Question
StockHolding Class Write a class called StockHolding. This class will contain information about a single stock holding. It includes a String holding the Symbol for
StockHolding Class
Write a class called StockHolding. This class will contain information about a single stock holding. It includes a String holding the Symbol for the stock (i.e. RCI, APPL, etc). It also holds an integer value that is the number of shares held.
Implement a constructor that takes the company symbol and the initial number of shares purchased.
Implement the following methods:
void addShares(int numberOfShares) This will add to the number of shares in the object (This would be called after purchasing more shares of the stock)
boolean deductShares(int numberOfShares) This will deduct from the total shares held. Make sure this verifies that the number of shares will not go negative. If they will, do not perform the deduction and print an error. Return true if the operation worked and false otherwise.
double getCurrentValue() This will compute the current value of the stock. It will call a method from the Market class that will look up the symbol and provide the current price per share.
Portfolio Class
Write a class called Portfolio. This class tracks your stock market holdings. The class can hold up to 10 objects of type StockHolding. This class should also hold the first and last name of the owner of the portfolio. Additionally, it should have a double value which is the balance of cash usable for stock purchases. Whenever stock is sold, the proceeds of the sale get added to the cash account. Whenever stock are purchased, cash is deducted from the account to cover the purchase. Purchases cannot exceed the cash balance.
Provide a constructor for a portfolio as follows:
Portfolio (String ownerFirstName, String ownerLastName, double startingCashBalance);
This class will hold portfolio information including the first and last name of the owner, a current cash balance (available to purchase stock) and an array of StockHolding objects describing stock held in the portfolio. This class makes use of the class Market (provided by instructor). To get an instance of Market, you can use the following code:
Market theMarket = Market.getMarket();
This will get a copy of the market which is filled with information on different companies and their current stock prices.
Provide the following methods in your Portfolio class:
boolean purchase(String symbol, int numberOfShares) This will purchase the number of shares of the named stock. It should see if the current portfolio contains the stock symbol already. If so, the purchased shares get added to the total in that StockHolding. If not, it should allocate a new StockHolding from the array. If no slot is available, the function returns an error saying the portfolio is full. The method must make sure there is enough cash by looking up the current price and calculating the cost based on the number of shares requested. A Market class will be provided and initialized. You will call market.getQuote(symbol) to get the current value of a share of stock.
The method returns true if the purchase was accomplished, false otherwise.
boolean sell(String symbol, int numberOfShares) This will sell the number of shares of the named stock. It will return false on any error, including:
The symbol is not in the portfolio
The number of shares to sell is greater than the number of shares currently held
The current price is not available from the market
If the sale can be completed, it deducts the number of shares sold from the StockHolding object, computes the sale price based on the price per share (from the market) and number of shares, and adds the sale price into the cash account. Finally, it returns true.
void printPortfolio() This method will print the owners name and then, for each stock holding, it will print the symbol, the number of shares held, and the current market value based on the price per share from the market. At the end of the list, it should print a total portfolio value.
I don't know how to do
The below resourses are for the questions.
public class Market { private static Market theMarket = null; int symbolCount = 0; private StockSymbol[] stocks; static String[] companies = {"IBM", "APPL", "RCI", "INTL", "MOT", "HP", "LCKH", "BOE", "DELL", "ORCL"}; static double[] initCosts = {105.5, 115.4, 98.70, 85.50, 25.30, 10, 55.70, 76.50, 101.10, 35.50}; private Market() { stocks = new StockSymbol[20]; for (int i = 0; i < companies.length; i++) { stocks[i] = new StockSymbol(companies[i], initCosts[i]); } symbolCount = companies.length; } public static Market getMarket() { if (theMarket == null) { theMarket = new Market(); } return theMarket; } public double getQuote(String symbol) { double returnValue = -1.0; for (int i = 0; i < symbolCount; i++) { if (stocks[i].symbol.equals(symbol)) { returnValue = stocks[i].currentPrice; break; } } return returnValue; } }
public class StockSymbol { String symbol; double currentPrice; StockSymbol(String symbol, double price) { this.symbol = symbol; this.currentPrice = price; } }
import Resources.Market;
public class StockHolding { // Add private members here StockHolding() { } StockHolding(String symbol, int numberOfShares) { } public void addShares(int numberToAdd) { } public boolean deductShare(int numberToDeduct) { } public double getCurrentValue() { } }
import Resources.Market;
public class Portfolio { // Add members here private Market theMarket; Portfolio(String firstName, String lastName, double cash) { // This gets the Market object needed for quotes. theMarket = Marget.getMarket(); } boolean purchase(String symbol, int numberOfShares) {
} boolean sell(String symbol, int numberOfShares) { } void printPortfolio() { } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
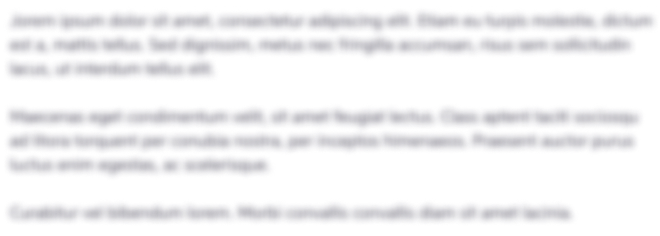
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started