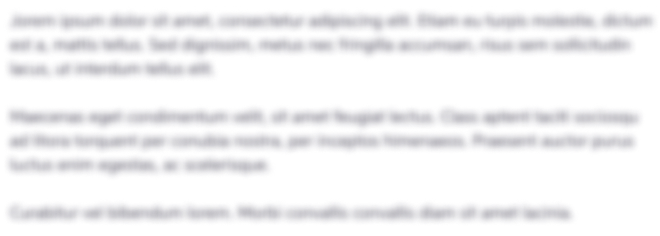
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started